How to Flatten a List in Python
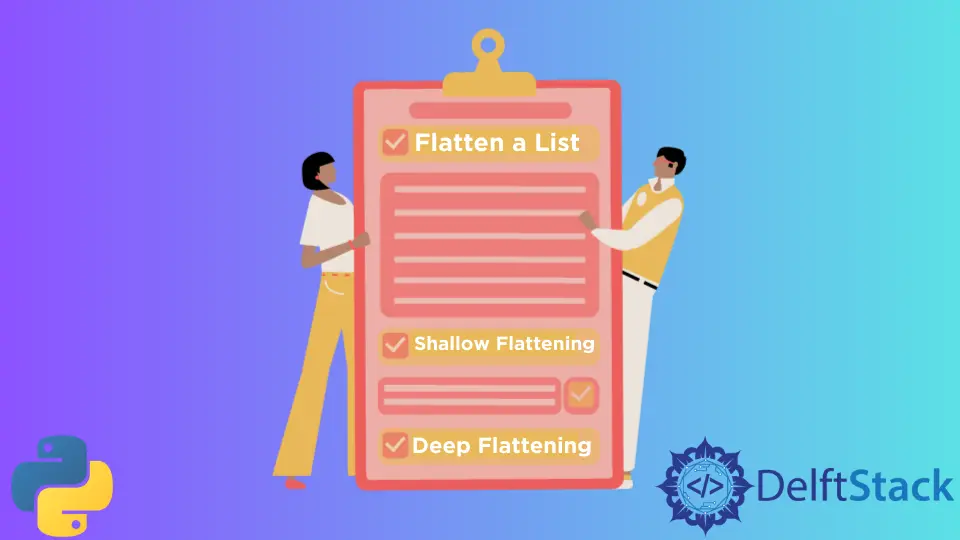
Today, we’re going to explore the process of flattening nested lists in Python, breaking it down into simple terms that everyone can grasp. Flattening, essentially, means taking a list that contains other lists and turning it into a new structure that has no nested lists.
This resulting structure is often referred to as a flat or flattened list.
The original list might have lists nested within it, but only at the first level—like this: [[1, 2], [3, 4]]
. On the other hand, in more complex scenarios, we can have lists within lists within lists, creating deeper levels of complexity, such as [[[1], [2]], [3, 4]]
.
In this case, we’re dealing with what we call deeply nested lists.
Now, if we’re aiming to just deal with the first level of nesting, we call it shallow flattening. Conversely, if our goal is to unwrap all levels of nesting, we call it deep flattening.
Understanding and mastering these concepts allows us to effectively handle and work with complex data structures.
Python Flatten List (Shallow Flattening)
Flattening a list, especially when it contains nested lists, is a common operation in Python. It involves converting a list with sublists into a single flat list.
There are multiple approaches to achieve this, and we’ll explore them in detail to provide a comprehensive understanding.
Shallow Flattening Using the Double for
Loop Method
A straightforward way to flatten a list is by using a double for
loop. This method utilizes two nested for
loops to iterate through the original list and its sublists.
During each iteration, the method appends the elements of the sublists to a result flat list.
Here’s a breakdown of the steps involved in using the double for
loop method to flatten a list:
-
Create an empty list that will store the flattened elements.
-
Use the first
for
loop to traverse the original list, which contains nested sublists. -
In the inner loop, append each element of the sublist to the result flat list.
-
After both loops complete their iterations, you’ll have a flat list containing all the elements from the nested sublists.
Now, let’s walk through a practical Python code example to illustrate this method.
flat_list = []
original_list = [[1, 2], [3, 4]]
for sublist in original_list:
for item in sublist:
flat_list.append(item)
print("Flattened List:", flat_list)
Output:
Flattened List: [1, 2, 3, 4]
We start by initializing an empty list called flat_list
and another list called original_list
containing two sublists, each with two elements.
To extract all the individual elements and store them in a single, one-dimensional list, we use a nested loop structure. The outer loop iterates over each sublist in original_list
, and the inner loop iterates over each item within the sublists.
For each item, it appends it to the flat_list
.
