How to Check if a Python String Is a Palindrome
- Check if a Python String Is a Palindrome Using List Slicing
-
Check if a Python String Is a Palindrome Using the
reversed()
Function - Check if a Python String Is a Palindrome Using a Loop
- Check if a Python String Is a Palindrome Using Recursion
- Check if a Python String Is a Palindrome Using Deque (Double-Ended Queue)
- Check if a Python String Is a Palindrome While Ignoring Case and Spaces
- Conclusion
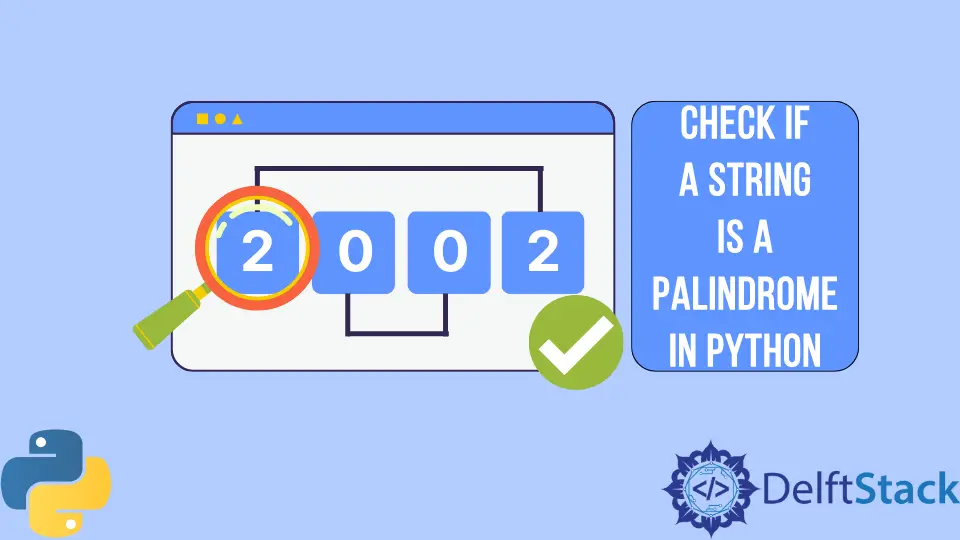
A palindrome is a sequence of characters that reads the same forwards as it does backward. Palindrome strings remain unchanged when their characters are reversed.
Similarly, if a reversed number is the same as the original one, then the number is a palindrome number.
This characteristic makes palindromes a fascinating subject for exploration, not only in language but also in various fields such as mathematics, literature, data science, and computer science.
In Python programming, checking whether a string is a palindrome is a common programming task and can be accomplished easily. In this article, we will walk through the methods on how to check if a string is a palindrome.
Check if a Python String Is a Palindrome Using List Slicing
One effective method to determine if a string is a palindrome is through list slicing. List slicing is a concise and powerful feature in Python that allows us to extract a portion of a sequence, such as a string.
To check if a string is a palindrome, we can leverage list slicing to create a reversed string version of the original string. The key insight is that a palindrome remains unchanged when read in reverse.
By comparing the original string with the reversed string counterpart, we can ascertain whether the given string is a palindrome.
Check Palindrome in Python Using List Slicing Example
# Enter string
word = input()
# Check for palindrome strings using list slicing
if str(word) == str(word)[::-1]:
print("Palindrome")
else:
print("Not Palindrome")
The program begins by prompting the user to input a string using the input()
function. This allows us to dynamically test any string for palindromic properties.
The core logic lies in the following lines:
if str(word) == str(word)[::-1]:
print("Palindrome")
Here, str(word)[::-1]
utilizes list slicing to create a reversed version of the input string. The [::-1]
notation indicates a step of -1
, effectively reversing the string.
The code then compares the original string (str(word))
with its reversed counterpart (str(word)[::-1])
. If they are equal, the string is deemed a palindrome, and Palindrome
is printed. Otherwise, Not Palindrome
is printed.
Code Output
Let’s consider the input string hello
.
In this example, the Palindrome program correctly identifies level
as a palindrome and hello
as not a palindrome, showcasing the effectiveness of the list slicing method for palindrome checks in Python.
Check if a Python String Is a Palindrome Using the reversed()
Function
In addition to list slicing, another approach to determining palindrome strings in Python involves using the reversed()
function in combination with the join()
method.
This method provides an alternative perspective on palindrome checks and can be particularly useful in certain scenarios.
