How to Loop Backward Iteration in Python
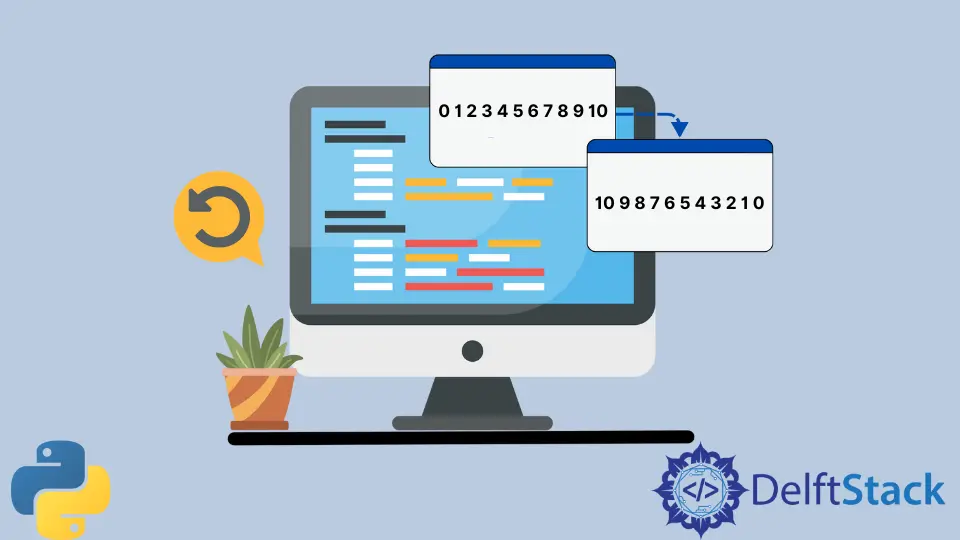
In Python, number iteration is accomplished through the use of looping techniques. In this article we perform looping using reserved()
function and range()
function in Python.
Loop Backwards in Python
There are numerous techniques that make looping easier. Sometimes we need to loop backward, and having shorthands can be very useful.
Use the reserved()
Function to Loop Backwards
One of the easiest ways to implement looping backward is to use the reversed()
function for the for
loop, and the iterative process will begin from the backside rather than the front side as in conventional counting. First, it initializes the number 10
and stores it in the Number
variable.
Following that, the for
loop with a reversed()
function to perform backward iteration and range, containing parameters with a variable count, increased by 1
to print the number.
Code:
print("The reversed numbers are : ", end="")
for num in reversed(range(Number + 1)):
print(num, end=" ")
Output:
The reversed numbers are : 10 9 8 7 6 5 4 3 2 1 0
The output of the above code starts at 10
and ends at 0
.
Use the range()
Function to Loop Backwards
This task can also be accomplished with the traditional range()
function, which performs the skip when given the third argument, and the second argument is used to start from the beginning.
Code:
N = 10
print("The reversed numbers are : ", end="")
for num in range(N, -1, -1):
print(num, end=" ")
Output:
The reversed numbers are : 10 9 8 7 6 5 4 3 2 1 0
Conclusion
In this short article, we discussed the iteration of the loop backward by using two methods. We use the reversed()
function in the first method.
The second method is the traditional range()
function, which performs the skip in the third given argument, and the second argument is used to start from the beginning.