How to Iterate Over Two Lists in Python
- Iterate Over Two Lists in Python
-
Use the
zip()
Function to Iterate Over Two Lists in Python -
Use the
izip()
Function to Iterate Over Two Lists in Python -
Use the
map()
Function to Iterate Over Two Lists in Python -
Use the
zip_longest()
Function to Iterate Over Two Lists in Python - Conclusion
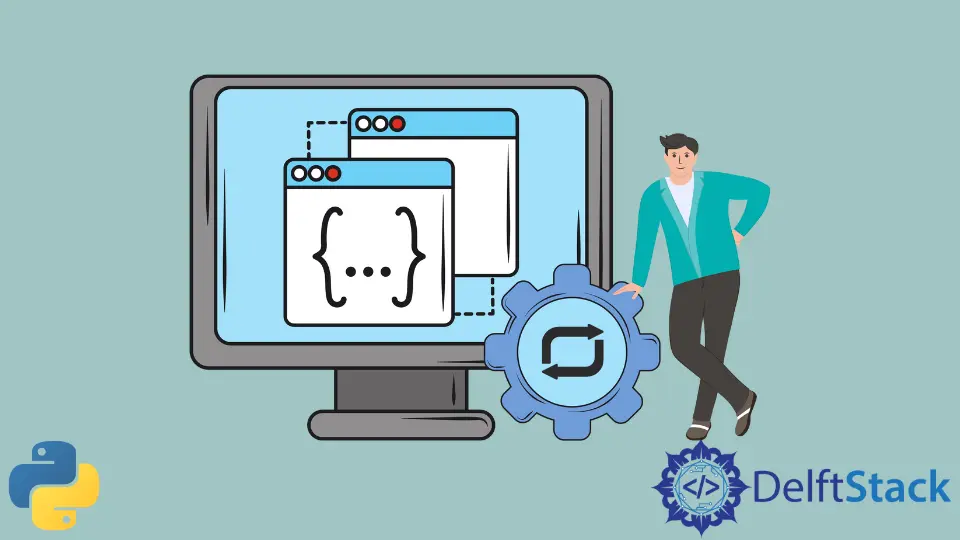
While parsing the data stored in data structures such as a dictionary, tuple, or list, we come across iterations to move over the data sequence stored in it.
Iterating a single data structure like a list in Python is common, but what if we come across a scenario that expects us to iterate over two/multiple lists together?
This article will unveil the different ways to iterate over two lists in Python with some demonstrations.
Iterate Over Two Lists in Python
We can iterate over a single Python list using a for
loop or a range()
function.
The range(start, stop, step)
function enables us to get a sequence of numbers from a defined range of values. It accepts start
, stop
, and step
as input.
Where:
- The
start
index is where therange()
function starts to iterate and get the numbers or elements. - The
stop
index determines the position or integer where the iteration of the sequence of elements should stop. - The
step
index is an optional parameter that allows us to include patterns such as increments in the iteration of the sequence of elements in the list.
Consider the example below. We declare a list with some arbitrary values and then loop in the list using any in-built function such as range()
.
lst = [10, 2, 0, 1]
for x in range(0, 4):
print("Iteration through a single list:", lst[x])
Output:
Here, we have passed the start
index to the function as 0 so that it starts to get the sequence of elements from the list right from position 0, i.e., the first element.
The stop
index is set as 4, equivalent to the list length so that the range()
function iterates the sequence until the last element and displays it.
Use the zip()
Function to Iterate Over Two Lists in Python
In the realm of Python programming, efficiently handling multiple data sequences is a common requirement. One effective technique is to iterate over two lists simultaneously, and [the zip()
function]({{relref “/HowTo/Python/zip python 3.en.md”}}) is a powerful tool for this task.
The primary purpose of zip()
is to aggregate elements from two or more iterables (like lists, tuples, etc.) and return an iterator of tuples. Each tuple contains elements from the corresponding positions of the iterables.
Syntax and Parameters
The basic syntax of zip()
is as follows:
zip(iterable1, iterable2, ...)
iterable1, iterable2, ...
: These are the iterables you want to combine. You can pass two or more iterables, and they don’t necessarily need to be of the same type (e.g., a list and a tuple can be zipped together).
Let’s dive into a practical example. Suppose we have two lists, one with student names and another with their corresponding grades.
student_names = ["Alice", "Bob", "Charlie"]
student_grades = ["A", "B", "C"]
for name, grade in zip(student_names, student_grades):
print(f"{name}: {grade}")
In this snippet, we first define two lists: student_names
and student_grades
. Using the zip()
function, we combine these lists.
The for
loop then iterates over this zipped object. On each iteration, name
and grade
are assigned values from the corresponding positions in the two lists.
We use a formatted string (f-string
) inside the print
function for a cleaner display of the output. This approach ensures that each student’s name is paired with their respective grade, a process that is both efficient and easy to understand.
