7 Ways to Concatenate Two or More Lists in Python
-
Concatenate Lists Using the
append()
Method in Python -
Concatenate Lists Using the
+
Operator in Python -
Concatenate Lists Using the
extend()
Method in Python -
Concatenate Lists Using the
+=
Operator in Python - Concatenate Lists Using Packing and Unpacking in Python
-
Concatenate Lists Using the
itertools.chain(
) Function in Python - Concatenate Lists Using List Comprehension in Python
- What Method Should You Use to Concatenate Lists in Python?
- Conclusion
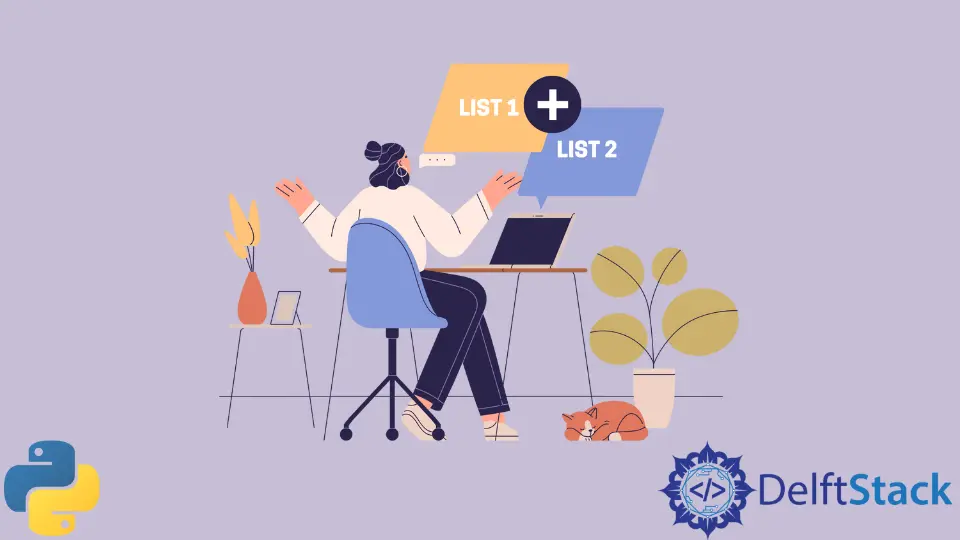
In Python, we use lists in almost every program. While working with Python lists, we need to concatenate two or more lists on several occasions.
In this article, we will look at various ways to concatenate two lists in Python. We will also discuss the efficiency of all the approaches in terms of time and memory usage so that you can choose the best way to concatenate lists in your programs in Python.
Concatenate Lists Using the append()
Method in Python
The first way to concatenate two lists in Python is to use a for loop with the append()
method. When executed on a list, the append()
method accepts an element as its input argument and adds it to the existing list, as shown in the following example.
oldList = [1, 2, 3, 4, 5, 6, 7]
element = 8
print("The existing list is:", oldList)
print("The element to be appended is:", element)
oldList.append(element)
print("The modified list is:", oldList)
Output:
The existing list is: [1, 2, 3, 4, 5, 6, 7]
The element to be appended is: 8
The modified list is: [1, 2, 3, 4, 5, 6, 7, 8]
The append()
method is normally used to add a single element to an existing list. To concatenate two lists using the append()
method, we will add each element of the second list to the first list using the append()
method and a for
loop as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
for element in list2:
list1.append(element)
print("The concatenated list is:", list1)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Here, we have defined list1
and list2
. After that, we have appended each element of list2
to list1
. In this way, we have concatenated the two lists using the append()
method. If you don’t want to modify the existing lists, you can concatenate the lists by creating a new empty list and adding the elements of the existing lists to the empty list as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
newList = list()
lists = [list1, list2]
for myList in lists:
for element in myList:
newList.append(element)
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Here, you can observe that we have created the concatenated list in a new list named newList
. After that, we have declared a list of the existing lists and named it lists
. Then, we have appended each element from the existing lists to the newList
using the for loop
and the append()
method. In this approach, you can also concatenate more than two lists at once. For that, you just need to add the extra lists that need to be concatenated to lists
.