Syntax of the reversed()
Function
The reversed()
function is used to reverse the elements of a sequence. In the context of checking palindromes, we can apply this function to reverse the characters within a string.
The syntax is as follows:
reversed_seq = reversed(sequence)
Here, sequence
represents the original sequence of elements, and reversed_seq
is the reversed version obtained using the reversed()
function.
The reversed()
function returns a reverse iterator, which can be converted back to a sequence using "".join()
. By applying this method to a string, we obtain the reversed string version.
We can then compare the original and reversed strings to determine whether the given string is a palindrome. Let’s see a Python program to check if a string is a palindrome or not.
Check Palindrome in Python Using reversed()
Example
# Enter string
word = input()
if str(word) == "".join(reversed(word)): # cycle through the string in reverse order
print("Palindrome")
else:
print("Not Palindrome")
Similar to the previous example, this Python program begins by obtaining a string input from the user using the input()
function.
The pivotal lines of code are:
if str(word) == "".join(reversed(word)):
print("Palindrome")
Here, the predefined function "".join(reversed(word))
is used, where reversed(word)
returns a reversed iterator for the input string, and "".join()
converts it back to a string.
The original and reversed strings are then compared for palindromic equality.
The code proceeds to check if the original string (str(word))
is equal to the reversed string ("".join(reversed(word)))
. If the condition is met, the string is identified as a palindrome, and Palindrome
is printed; otherwise, Not Palindrome
is printed.
Code Output
Let’s consider the input string world
.
This Palindrome program in Python correctly recognizes deified
as a palindrome and world
as not a palindrome, showcasing the efficacy of the reversed()
function in conjunction with join()
for palindrome checks in Python.
Check if a Python String Is a Palindrome Using a Loop
Another method for checking if a string is a palindrome in Python involves using an iterative loop. This approach provides a more explicit way to iterate through the string and compare characters.
The idea behind using a loop for palindrome checking is to iterate through the characters of the string, comparing the first and last, then moving inward. If, at any point, the characters don’t match, the string is not a palindrome.
This method manually simulates the process of checking symmetry that is inherent in palindromes.
Check Palindrome String in Python Using a Loop Example
# Enter string
word = input()
# Check if string is palindrome using a loop
is_palindrome = True
length = len(word)
for i in range(length // 2):
if word[i] != word[length - i - 1]:
is_palindrome = False
break
if is_palindrome:
print("Palindrome")
else:
print("Not Palindrome")
As in the previous examples, the program begins by obtaining a string input from the user using the input()
function.
The crucial loop section is:
for i in range(length // 2):
if word[i] != word[length - i - 1]:
is_palindrome = False
break
This loop iterates through the first half of the string, comparing characters symmetrically from both ends of the sliced string. If a mismatch is found, is_palindrome
is set to False
, and the loop breaks.
Following the loop execution, the program checks the value of is_palindrome
. If it remains True
, the string is identified as a palindrome, and Palindrome
is printed; otherwise, Not Palindrome
is printed.
Code Output
Let’s consider the input string python
.
This Python program correctly recognizes radar
as a palindrome and python
as not a palindrome using the loop method, emphasizing the versatility of different approaches for palindrome checks in Python.
Check if a Python String Is a Palindrome Using Recursion
Another interesting approach to determine if a string is a palindrome involves using the recursion method.
Recursion is a concept where a function calls itself, and in the context of checking palindromes, we approach the problem by comparing the first and last characters of the string.
If they match, we move on to the second and second-to-last characters, and so on. This process continues until we either find a pair of characters that don’t match or the string is reduced to a length of 1 or 0, signifying a palindrome.
Check Palindrome String in Python Using Recursion Example
# Define a function for palindrome check using recursion
def is_palindrome(s):
if len(s) <= 1:
return True
return s[0] == s[-1] and is_palindrome(s[1:-1])
# Enter string
word = input()
# Check if the string is a palindrome using recursion
if is_palindrome(word):
print("Palindrome")
else:
print("Not Palindrome")
The program starts by defining a function, is_palindrome(s)
, which takes a string argument s
as input. In the function body, we check if the string is a palindrome by comparing the first and last characters and making a recursive call on the remaining substring.
The core logic is encapsulated in the recursive function:
def is_palindrome(s):
if len(s) <= 1:
return True
return s[0] == s[-1] and is_palindrome(s[1:-1])
The function first checks if the length of the string is 1 or less, in which case it is inherently a palindrome. Otherwise, the method compares the first and last characters (s[0] == s[-1]
) and makes a recursive call on the substring between them (is_palindrome(s[1:-1])
).
