How to Convert List to Pandas DataFrame in Python
- Convert List to a Pandas DataFrame in Python
- Store the List in a Column in Pandas DataFrame in Python
- Convert a List to a DataFrame With Index in Python
-
Zip Two Lists Into a Single DataFrame Using
zip()
in Python - Convert a Multi-Dimensional List to a Pandas DataFrame in Python
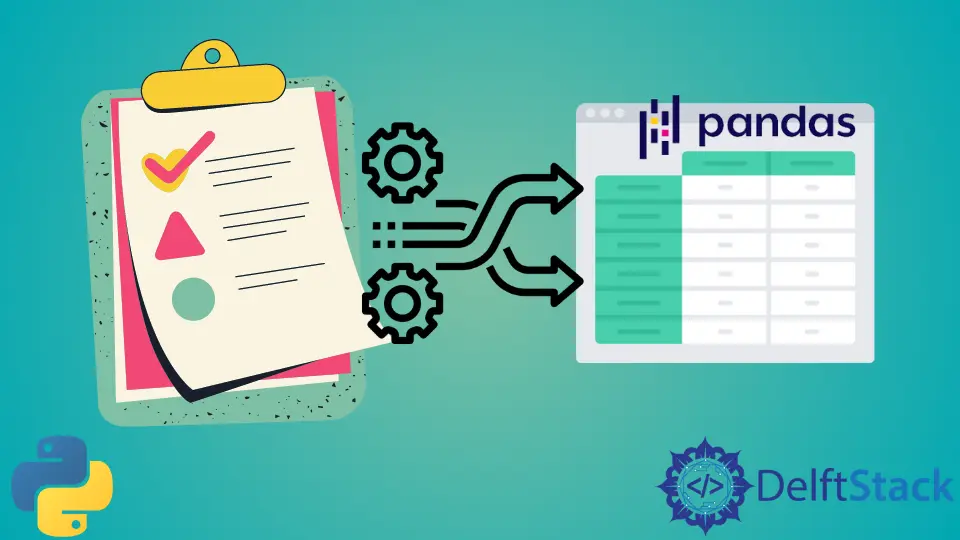
This article will introduce methods to convert items in a list to a Pandas DataFrame.
Convert List to a Pandas DataFrame in Python
Data frame, generally, is a two-dimensional labeled data structure. Pandas is an open-source Python package that is very useful for data science.
Here, we will first import the pandas package. We will define the pandas package as pd
in this particular program. Then we will create a list my_list
to store the list values, Tom
, Mark
, and Tony
, which are nothing but random names. Then we will assign pd.DataFrame(my_list)
to a variable df
. The DataFrame(my_list)
method takes the values of my_list
and creates a data frame with it. In the last line of our program, we called the printed data frame we stored in variable df
. Note that we could also have just written df
instead of print(df)
to see our data frame.
Example Code:
# python 3.x
import pandas as pd
my_list = ["Tom", "Mark", "Tony"]
df = pd.DataFrame(my_list)
print(df)
Output:
0
0 Tom
1 Mark
2 Tony
We can see that the items we provided in the list are now in a column in the above output.
Store the List in a Column in Pandas DataFrame in Python
We can convert a list to the pandas DataFrame by creating a column in the DataFrame and storing the converted data in the column.
To convert a list to a pandas DataFrame column’s data, we will create a list my_list
and give some random names as values to the list. Our goal is to make sure the list elements become the entries of a column titled Names
. For that, we will pass the variable my_list
to pd.DataFrame()
with columns = ['Names']
as below. Then we print the df
variable and run our code to see the output.
Example Code:
# python 3.x
import pandas as pd
my_list = ["Tom", "Mark", "Tony"]
df = pd.DataFrame(my_list, columns=["Names"])
print(df)
Output:
Names
0 Tom
1 Mark
2 Tony
After we put an extra attribute columns = ['Names']
, we see that the names in my_list
went as the values of column Names
in the DataFrame.
Convert a List to a DataFrame With Index in Python
We can also index the list items while converting them to a DataFrame.
We will create a list my_list
. Our goal is to make sure the list elements become column entries titled Names
with predefined row-wise indexes. For that, we will create a list index
and populate it with i
, ii
and iii
. We can use the list as the second parameter in pd.DataFrame()
. The first and the third parameters are my_list
and columns =['Names']
. Then, we will print the variable df
where the expression we wrote is stored.
Example Code:
# python 3.x
import pandas as pd
my_list = [" Tom", "Mark", "Tony"]
df = pd.DataFrame(my_list, index=["i.", "ii.", "iii."], columns=["Names"])
print(df)
Output:
Names
i. Tom
ii. Mark
iii. Tony
We can see that the values inside the list index
have replaced the default pandas indexes. We can put any value inside the index
and produce results accordingly.
Zip Two Lists Into a Single DataFrame Using zip()
in Python
The zip()
function combines the values of two different lists into one by grouping the lists’ values with the same index together. Before we create a DataFrame, let’s see how zip()
works first.
Example Code:
# python 3.x
a = ["1", "2", "3"]
b = ["4", "5", "6"]
c = zip(a, b)
list1 = list(c)
print(list1)
Output:
[('1', '4'), ('2', '5'), ('3', '6')]
We can see that the zip()
function helped us combine the lists a
and b
with similar indexed items grouped. We stored the zipped status of lists a
and b
onto c
and then created list1
, storing the zipped list c
into it. We will use the zip()
to create a pandas DataFrame in the following example.
We will create two different lists, name_list
and height_list
, and store some names and heights,respectively. Then we zip name_list
and height_list
with zip(name_list, height_list)
to create a pandas DataFrame.
Note that we can also index our data by simply putting another attribute index = [ 'index1', 'index2', 'index3' ]
where the items inside the index list can be anything.
Example Code:
# python 3.x
import pandas as pd
name_list = ["Tom", "Mark", "Tony"]
height_list = ["150", "151", "152"]
df = pd.DataFrame((zip(name_list, height_list)), columns=["Name", "Height"])
print(df)
Output:
Name Height
0 Tom 150
1 Mark 151
2 Tony 152
We can see that the formed DataFrame consists of values of both name_list
and height_list
in proper order.
We can also use this technique to zip more than two lists.
Convert a Multi-Dimensional List to a Pandas DataFrame in Python
We can even convert the multi-dimensional list to a pandas DataFrame. We can set the column names for the list items in the multi-dimensional list. We will demonstrate this method with a two-dimensional list.
To convert a multi-dimensional list to a pandas DataFrame, we need to create a list with multiple lists inside first. So we will first import pandas and then create a list info
where we will store the name and age of three different individuals in three separate lists. Then we will call pd.DataFrame()
and process the list into it and specify two column titles, Name
and Age
for our data.
Example Code:
# python 3.x
import pandas as pd
info = [["Tom", 18], ["Mark", 25], ["Tony", 68]]
df = pd.DataFrame(info, columns=["Name", "Age"])
print(df)
Output:
Name Age
0 Tom 18
1 Mark 25
2 Tony 68
We have got two columns as output with the names and their ages in respective orders. We can add other values to the individual lists inside info
and give them column titles to get more columns in our DataFrame.
Related Article - Python Pandas
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python