In other words, the code goes through each sublist (e.g., [1, 2]
and [3, 4]
), then for each sublist, it iterates through the items (e.g., 1, 2, 3, and 4), appending them to the flat_list
. After this process, flat_list
contains all the individual elements from the sublists in a flattened manner.
The output shows the flattened list, [1, 2, 3, 4]
, which is the result of the flattening operation performed by the code.
Shallow Flattening Using List Comprehensions
Python’s list comprehensions provide a concise and elegant way to achieve the same flattening outcome in just one line of code. The basic structure of a list comprehension includes an expression, followed by at least one for
clause, and optionally one or more if
clauses. You can read more details from this one line for
loop article.
This structure allows you to iterate over an iterable and apply expressions to filter or transform the elements. List comprehensions are efficient and often faster than traditional loops due to their optimized implementation in Python.
To flatten a list using list comprehensions, we’ll iterate through the original list and its sublists, appending the elements to a new list.
Follow these steps to flatten a list using list comprehensions:
-
Begin by writing the list comprehension syntax, specifying the expression and the
for
loop to iterate over the original list and sublists. -
In the expression part of the list comprehension, append each element of the sublist to the new list.
-
Obtain the flattened list. The list comprehension will generate a new flat list containing all the elements from the nested sublists.
Let’s walk through a practical Python code example to demonstrate how to use list comprehensions to flatten a list.
original_list = [[1, 2], [3, 4]]
flat_list = [item for sublist in original_list for item in sublist]
print("Flattened List:", flat_list)
Output:
Flattened List: [1, 2, 3, 4]
Here, we flattened a list of lists, converting a two-dimensional list into a one-dimensional list. The original list, original_list
, contains two sublists: [1, 2]
and [3, 4]
(similar to the previous example).
We then used a list comprehension to iterate over each sublist (sublist
) within the original_list
. For each sublist
, it further iterates over its elements (item
) and appends them to a new list called flat_list
.
This process effectively flattens the nested sublists into a single list. After the flattening process, the code prints the message Flattened List:
followed by the resulting flattened list (flat_list
).
Shallow Flattening Using itertools.chain()
If you need an iterator to traverse the elements as if they were from a single flat data structure, itertools.chain()
is a useful tool. This function is part of the Python itertools
module, which provides a collection of tools for handling iterators and combining them efficiently.
itertools.chain()
takes multiple iterable arguments and returns an iterator over the elements of each iterable in sequence. This makes it a perfect approach for flattening nested lists.
Here are the steps involved in using itertools.chain()
to flatten a list:
-
Begin by importing the
itertools
module, which contains thechain()
function. -
Pass the nested list to the
chain()
function, using the*
operator to unpack the list and provide its elements as arguments. This will effectively flatten the list. -
Iterate over the resulting iterator to access each element in the flattened list.
Let’s go through a practical Python code example to illustrate how to use itertools.chain()
to flatten a list.
import itertools
original_list = [[1, 2], [3, 4]]
flattened_iterator = itertools.chain(*original_list)
for item in flattened_iterator:
print(item)
Output:
1
2
3
4
In the code above, we first imported itertools
to access its functions. Then, we created an original_list
with two sublists.
Now, the magic happened with itertools.chain(*original_list)
. The chain()
function took the sublists and merged them into a single iterator.
We then ran a for
loop on this flattened iterator. For each item in the iterator, we simply printed it to the console.
In this case, we printed each item on a new line. The flattened iterator contains the elements 1
, 2
, 3
, and 4
, and the loop prints each one on a separate line.
Shallow Flattening Using itertools.chain.from_iterable()
The itertools.chain.from_iterable()
function is an alternative to itertools.chain()
. It takes an iterable of iterables and flattens it into a single iterator, yielding elements from the sub-iterables consecutively.
This function is particularly useful for flattening nested data structures.
Steps to flatten a list using itertools.chain.from_iterable()
:
-
Begin by importing the
itertools
module, which contains thechain
function. -
Create the original list containing nested sublists that need to be flattened.
