How to Get Longest Substring Without Repeating Characters in Python
- Longest Substring Without Repeating Characters in Python
-
Use a
While
Loop to Get the Longest Substring in Python -
Use a
For
Loop and anIf-Else
Statement to Get the Longest Substring in Python
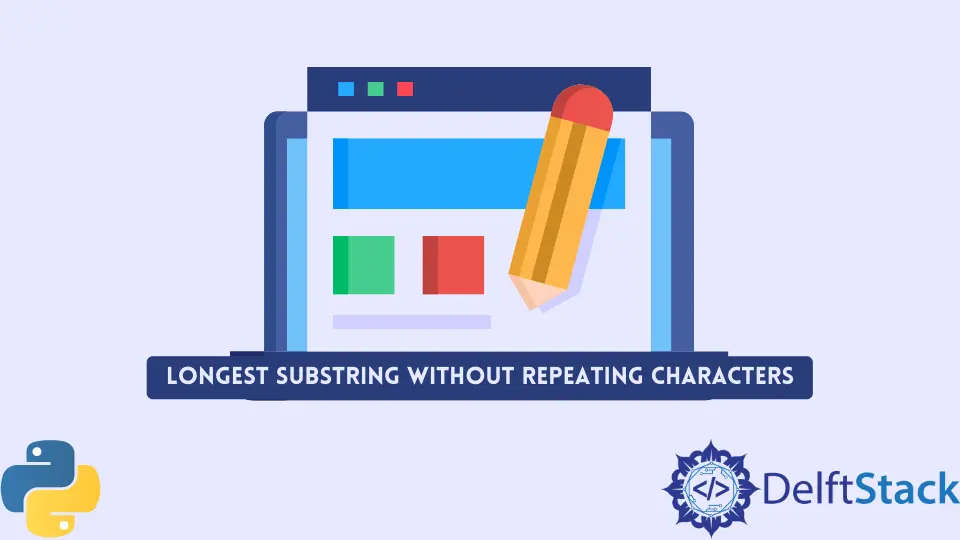
We will introduce how to make substrings in Python and how we can make a substring without repeating characters with examples.
Longest Substring Without Repeating Characters in Python
In this tutorial, we will learn something unique that can boost your knowledge and make your loops even more perfect. We will learn how to make substrings from a string without repeating characters.
The substring means that if we have a string and break it into multiple small parts, they will be called substrings of that specific string. The longest substring method is very helpful if we want to get the longest substring that does not repeat the same alphabets.
In this method, we will use a loop that goes through the complete string, checks each element one by one, and gives the non-repeated substring of the original string.
In Python programming, there are many methods of getting the longest substring. These methods are very simple and helpful; we will discuss them in detail.
Use a While
Loop to Get the Longest Substring in Python
We will create a class GetLongestSubstring
that will take object
as a parameter in this method. We will define a function called Length
that will take two parameters inside this class.
We will go through the while
loop until we have found the longest substring from the given string.
# python
class GetLongestSubstring(object):
def Length(self, x):
a = 0
b = 0
c = {}
sol = 0
while b < len(x):
if x[b] not in c or a > c[x[b]]:
sol = max(sol, (b - a + 1))
c[x[b]] = b
else:
a = c[x[b]] + 1
sol = max(sol, (b - a + 1))
b -= 1
b += 1
return sol
obj = GetLongestSubstring()
print(obj.Length("ABCDEFGABCGHIJKLMNO"))
Output:
As you can see from the above example, the longest substring possible has a length of 12, the same as the ABCGHIJKLMNO
substring from the original string.
Use a For
Loop and an If-Else
Statement to Get the Longest Substring in Python
We will try to achieve the same scenario using the for
loop. First, we will define the function GetLongestSubstring
as shown below.
This function will convert the original string into multiple substrings and check if the substring is unique or not. As shown below, this function will return the longest substring with its length.
# python
def GetLongestSubstring(x):
if len(set(x)) == len(x):
return len(x)
Substring = ""
StrLen = 1
for a in x:
if a not in Substring:
Substring = Substring + a
StrLen = max(StrLen, len(Substring))
else:
Substring = Substring.split(a)[1] + a
print(Substring)
return StrLen
print(GetLongestSubstring("ABCDEFGABCGHIJKLMNO"))
Output:
As you can see from the above solution, we can easily get the longest substring from a string using the for
loop with the if-else
statement.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn