How to Print List Without Square Brackets in Python
-
Use the
join()
Function to Print Lists Without Square Brackets in Python - Use the Unpack Method to Print Lists Without Square Brackets in Python
-
Use the
str
Function to Print Lists Without Square Brackets -
Use the
for
Loop andend
Parameter to Print List without Brakcets in Python - Printing List Elements without Square Brackets
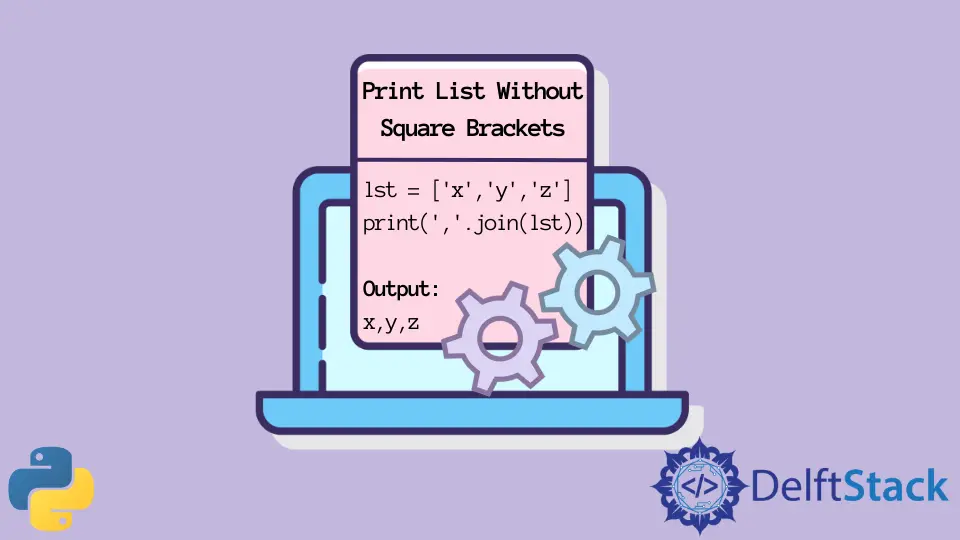
This tutorial will introduce how to print a list without showing these square brackets.
Use the join()
Function to Print Lists Without Square Brackets in Python
The join()
function takes all the elements from an iterable object, like a list, and returns a string with all elements separated by a character specified with the function. Using this method, we can remove the square brackets from a list and separate the elements using a comma or whichever character we desire.
The join()
function is a string method that takes an iterable object (e.g., a list) as its argument and returns a new string by joining all the elements of the iterable together. You can specify a character or a string that acts as a delimiter to separate the elements in the resulting string.
Here’s the basic syntax of the join()
function:
delimiter.join(iterable)
delimiter
: The character or string used to separate the elements in the resulting string.iterable
: The iterable object (e.g., a list) containing the elements to be joined.
Let’s start with a simple example where we have a list of strings, and we want to join them into a single string with a comma as the delimiter.
lst = ["x", "y", "z"]
print(",".join(lst))
Output:
x,y,z
Note that this method only works with a list containing strings and fails with a list of integer or float values.
Handling Non-String Elements
It’s important to note that the join()
method works with string elements in the iterable. If you attempt to join a list of integers or floats, you’ll encounter a TypeError. To work with non-string elements, you need to convert them to strings first. Here’s an example illustrating this:
pythonCopy codenumbers = [1, 2, 3]
# This will raise a TypeError
# result = ",".join(numbers)
# Convert numbers to strings and then join
result = ",".join(map(str, numbers))
print(result)
Output:
1,2,3
In this modified example:
- We initially have a list
numbers
containing integer elements. - Attempting to use
join()
directly on the list of integers raises a TypeError. - To resolve this, we use the
map()
function to convert each integer to a string, and then we usejoin()
on the converted strings.
Use the Unpack Method to Print Lists Without Square Brackets in Python
The *
operator in Python is a versatile tool used for unpacking elements from iterable objects like lists, tuples, or dictionaries. When applied to a list, it extracts all the elements from the list and allows you to use them as separate arguments in a function or as standalone elements in an expression.