-
Pass the nested list to
chain.from_iterable()
to obtain an iterator that yields each element from the sublists in a flattened sequence. -
Iterate over the resulting iterator to access each element in the flattened list.
Let’s have a code example to illustrate how to use itertools.chain.from_iterable()
to flatten a list.
import itertools
original_list = [[1, 2], [3, 4]]
flattened_iterator = itertools.chain.from_iterable(original_list)
for item in flattened_iterator:
print(item)
Output:
1
2
3
4
In this Python code, we began by importing the itertools
module. We have a list called original_list
, which contains two sublists, each holding pairs of numbers.
To flatten this list of lists, we used the itertools.chain.from_iterable
method. This method took our original_list
and flattened it into an iterator.
The from_iterable
method concatenated the sublists, creating an iterator that gave us each number in order. We then used a for
loop to go through this iterator, printing each item.
Shallow Flattening Using the flatten()
Function in Pandas
The flatten()
function is a method provided by Pandas
, specifically available in the pandas.core.common
module. This function simplifies the process of flattening a list or any iterable containing iterables, converting a nested list into a single flat list or iterable.
By using this function, you can streamline data processing and make it more efficient, especially when dealing with complex data structures.
Syntax of the flatten()
function:
flatten(iterable)
iterable
: The iterable object (e.g., a list, tuple, etc.) that you want to flatten.
The flatten()
function returns an iterator over the elements of the iterable in a flattened sequence.
Steps to flatten a list using the flatten()
function:
-
Begin by importing the necessary libraries, including the
Pandas
library and theflatten()
function. -
Create the original list containing nested sublists that need to be flattened.
-
Utilize the
flatten()
function to flatten the original list and convert it into a single-dimensional structure. -
Optionally, you can convert the flattened structure to a regular Python list using the
list()
function. -
You can now print the flattened list or further use it in your data analysis or processing.
The flatten()
function is straightforward to use and eliminates the need for writing custom flattening code.
Let’s illustrate the usage of the flatten()
function from Pandas
to flatten a list:
from pandas.core.common import flatten
original_list = [[1, 2], [3, 4], [5, 6, 7]]
flattened_list = flatten(original_list)
flat_list = list(flattened_list)
print("Flattened List:", flat_list)
Output:
Flattened List: [1, 2, 3, 4, 5, 6, 7]
We started by importing the flatten()
function from the pandas.core.common
module. Next, we created an original_list
containing nested lists, each with varying numbers of elements.
In this case, we have a list with sublists containing 2, 2, and 3 elements, respectively.
We then used the flatten()
function to flatten the original_list
, resulting in a single-dimensional iterable object. To convert this iterable to a list, we used the list()
function and stored the flattened list in a variable called flat_list
.
Finally, we printed a message indicating that we had successfully flattened the list, along with the flattened list itself.
Let’s have another example demonstrating that the flatten()
function can also handle a tuple of lists:
from pandas.core.common import flatten
tuple_of_lists = ([1, 2], [3, 4], [5, 6, 7])
flattened_iterator = flatten(tuple_of_lists)
flattened_list = list(flattened_iterator)
print("Flattened List:", flattened_list)
Output:
Flattened List: [1, 2, 3, 4, 5, 6, 7]
Initially, we imported the flatten
function from the pandas.core.common
module, a function specialized in flattening nested iterables like tuples of lists.
We have a tuple_of_lists
containing three lists as its elements, representing a tuple with nested lists. To achieve a flattened structure from this nested arrangement, we applied the flatten
function to the tuple_of_lists
.
This function yielded an iterator that traverses through the elements in a flattened sequence.
Subsequently, to obtain a flattened list, we converted the iterator into a list utilizing the list
function and stored the result in flattened_list
. Now, flattened_list
holds all the elements from the nested lists, organized in a flattened, one-dimensional structure.
Lastly, we printed the flattened list alongside a descriptive message employing the print
function.
Python Flatten List (Deep Flattening)
Shallow flattening, as showcased in the provided example, unnests only the first level of nestedness in a list. For deeply nested lists, this method falls short and fails to flatten the structure entirely.
