How to Reverse a List in Python
-
Use
range()
to Reverse a List in Python -
Reverse a List by the
while
Loop in Python - Reverse a List Using the Slice Operator in Python
-
Use
reversed()
to Reverse a List in Python
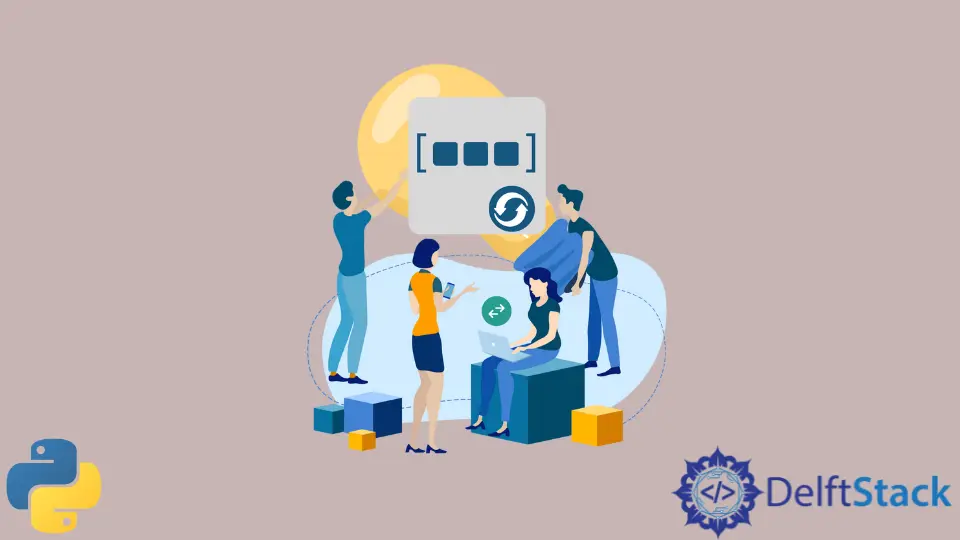
This tutorial will demonstrate different ways of how to reverse a list in Python.
List reversal is one of the most common starter programming problems you experience when learning to program. In Python, there several easy ways to reverse a list.
Use range()
to Reverse a List in Python
range()
is a Python built-in function that outputs a list of a range of numbers.
Syntax of range()
range(start, stop, step)
This function has 3 arguments; the main required argument is the second argument stop
, a number denoting where you want to stop. There are 2 optional arguments, start
specifies where you should start counting and step
specifies the incrementation of the sequence.
Take note that stop
will have an offset of 1 since the counting starts at 0
. To create a list that stops at 5 using range()
, the stop value would have to be 6.
numbers = list(range(6))
print(numbers)
Output:
[0, 1, 2, 3, 4, 5]
To reverse this list, then you have to specify the start
and step
arguments.
The start
is set to 5, while step
is -1
since we want to decrement the range by 1 each time. The stop
argument should also be set to -1
, since we want to stop at 0
(Since stop
has an offset of 1).
numbers = list(range(5, -1, -1))
print(numbers)
Output:
[5, 4, 3, 2, 1, 0]
Reverse a List by the while
Loop in Python
Declare a list of 10 random integers that we want to create a new list in reverse order.
numbers = [66, 78, 2, 45, 97, 17, 34, 105, 44, 52]
Use a while
loop over the list to output it in reverse. First, get the size of the list and deduct it by 1 to point to the last element of the list. Let’s also declare an empty list to store the new reversed version of the previous list.
idx = len(numbers) - 1
newList = []
Now use the while
loop to iterate and store each element in the new list with each iteration decrementing idx
until it hits 0
.
while idx >= 0:
newList.append(numbers[idx])
idx = idx - 1
print(newList)
Output:
[52, 44, 105, 34, 17, 97, 45, 2, 78, 66]
Reverse a List Using the Slice Operator in Python
If you prefer not to loop over the list, then use the slice
operator to decrement the array index by 1.
Similar to range()
, the slice operator accepts three arguments: start
, stop
, and step
.
Leave the first two arguments blank so it will cover the whole array and set the step
value to -1
so it starts with at the end of the array and decrements it by 1 each time.
newList = numbers[::-1]
print(newList)
Output:
[52, 44, 105, 34, 17, 97, 45, 2, 78, 66]
Use reversed()
to Reverse a List in Python
Another easy way to reverse a list in Python is to use the built-in function reversed()
. This function accepts a list argument and returns an iterator of the reversed version of the same list.
Using the same example numbers
above, reverse the list using this function. Don’t forget to wrap the function with list()
to actually store the return value of reversed()
into a list.
newList = list(reversed(numbers))
print(newList)
Alternatively, you can also use a for
loop to iterate over the reversed list and store it directly in newList
.
newList = [num for num in reversed(numbers)]
print(newList)
The output of both solutions will be the same.
[52, 44, 105, 34, 17, 97, 45, 2, 78, 66]
In summary, Python provides a simple way to reverse a list by making use of the function reversed()
. You also can reverse a list manually by looping it in a for
or while
loop. Python also has an easy method of reversing a list in a single line if you are comfortable using the slice operator.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python