How to Loop Through Multiple Lists in Python
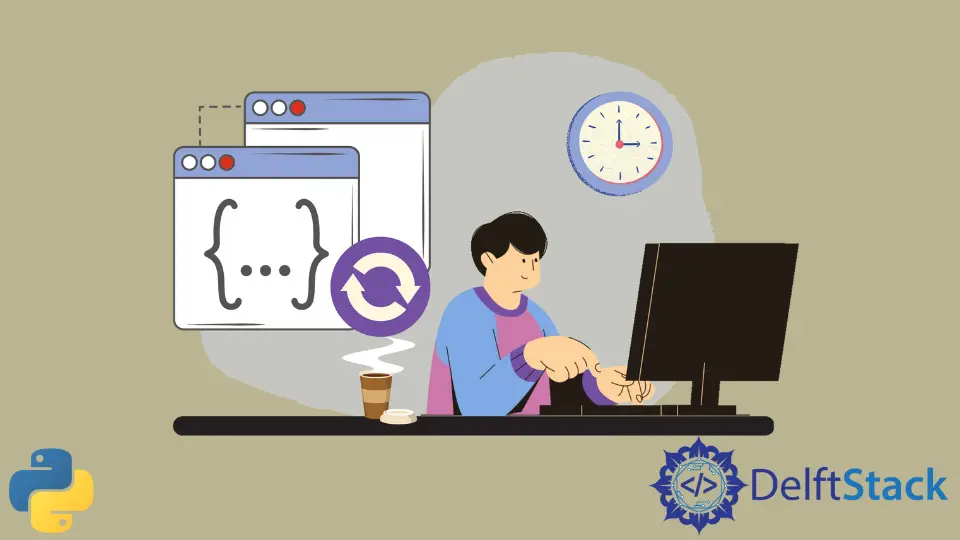
This tutorial explains how to iterate through two lists/tuples at the same time in Python. We will use zip()
and itertools.zip_longest()
and explain the differences between them and how to use each one. We’ll also see how [the zip()
function]({{relref “/HowTo/Python/zip python 3.en.md”}}) return type is different in Python 2 and 3.
zip()
Function in Python 3.x
zip()
function accepts multiple lists/tuples as arguments and returns a zip
object, which is an iterator of tuples.
Use zip()
to Iterate Through Two Lists
Pass both lists to the zip()
function and use for loop to iterate through the result iterator.
listA = [1, 2, 3, 4]
listB = [10, 20, 30, 40]
for a, b in zip(listA, listB):
print(a, b)
Output:
1 10
2 20
3 30
4 40
Use zip()
to Iterate Through Two Lists With Different Lengths
If lists have different lengths, zip()
stops when the shortest list end. See the code below.
listA = [1, 2, 3, 4, 5, 6]
listB = [10, 20, 30, 40]
for a, b in zip(listA, listB):
print(a, b)
Output:
1 10
2 20
3 30
4 40
Use itertools.zip_longest()
to Iterate Through Two Lists
If you need to iterate through two lists till the longest one ends, use itertools.zip_longest()
. It works just like the zip()
function except that it stops when the longest list ends.
It fills the empty values with None
, and returns an iterator of tuples.
import itertools
listA = [1, 2, 3, 4, 5, 6]
listB = [10, 20, 30, 40]
for a, b in itertools.zip_longest(listA, listB):
print(a, b)
Output:
1 10
2 20
3 30
4 40
5 None
6 None
The default fillvalue
is None
, but you can set fillvalue
to any value.
import itertools
listA = [1, 2, 3, 4, 5, 6]
listB = [10, 20, 30, 40]
for a, b in itertools.zip_longest(listA, listB, fillvalue=0):
print(a, b)
Output:
1 10
2 20
3 30
4 40
5 0
6 0
Use zip()
With Multiple Lists
zip()
and its sibling functions can accept more than two lists.
import itertools
codes = [101, 102, 103]
students = ["James", "Noah", "Olivia"]
grades = [65, 75, 80]
for a, b, c in itertools.zip_longest(codes, students, grades, fillvalue=0):
print(a, b, c)
Output:
101 James 65
102 Noah 75
103 Olivia 80
zip()
Function in Python 2.x
zip()
function in Python 2.x also accepts multiple lists/tuples as arguments but returns a list of tuples. That works fine for small lists, but if you have huge lists, you should use itertools.izip()
instead, because it returns an iterator of tuples.
Use itertools.izip()
to Iterate Through Two Lists
import itertools
listA = [1, 2, 3, 4]
listB = [10, 20, 30, 40]
for a, b in itertools.izip(listA, listB):
print(a, b)
Output:
1 10
2 20
3 30
4 40
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python