Let’s revisit the example of using list comprehensions to flatten a deeply nested list to understand this:
deeply_nested_list = [[[1, 2], 3], [4, 5, 6]]
flat_list = [item for l in deeply_nested_list for item in l]
print("Flattened List:", flat_list)
Output:
Flattened List: [[1, 2], 3, 4, 5, 6]
In this shallow flattening attempt, the first level of nesting is removed, but the subsequent levels remain intact, resulting in a partially flattened list.
Deep Flattening Using iteration_utilities
To achieve deep flattening, we can leverage the deepflatten()
function from the iteration-utilities
PyPI package. This function effectively flattens deeply nested lists, ensuring all levels of nesting are removed.
To proceed, start by installing the iteration-utilities
package using pip
:
$ pip install iteration-utilities
Next, import the deepflatten()
function from iteration_utilities
and apply it to your deeply nested list:
from iteration_utilities import deepflatten
deeply_nested_list = [[[1, 2], 3], [4, 5, 6]]
flat_list = list(deepflatten(deeply_nested_list))
print("Flattened List:", flat_list)
Output:
Flattened List: [1, 2, 3, 4, 5, 6]
Here, we started by importing the deepflatten()
function from the iteration_utilities
module. Next, we defined a variable called deeply_nested_list
, which is a list containing multiple levels of nesting.
We have sublists within sublists, some with numeric elements and others with additional sublists.
We then applied the deepflatten()
function to the deeply_nested_list
, effectively flattening it into a single-dimensional iterable object. To convert this iterable into a regular list, we used the list()
function and stored the flattened list in a variable named flat_list
.
Finally, we printed a message indicating that we had successfully flattened the list, along with displaying the flattened list itself.
Deep Flattening Using Recursive Function
A recursive approach is another way to flatten a list, especially when dealing with arbitrarily nested lists. This involves defining a function that calls itself in order to solve a smaller instance of the problem.
In the context of flattening a nested list, the recursive function traverses through the elements, and if an element is itself a list, it calls itself on that list. This process continues until all levels of nesting are flattened.
Here are the steps to implement this approach:
-
Start by defining a recursive function, such as
flatten
, that takes a list as input. -
Inside the
flatten
function, iterate through each element in the list. -
For each element, check if it is a list (i.e., a nested list) using the
isinstance()
function. -
If an element is a list, call the
flatten
function recursively on that list. This step handles the flattening of nested lists. -
If an element is not a list, append it to a new list, which will hold the flattened elements.
-
Finally, return the fully flattened list, which is the result of the recursion.
Here’s a code snippet illustrating these steps:
def flatten(lst):
flattened = []
for item in lst:
if isinstance(item, list):
flattened.extend(flatten(item))
else:
flattened.append(item)
return flattened
nested_list = [[1, 2, [3]], [4, [5]], [6, 7, [8, 9]]]
flattened_list = flatten(nested_list)
print("Flattened List:", flattened_list)
Output:
Flattened List: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this code, the flatten
function is defined to take one argument, lst
, which is the nested list we want to flatten. Inside the function, we initialized an empty list called flattened
to store the flattened elements.
We then used a for
loop to iterate through each item in the input list lst
. For each item, it checks if it is a list using the isinstance
function.
If the item is a list, it recursively calls the flatten
function on that sublist and extends the flattened
list with the result. This recursive approach is essential for handling multiple levels of nesting.
If the item is not a list, it means it’s a single value, so it is directly appended to the flattened
list.
Once all the elements in the nested list are processed, the flatten
function returns the flattened
list, which contains all the elements in a flat structure.
In the code, there’s a predefined nested_list
that represents a list containing other lists. The flatten
function is called with nested_list
as the input, and the result is stored in the flattened_list
.
Finally, we printed the flattened list with the message: Flattened List
, so you can see the result.
Conclusion
Flattening a list in Python is a common operation when dealing with nested data structures. We explored various methods to achieve this, including double for
loops, list comprehension, using itertools.chain
and itertools.chain.from_iterable
, iteration_utilities
, and a recursive approach.
Choose the method that best fits your use case and helps simplify your data for further processing and analysis.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python