Here’s the basic syntax of the *
operator:
*iterable
In the context of list printing, we can use the *
operator to print the list elements without square brackets. Let’s look at an example:
pythonCopy codelst = ["x", "y", "z"]
print(*lst, sep=",")
Output:
x,y,z
In this example:
- We have a list
lst
containing the elementsx
,y
, andz
. - We use the
*lst
syntax within theprint()
function, which unpacks the list elements as separate arguments. - The
sep=","
parameter is used to specify that we want to separate the elements with a comma.
Understanding the sep
Parameter
The sep
parameter in the print()
function allows you to define the separator character or string used between multiple items in the printed output. By default, it is set to a space character " "
. However, you can specify a custom separator to format the output as desired.
In the previous example, we used sep=","
to separate the elements with commas. If we remove the sep
parameter, the default behavior (using a space separator) is applied:
lst = ["x", "y", "z"]
print(*lst)
Output:
x y z
Handling Different Types of Elements
The unpacking method is not limited to lists of strings; it can handle lists containing elements of different types, including numbers, strings, or any other valid Python objects. Here’s an example illustrating this versatility:
mixed_lst = [1, "two", 3.0, True]
print(*mixed_lst, sep=" | ")
Output:
1 | two | 3.0 | True
In this example, we have a list mixed_lst
containing a mix of integers, strings, floats, and a boolean value. The *mixed_lst
expression effectively unpacks these diverse elements and prints them with a custom separator.
Use the str
Function to Print Lists Without Square Brackets
The str()
function in Python is used to convert objects to string representations. When applied to a list, it converts the entire list into a string. To remove the square brackets, you can slice the string to exclude the first and last characters.
lst = [1, 2, 3]
lst_str = str(lst)[1:-1]
print(lst_str)
Output:
1, 2, 3
In this example:
- We have a list
lst
containing the elements 1, 2, and 3. - We use the
str(lst)
function to convert the entire list into a string representation. - We slice the resulting string using
[1:-1]
, which removes the first and last characters (the square brackets). - Finally, we print the modified string, which contains the list elements separated by commas.
Note that this method can work with a list containing integers or floats values also.
Another way in which we can use this function is to convert each element of a list to a string. We can then proceed to remove the brackets using the join()
function as discussed earlier. For example,
lst = [1, 2, 3]
lst_new = [str(a) for a in lst]
print(",".join(lst_new))
Output:
1,2,3
In this example:
- We have the same list
lst
containing the elements 1, 2, and 3. - We use a list comprehension
[str(a) for a in lst]
to convert each element of the original list to a string. - Then, we use the
join()
function with a comma","
as the separator to join the converted elements into a single string.
This approach allows you to customize the separator and format the output as needed.
Use the for
Loop and end
Parameter to Print List without Brakcets in Python
Python’s for
loop is a versatile construct for iterating over elements in an iterable, such as a list. It allows you to access each element one at a time and perform actions on them. Additionally, Python’s print()
function provides the end
parameter, which allows you to specify a character or string to be printed at the end of each print()
call, instead of the default newline character ("\n"
).
Here’s the basic syntax of using the for
loop and the end
parameter:
for element in iterable:
print(element, end=separator)
element
: Represents the current element being processed in the loop.iterable
: The iterable object, such as a list, over which the loop iterates.separator
: The character or string used to separate the printed elements.
Printing List Elements without Square Brackets
Let’s use this method to print the elements of a list without square brackets. We’ll start with a simple example:
lst = [1, 2, 3]
for item in lst:
print(item, end=" ")
Output:
1 2 3
In this example:
- We have a list
lst
containing the elements 1, 2, and 3. - We use a
for
loop to iterate over each element (item
) in the list. - Within the loop, we print each element followed by a space character (
" "
) as the separator, usingprint(item, end=" ")
.
The result is a clean and space-separated list of elements, with no square brackets.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python