Agregar pares de valores-clave a un diccionario dentro de un bucle en Python
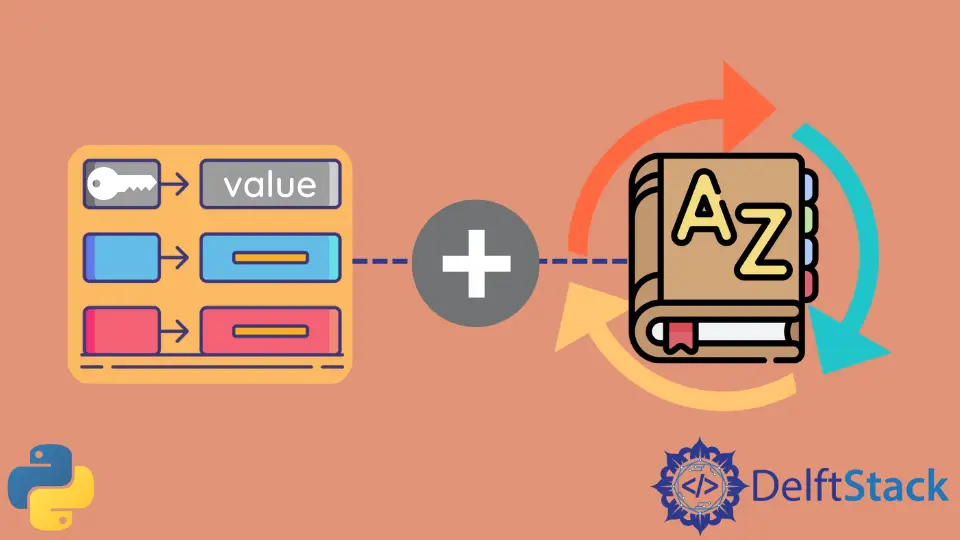
Dictionary es una estructura de datos asombrosa y eficiente para almacenar datos en forma de pares clave-valor en Python.
Dado que es una estructura de datos, un diccionario no solo es específico de Python, sino que también está disponible en otros lenguajes de programación como C++, Java, JavaScript, etc. Se lo conoce con un nombre diferente, como mapa y objeto JSON (JavaScript Object Notation).
Un diccionario tiene una clave, y una clave puede ser cualquier valor u objeto que sea hash e inmutable. La razón detrás de estos dos requisitos es que la representación hash de un objeto depende de los valores que almacena en su interior.
Si los valores se pueden manipular a lo largo del tiempo, el objeto no tendría una representación hash única y fija. Un valor dentro de un diccionario puede ser cualquier cosa; puede ser un valor entero, un valor flotante, un valor doble, un valor de cadena, un objeto de clase, una lista, un árbol binario, una lista vinculada, una función e incluso un diccionario.
En lo que respecta a la complejidad del tiempo, un diccionario requiere un tiempo constante, O(1)
, en promedio, para agregar, eliminar y acceder a un elemento.
Este artículo hablará sobre cómo agregar pares clave-valor a un diccionario dentro de un bucle.
Agregar pares clave-valor a un diccionario dentro de un bucle
Para agregar pares clave-valor a un diccionario dentro de un bucle, podemos crear dos listas que almacenarán las claves y valores de nuestro diccionario. A continuación, asumiendo que la clave ith
está destinada al valor ith
, podemos iterar sobre las dos listas juntas y agregar valores a sus respectivas claves dentro del diccionario.
Entendamos esto con la ayuda de algún código de Python, consulte el siguiente código:
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print(dictionary)
Producción :
{'Integer': 1, 'String': 'Hello', 'Float': 2.567, 'List of Strings': ['Computer', 'Science'], 'List of Float Numbers': [1.235, 5.253, 77.425], 'List of Integers': [11, 22, 33, 44, 55], 'Dictionary': {'a': 500, 'b': 1000, 'c': 1500}, 'Class Object': <__main__.Point object at 0x7f2c74906d90>, 'Function': <function add at 0x7f2c748a3d30>, 'List of Class Objects': [<__main__.Point object at 0x7f2c749608b0>, <__main__.Point object at 0x7f2c748a50a0>, <__main__.Point object at 0x7f2c748a5430>, <__main__.Point object at 0x7f2c748a53d0>]}
La complejidad temporal de la solución anterior es O(n)
, y la complejidad espacial de la solución anterior también es O(n)
, donde n
es el tamaño de las listas de claves
y valores
. Además, el código anterior describe que todos los tipos de valores de los que hablamos se pueden almacenar dentro de un diccionario.
Podemos embellecer la salida repitiendo el diccionario e imprimiendo cada par clave-valor o imprimiendo cada par clave-valor mientras los agregamos al diccionario. Tenga en cuenta que también se pueden usar paquetes de Python prediseñados como el paquete json
y paquetes externos de código abierto para jugar con la salida JSON, agregar codificación de colores, agregar sangría, etc.
Para nuestro caso de uso, crearemos una función stub para imprimir un diccionario. Consulte el siguiente código:
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print_dictionary(dictionary)
Producción :
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7f7d94160d90>
Function: <function add at 0x7f7d940fddc0>
List of Class Objects: [<__main__.Point object at 0x7f7d941ba8b0>, <__main__.Point object at 0x7f7d940ff130>, <__main__.Point object at 0x7f7d940ff310>, <__main__.Point object at 0x7f7d940ff3d0>]
La complejidad temporal y espacial de la solución anterior es la misma que la de la solución anterior, O(n)
.
Los dos fragmentos de código anteriores utilizan un bucle for
. Podemos realizar la misma tarea usando un bucle while
.
El siguiente fragmento de código muestra la adición de valores a un diccionario mediante un bucle while
.
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
n = min(len(keys), len(values))
i = 0
while i != n:
dictionary[keys[i]] = values[i]
i += 1
print_dictionary(dictionary)
Producción :
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7fdbe16c0d90>
Function: <function add at 0x7fdbe165ddc0>
List of Class Objects: [<__main__.Point object at 0x7fdbe171a8b0>, <__main__.Point object at 0x7fdbe165f130>, <__main__.Point object at 0x7fdbe165f310>, <__main__.Point object at 0x7fdbe165f3d0>]
La complejidad temporal y espacial de la solución anterior es la misma que la de la solución anterior, O(n)
.
Artículo relacionado - Python Dictionary
- Cómo comprobar si existe una clave en un diccionario en Python
- Convertir un diccionario en una lista en Python
- Cómo obtener todos los archivos de un directorio
- Cómo encontrar el valor máximo en el Diccionario Python
- Cómo ordenar un diccionario Python por valor
- Cómo fusionar dos diccionarios en Python 2 y 3