How to Copy a File in Python
-
Shutil
copyfile()
Method to Copy File in Python -
Shutil
copy()
Method to Copy File With Permissions -
Comparison Between
copy()
andcopyfile()
method -
copyfileobj()
Method to Copy File Object -
copy2()
Method to Preserve Metadata - Conclusion of Methods of Copying a File in Python
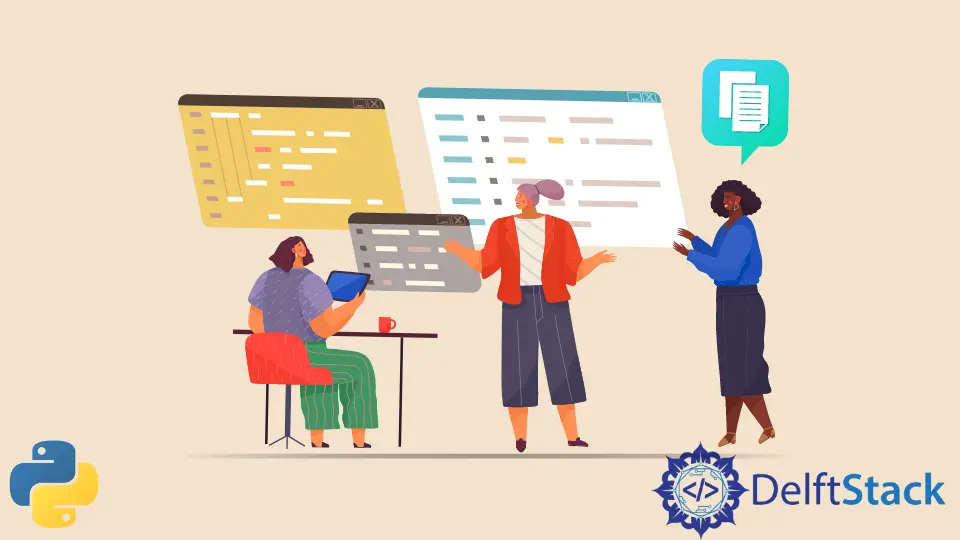
Python comes with several out-of-box modules to support file I/O operations (such as OS
, subprocess
, and shutil
). You will simply copy files and directories using the shutil
module. Operations such as duplication, transferring, or deleting files and directories are completed using this utility function. Here four methods are discussed as follows.
Shutil copyfile()
Method to Copy File in Python
It copies the source material to a destination-named file. If the target is not writable, an IOError
exception will occur in the copy process. If the origin and target files are the same, SameFileError
returns. The pathnames of the source and destination are provided as strings.
copyfile(source_file, destination_file)
Character or block devices and the pipes are not supported by this method.
import shutil
source = r"C:\Users\DelftStack\Documents\test\test.txt"
destination = r"C:\Users\DelftStack\Pictures\test2\test2.txt"
shutil.copyfile(source, destination)
Shutil copy()
Method to Copy File With Permissions
The copy()
method works like the Unix command cp
. Upon copying its content, this method synchronizes the permissions of the target file with the source file. If you are copying the same file, it also throws the SameFileError
. Its syntax is as follows.
shutil.copy(src_file, dest_file, *, follow_symlinks=True)
Example of Shutil copy()
Method to Copy File With Permissions
import shutil
src = r"C:\Users\DelftStack\Documents\test\test.txt"
des = r"C:\Users\DelftStack\Pictures\test2\test2.txt"
shutil.copy(src, des)
Comparison Between copy()
and copyfile()
method
- The
copy()
method often sets the file permission as the contents are copied, while thecopyfile()
copies the data only. - Essentially, in its implementation, the
copyfile()
method uses thecopyfileobj()
method. In comparison, thecopy()
method uses the functionscopyfile()
andcopymode()
. - The above point makes it clear that
copyfile()
will be a little faster thancopy()
because there is an extra job at hand with the latter (preservation of permissions).
copyfileobj()
Method to Copy File Object
This function copies the file to the object of the target path or file. If the target object is a file object, you need to close it after calling copyfileobj()
directly. The buffer size is used to specify the length of the buffer. It is the number of bytes retained in memory during the process of copying.
The syntax of the copyfileobj()
method is below.
shutil.copyfileobj(src_file_object, dest_file_object[, length])
import shutil
filename1 = r"C:\Users\DelftStack\Documents\test\test.txt"
fileA = open(filename1, "rb")
filename2 = r"C:\Users\DelftStack\Pictures\test2\test2.txt"
fileB = open(filename2, "wb")
shutil.copyfileobj(fileA, fileB)
copy2()
Method to Preserve Metadata
The copy2()
method is similar to the copy()
method, but in addition to copying the file contents, it also preserves all the metadata of the source file.
shutil.copy2(src_file, dest_file, *, follow_symlinks=True)
import shutil
src = r"C:\Users\DelftStack\Documents\test\test.txt"
des = r"C:\Users\DelftStack\Pictures\test2\test2.txt"
shutil.copy2(src, des)
Conclusion of Methods of Copying a File in Python
In the end, a table is attached for all the methods and helps understand the shutil
utility better.
Function | copies metadata | copies permissions | can use buffer | destination may be directory |
---|---|---|---|---|
shutil.copy |
No | Yes | No | Yes |
shutil.copyfile |
No | No | No | No |
shutil.copy2 |
Yes | Yes | No | Yes |
shutil.copyfileobj |
No | No | Yes | No |