How to Get Parts of a String in Python
- the Slicing Method in Python
- Get the Left String Using the Slicing Method in Python
- Get the Middle String Using the Slicing Method in Python
- Get the Right String Using the Slicing Method in Python
- Get the Last Element of the String Using the Slicing Method in Python
-
Get the String Using the
Step
Parameter in the Slicing Method in Python
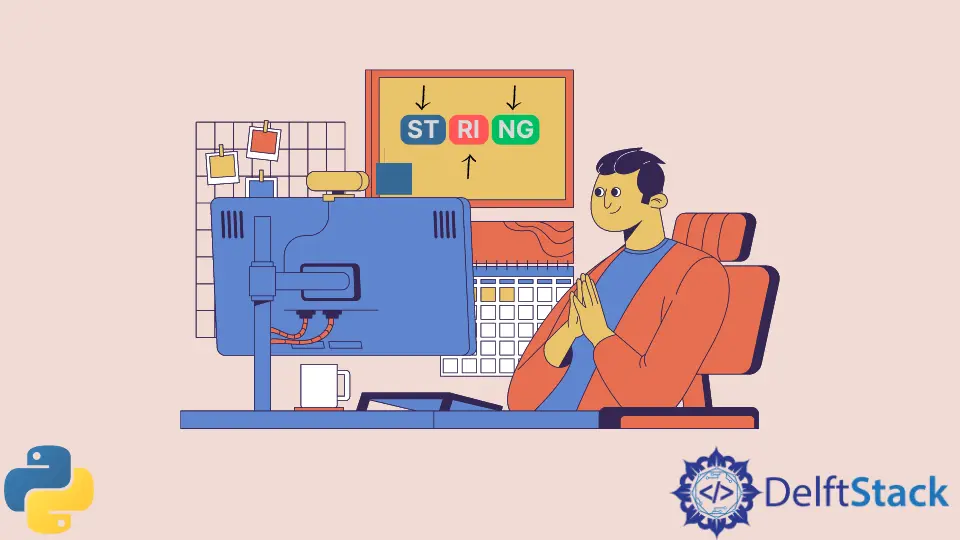
This article will study how to get parts of a string in Python. There are situations when we need to get some of the alphabets or elements of the original string in Python.
For this purpose, we use the substring method, which gives some specific elements of that string. The slicing method is also the same as the substring method.
the Slicing Method in Python
We can use the slicing method to get the specific parts of the list, array, or tuples. We can also use it to delete or modify the particular parts of the strings and lists.
We can specify where we want to start and end the slicing process in Python in the slicing function.
The starting point of the string can be called the left string, the middle part of the string can be called the middle string, and the end of the string can be called the right string. This is based on the positions of strings from their original string.
Get the Left String Using the Slicing Method in Python
The starting point of the string is the initial part that specifies from where we want to start slicing. The starting point or the left string is always specified whenever slicing a string.
If it is not specified, then the default value will be zero.
We also have to specify the end of the string where we want to slice the original string to get a substring. It is essential to determine the ending point of the slice if we’re going to convert the original string into multiple or two substrings.
Because its default value is the second last element of the original string, if we don’t specify the ending point, it will keep the original string as a substring without the last element of the original string.
The step
is the character used in slicing to select the next element of the list or string. If we want to add an increment of 1 element, then step
is 1
, and if we’re going to add 2, then step
is 2
.
Now, let’s talk about using the slicing method with some examples.
If we have a string and want to get the first six elements from that string, we can use the following code below.
# python
originalString = "Slicing the string with Python"
leftString = originalString[0:6]
print("The left string collected is ", leftString)
Output:
As you can see from the above example, by telling Python that the starting point is 0
and the ending point is 6
, we can get the specific part of the string using the slicing method.
We got the left part of the string.
Get the Middle String Using the Slicing Method in Python
Now let’s try to get the middle part of the string using the same function as shown below.
# python
originalString = "Slicing the string with Python"
middleString = originalString[8:18]
print("The middle string collected is ", middleString)
Output:
As you can see, we specified which middle part we wanted as the middle string in Python using the slicing method, and we quickly got that part of the string.
Get the Right String Using the Slicing Method in Python
Now, let’s go through another example in which we will try to get the last part of the original string using the same method as shown below.
# python
originalString = "Slicing the string with Python"
rightString = originalString[19:]
print("The right string collected is ", rightString)
Output:
As you can see from the above example, we didn’t mention the ending point for the slicing method. We only specified the starting point, and it sliced the string until the end because, by default, the value was the last element.
Get the Last Element of the String Using the Slicing Method in Python
Now, suppose we only want to get the last element of the string using the same method. We can quickly achieve this using the following method.
# python
originalString = "Slicing the string with Python"
lastElement = originalString[-1]
print("The last element collected is ", lastElement)
Output:
As you can see in the above example, we can get the last elements of the strings using the negative index.
We used -1
to get the last element of the string. If we decrease the value, such as -5
, we will get the fifth final element of the original string.
Get the String Using the Step
Parameter in the Slicing Method in Python
Now, let’s use the step
as a parameter in our slicing method. In this example, we get the elements by adding step = 2
in the program.
As shown below, we get the elements with a gap of 1 element.
# python
originalString = "Slicing the string with Python"
stepString = originalString[::2]
print("The string we collected by using the step is: ", stepString)
Output:
As you can see from the above example, we skipped 1 element after every element using the step
parameter and got the result string.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn