How to Convert List to Matrix in Python
- Use a Loop and List Slicing to Convert a List to an Array or Matrix in Python
-
Use the
array()
Function From the Numpy Library to Convert a List to an Array or Matrix in Python -
Use the
asarray()
Function From the Numpy Library to Convert a List to an Array or Matrix in Python
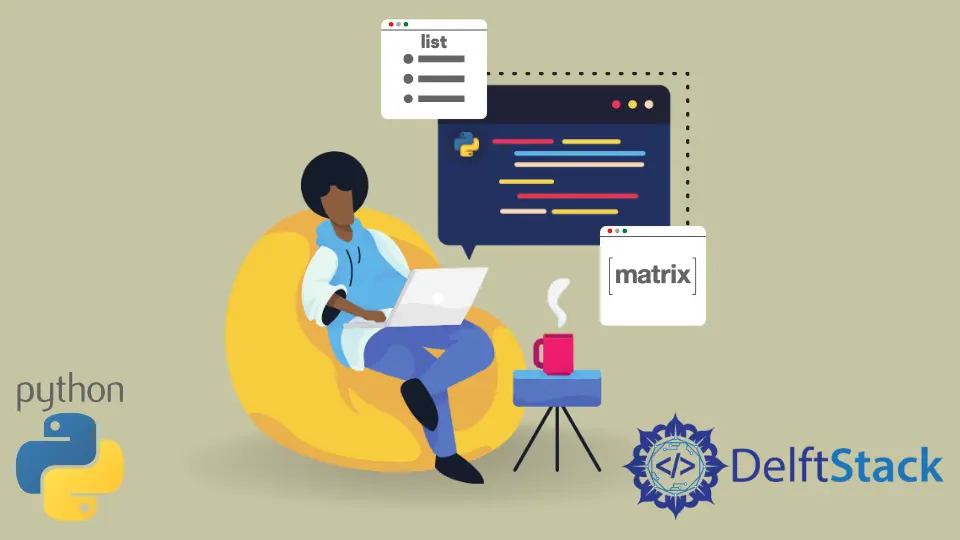
This tutorial will demonstrate the different methods available to convert a list to a matrix in Python.
Both lists and matrices are different data types provided by Python to stock several items under a single variable. Accessing these items becomes relatively easy in both cases.
Unfortunately, we cannot perform some mathematical functions on lists. Therefore, lists are consequently converted to matrices or arrays, in most cases, to execute specific mathematical functions successfully.
Python does not directly contain an array data type but contains matrices that are subclasses of arrays, which gives them all the features of an array apart from their own. There are several ways to convert a list to a matrix in Python, all mentioned below.
Use a Loop and List Slicing to Convert a List to an Array or Matrix in Python
A simple matrix can be made using the concept of nested lists. In this method, the task is to convert the list containing the given items into lists.
This can be implemented by simple utilization of a loop, the append()
function, and the concept of list slicing. Here, we will utilize the while
loop to implement the task at hand.
The following code uses a loop and list slicing to convert a list to a matrix in Python.
x = [2, 10, 20, 200, 4000]
mat = []
while x != []:
mat.append(x[:2])
x = x[2:]
print(mat)
Output:
[[2, 10], [20, 200], [4000]]
In the above code, we choose to implement the list slicing of value 2
. This can vary according to the user’s needs and can change the position of the elements in the matrix.
Use the array()
Function From the Numpy Library to Convert a List to an Array or Matrix in Python
NumPy is an essential library that enables the user to create and manipulate arrays and matrices in Python.
The numpy.array
method can declare an array in Python. A list can directly be taken as an argument to this function, and the result after using this function is a matrix.
The NumPy library needs to be imported to the Python code to run this program without any errors.
The following code uses the array()
function from the NumPy library to convert a list to an array or matrix in Python.
import numpy as np
x = [12, 10, 20, 200, 4000]
mat = np.array(x)
print(mat)
Output:
[ 12 10 20 200 4000]
Moreover, the NumPy library also contains another function, reshape()
, that lets the programmer decide the shape and positioning of the list elements according to their need.
The following code can be looked at as an example to implement the numpy.reshape()
function.
import numpy as np
x = np.array([12, 10, 20, 200])
shape = (2, 2)
print(x.reshape(shape))
Output:
[[ 12 10]
[ 20 200]]
Use the asarray()
Function From the Numpy Library to Convert a List to an Array or Matrix in Python
The numpy.asarray()
function’s working is similar to the numpy.array()
function. This function can enable the conversion process of several data-type objects like dictionaries, lists, and more into simple NumPy matrices.
The numpy.array()
method is called within the numpy.asarray()
function, which is why the syntax of the former function is said to be just an extension of the latter.
In the numpy.asarray()
function, the copy
flag defaults to False, which makes it different from the numpy.array()
function which defaults the copy
flag to True.
The following code uses the asarray()
function from the NumPy library to convert a list to an array or matrix in Python.
import numpy as np
x = [2, 10, 20, 200, 4000]
mat = np.asarray(x)
print(mat)
Output:
[ 2 10 20 200 4000]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python