Following the function definition, the program takes user input and calls the is_palindrome
function. If the function returns True
, the string is identified as a palindrome, and Palindrome
is printed; otherwise, Not Palindrome
is printed.
Code Output
Let’s consider the input string python
.
As we can see, the Palindrome program correctly recognizes level
as a palindrome using the recursive method, highlighting the simplicity and effectiveness of recursion for palindrome checks in Python.
Check if a Python String Is a Palindrome Using Deque (Double-Ended Queue)
Yet another approach to determine if a string is a palindrome involves utilizing the deque
(double-ended queue) data structure. A deque
allows efficient adding and removing of elements from both ends, making it well-suited for palindrome checks.
A deque
provides O(1)
time complexity for appending and popping elements from both ends. In the context of checking palindromes, we can use a deque
to compare characters symmetrically from both ends of the string.
This method is efficient and avoids unnecessary string slicing or reversing. Here’s a Python program to check if a string is a palindrome or not.
Check Palindrome String in Python Using Deque and while
Loop Example
from collections import deque
# Input: Take a string from the user
word = input()
# Function to check string using a deque and `while` loop
def is_palindrome(s):
chars = deque(s)
while len(chars) > 1:
if chars.popleft() != chars.pop():
return False
return True
# Check and print the result
if is_palindrome(word):
print("Palindrome")
else:
print("Not Palindrome")
We start by obtaining a string input from the user using the input()
function.
The is_palindrome
function initializes a deque
with the characters of the input string. Using the while
loop, it then iteratively compares the first character with the last character using popleft()
and pop()
.
If a mismatch is found, it returns False
; otherwise, it returns True
.
The program then checks the result of the is_palindrome
function. If it returns True
, the string is identified as a palindrome, and Palindrome
is printed; otherwise, Not Palindrome
is printed.
Code Output
Let’s consider the input string python
.
In this example, the program correctly recognizes radar
as a palindrome and python
as not a palindrome using the deque
method, highlighting the efficiency and simplicity of utilizing a double-ended queue for palindrome checks in Python.
Check if a Python String Is a Palindrome While Ignoring Case and Spaces
When checking if a string is a palindrome in Python, it’s often desirable to ignore differences in case and spaces. This modification allows for a more flexible palindrome check that considers alphanumeric characters only.
To create a case-insensitive and space-agnostic palindrome check, we preprocess the input string by converting it to lowercase and filtering out non-alphanumeric characters.
This ensures that the comparison is solely based on the sequence of letters and digits, disregarding case and spaces.
Check Palindrome String in Python While Ignoring Case and Spaces Example
# Enter input number or string
word = input()
# Check if the string is a palindrome, ignoring case and spaces
def is_palindrome(s):
s = "".join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
# Check and print the result
if is_palindrome(word):
print("Palindrome")
else:
print("Not Palindrome")
The program begins by obtaining a string input from the user using the input()
function.
The is_palindrome
function takes a string argument and converts it to lowercase (c.lower()
) and filters out non-alphanumeric characters (if c.isalnum()
). This preprocessed string is then compared with the reversed string version using the list slicing method (s == s[::-1]
).
The program then checks the result of the is_palindrome
function. If it returns True
, the string is identified as a palindrome, and Palindrome
is printed; otherwise, Not Palindrome
is printed.
For illustration purposes, let’s consider the palindrome phrase A man, a plan, a canal, Panama!
.
In this example, the Palindrome program correctly recognizes A man, a plan, a canal, Panama!
as a palindrome while ignoring case and spaces, showcasing the adaptability of this method for various input formats in Python palindrome checks.
Conclusion
In this article, we’ve uncovered a range of approaches to check if a string is a palindrome in Python, each with its strengths and characteristics. From list slicing to using a deque
and recursion to the while
loop, Python provides multiple tools for tackling this common programming challenge.
List slicing provides a concise and readable solution, while the reversed()
function and join()
method offer an alternative approach. The recursive method allows for an elegant expression of the palindrome-checking logic.
Meanwhile, leveraging a deque
showcases the efficiency gained by utilizing a specialized data structure. Lastly, the simplicity of a while
loop provides a clear and understandable solution. Ultimately, the choice of method depends on the specific needs of the task at hand.
To improve your coding skills and deepen your understanding of string manipulation and pattern matching, consider delving into Python regular expressions (regex). It’s widely used in text-processing tasks and can be a valuable addition to your Python toolkit.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn