How to Add Key-Value Pairs to a Dictionary Within a Loop in Python
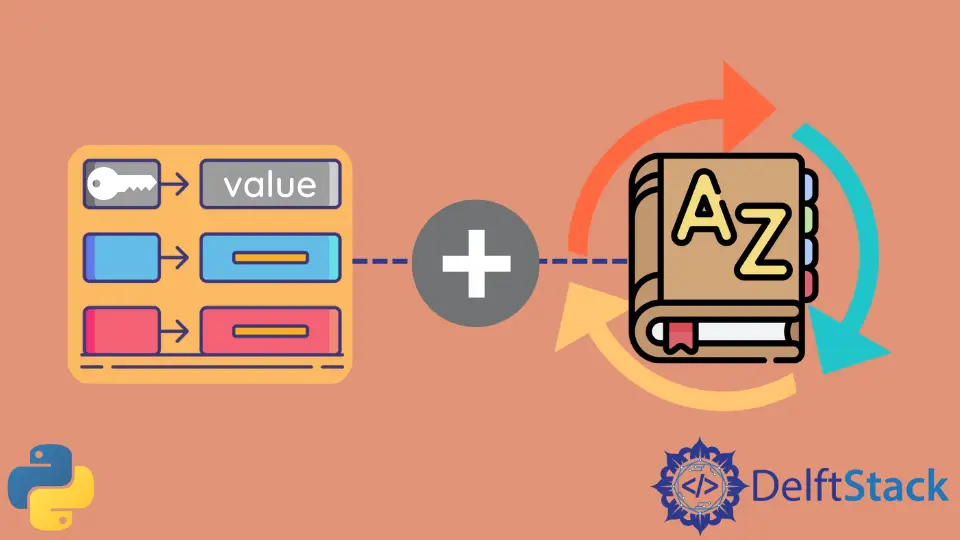
Dictionary is an amazing and efficient data structure to store data in the form of key-value pairs in Python.
Since it is a data structure, a dictionary is not just specific to Python but also available in other programming languages such as C++, Java, JavaScript, etc. It is referred to with a different name such as map and JSON (JavaScript Object Notation) object.
A dictionary has a key, and a key can be any value or object that is hashable and immutable. The reason behind these two requirements is that an object’s hash representation depends on the values it is storing inside it.
If the values can be manipulated over time, the object would not have a unique and fixed hash representation. A value within a dictionary can be anything; it can be an integer value, a float value, a double value, a string value, a class object, a list, a binary tree, a linked list, a function, and even a dictionary.
When it comes to time complexity, a dictionary takes constant time, O(1)
, on average, to add, delete, and access an element.
This article will talk about how to add key-value pairs to a dictionary within a loop.
Add Key-Value Pairs to a Dictionary Within a Loop
To add key-value pairs to a dictionary within a loop, we can create two lists that will store the keys and values of our dictionary. Next, assuming that the ith
key is meant for the ith
value, we can iterate over the two lists together and add values to their respective keys inside the dictionary.
Let us understand this with the help of some Python code, refer to the following code:
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print(dictionary)
Output:
{'Integer': 1, 'String': 'Hello', 'Float': 2.567, 'List of Strings': ['Computer', 'Science'], 'List of Float Numbers': [1.235, 5.253, 77.425], 'List of Integers': [11, 22, 33, 44, 55], 'Dictionary': {'a': 500, 'b': 1000, 'c': 1500}, 'Class Object': <__main__.Point object at 0x7f2c74906d90>, 'Function': <function add at 0x7f2c748a3d30>, 'List of Class Objects': [<__main__.Point object at 0x7f2c749608b0>, <__main__.Point object at 0x7f2c748a50a0>, <__main__.Point object at 0x7f2c748a5430>, <__main__.Point object at 0x7f2c748a53d0>]}
The time complexity of the above solution is O(n)
, and the space complexity of the above solution is also O(n)
, where n
is the size of the keys
and values
lists. Moreover, the code above depicts that all types of values that we talked about can be stored inside a dictionary.
We can beautify the output by re-iterating the dictionary and printing each key-value pair or printing each key-value pair while adding them to the dictionary. Note that one can also use pre-built Python packages such as the json
package and external open-source packages to play around with the JSON output, add color coding, add indentation, etc.
For our use case, we will create a stub function for printing a dictionary. Refer to the following code:
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print_dictionary(dictionary)
Output:
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7f7d94160d90>
Function: <function add at 0x7f7d940fddc0>
List of Class Objects: [<__main__.Point object at 0x7f7d941ba8b0>, <__main__.Point object at 0x7f7d940ff130>, <__main__.Point object at 0x7f7d940ff310>, <__main__.Point object at 0x7f7d940ff3d0>]
The time and space complexity of the above solution is the same as the previous solution’s, O(n)
.
The above two code snippets use a for
loop. We can perform the same task using a while
loop.
The following code snippet depicts adding values to a dictionary using a while
loop.
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
n = min(len(keys), len(values))
i = 0
while i != n:
dictionary[keys[i]] = values[i]
i += 1
print_dictionary(dictionary)
Output:
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7fdbe16c0d90>
Function: <function add at 0x7fdbe165ddc0>
List of Class Objects: [<__main__.Point object at 0x7fdbe171a8b0>, <__main__.Point object at 0x7fdbe165f130>, <__main__.Point object at 0x7fdbe165f310>, <__main__.Point object at 0x7fdbe165f3d0>]
The time and space complexity of the above solution is the same as the previous solution’s, O(n)
.