Output:
This result demonstrates how zip()
effectively pairs each name with the matching grade.
Use the izip()
Function to Iterate Over Two Lists in Python
To use a version before Python 3.0, we use the izip()
function instead of the zip()
function to iterate over multiple lists.
The izip()
function also expects a container, such as a list or string, as input. It iterates over the lists until the smallest of them gets exhausted.
It then zips or maps the elements of both lists together and returns an iterator
object. It returns the elements of both lists mapped together according to their index.
Syntax and Parameters (Python 2)
The basic syntax of izip()
in Python 2’s itertools
module is:
itertools.izip(iterable1, iterable2, ...)
iterable1, iterable2, ...
: These are the iterables (like lists, tuples) to be combined.
Let’s see an example using izip()
to iterate over two lists.
import itertools
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
for item1, item2 in itertools.izip(list1, list2):
print(item1, item2)
In this code, we first import the itertools
module. We then define two lists, list1
and list2
.
By calling itertools.izip(list1, list2)
, we create an iterator that pairs up elements from list1
and list2
. The for
loop then iterates over these pairs, and item1
and item2
receive values from corresponding positions in list1
and list2
, respectively.
Output:
This output illustrates how each element from list1
is paired with the corresponding element from list2
. izip()
, therefore, provides an efficient way to iterate over multiple lists in parallel, especially in scenarios where memory optimization is crucial.
Use the map()
Function to Iterate Over Two Lists in Python
While it’s commonly used with a single iterable, map()
can be an effective way to iterate over two (or more) lists simultaneously. This approach can be particularly useful when you need to apply a function to corresponding elements of multiple lists.
The map()
function accepts iterable data structures such as lists and strings as input along with a function. After applying the specified function, it maps the iterable data elements and returns a tuple after mapping the values.
Syntax and Parameters
The basic syntax of map()
is as follows:
map(function, iterable1, iterable2, ...)
function
: A function to which the items from the iterables will be passed. This function should accept as many arguments as there are iterables.iterable1, iterable2, ...
: These are the iterables whose elements are to be passed to the function.
Let’s consider an example where we have two lists, one with numbers and another with their string representations, and we want to create a list of tuples pairing these elements.
def pair_elements(elem1, elem2):
return (elem1, elem2)
numbers = [1, 2, 3]
strings = ["one", "two", "three"]
result = list(map(pair_elements, numbers, strings))
for item in result:
print(item)
In this code, we define a function pair_elements
that takes two arguments and returns a tuple containing these arguments. We have two lists, numbers
and strings
.
We then use map()
to apply pair_elements
to each pair of elements from numbers
and strings
, creating an iterable of tuples. By converting this iterable to a list (list(map(...))
), we make it easy to iterate over and print its contents.
Output:
This result demonstrates how map()
effectively pairs each number with its corresponding string representation.
Use the zip_longest()
Function to Iterate Over Two Lists in Python
The zip_longest()
function is a replacement for the map()
function available in Python version 2. It accepts iterable
objects such as lists and strings as input.
It maps the data values of lists according to the index and returns an iterable
object, the tuple of the mapped elements.
Similarly, it iterates over two lists until all the elements of both lists are exhausted. Also, in the output, it maps an element of list 01
with another element of list 02
.
If one of the lists gets exhausted of elements, it replaces them with None
.
Syntax and Parameters
The syntax of itertools.zip_longest()
is:
itertools.zip_longest(*iterables, fillvalue=None)
*iterables
: One or moreiterable
objects (like lists or tuples).fillvalue
: An optional parameter specifying what value should be used for missing values. The default isNone
.
Consider an example where we have two lists of different lengths, and we want to pair their elements.
import itertools
list1 = [1, 2, 3, 4]
list2 = ["a", "b", "c"]
for number, letter in itertools.zip_longest(list1, list2, fillvalue="N/A"):
print(number, letter)
In the given code, we first import the itertools
module. We define two lists, list1
and list2
, of different lengths.
Using itertools.zip_longest()
, we create an iterator that pairs up elements from these lists. If one list is shorter, the fillvalue
(N/A
in our case) is used to substitute missing values.
Output:
This output shows how itertools.zip_longest()
pairs each element from the two lists and uses N/A
for the missing elements in the shorter list (list2
).
Conclusion
In conclusion, iterating over two lists in Python can be achieved through various methods, each suited to different scenarios. We explored the zip()
function for pairing elements of equal-length lists, itertools.izip()
in Python 2 for memory efficiency, the map()
function for applying a function to elements of multiple lists, and itertools.zip_longest()
for handling lists of unequal lengths.
Each method has its unique syntax and parameters, catering to specific needs - zip()
for simple pairing, izip()
for large data sets in older Python versions, map()
for element-wise function applications, and zip_longest()
for pairing with placeholder values in mismatched-length lists. Understanding these methods enhances your ability to handle multiple data sequences in Python effectively.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python