Concatenate Lists Using the +
Operator in Python
Instead of using the append()
method, we can use the +
operator to concatenate two lists in Python. Just like we concatenate two strings using the +
operator in Python, we can concatenate two lists as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
newList = list1 + list2
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Here, we have stored the concatenated list in a third list named newList
. If you don’t need list1
and list2
further in the program, you can assign the concatenated list to any of them as given below. This will help you reduce memory usage of the program.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
list1 = list1 + list2
print("The concatenated list is:", list1)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Using the +
operator to concatenate two lists has the advantage of concatenating more than two lists using a single statement. For instance, we can concatenate four lists in Python using the +
operator as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
list3 = [11, 12]
list4 = [13, 14, 15]
print("First list is:", list1)
print("Second list is:", list2)
print("Third list is:", list3)
print("Fourth list is:", list4)
newList = list1 + list2 + list3 + list4
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
Third list is: [11, 12]
Fourth list is: [13, 14, 15]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
Concatenate Lists Using the extend()
Method in Python
We can also use the extend()
method to concatenate two lists in Python. When executed on a list, the extend()
method accepts any iterable object like list, set, or tuple and adds the elements of the iterable object to the end of the list.
To concatenate two lists, we can execute the extend()
method on the first list and pass the second list as an input argument to the extend()
method as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
list1.extend(list2)
print("The concatenated list is:", list1)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Here, we have appended the elements of list2
to list1
using the extend()
method.
Concatenate Lists Using the +=
Operator in Python
The +=
operator is an unpopular but efficient tool to concatenate two lists in Python. It works similarly to the extend()
method. The syntax for using the +=
operator is as follows.
oldList += iterable
Here,
oldList
is the existing list that we already have.iterable
is any iterable object like list, tuple, or set. The elements in theiterable
are added to theoldList
when we execute this statement.
To concatenate two lists using the +=
operator, we will use the first list as the oldList
and the second list as the iterable
as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
list1 += list2
print("The concatenated list is:", list1)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In the output, you can observe that we have obtained the concatenated list in list1
.
Concatenate Lists Using Packing and Unpacking in Python
In Python, packing and unpacking are often used to convert a container object to another container object having the same elements. For unpacking, we use the *
operator before the name of the container object as *container_object
. We will put the unpacked elements inside brackets []
to pack the elements again in a container object like a list.
To concatenate two or more lists using the packing and unpacking operations, we will first unpack the lists using the *
operator. Then, we will pack the unpacked elements of the lists into a new list as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
newList = [*list1, *list2]
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Here, you can pack the elements of any number of lists into a single list. Thus, this method is also useful for concatenating more than two lists. For instance, you can concatenate four lists in a single statement as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
list3 = [11, 12]
list4 = [13, 14, 15]
print("First list is:", list1)
print("Second list is:", list2)
print("Third list is:", list3)
print("Fourth list is:", list4)
newList = [*list1, *list2, *list3, *list4]
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
Third list is: [11, 12]
Fourth list is: [13, 14, 15]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
Concatenate Lists Using the itertools.chain(
) Function in Python
In Python, we use the chain()
function from the itertools
module to create iterators. The chain()
function, when executed, accepts multiple iterable objects as its input arguments, chains all the elements in a single iterator, and returns the iterator. To use the chain()
function, you can import the itertools
module as follows.
import itertools
To concatenate two or more lists using the chain()
function, we will pass the original lists as input arguments to the chain()
function. After execution, the function will return an iterator
. We will use the list()
constructor to convert the iterator to a list. Hence, we will get the concatenated list. You can observe this in the following example.
import itertools
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
iterator = itertools.chain(list1, list2)
newList = list(iterator)
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Concatenate Lists Using List Comprehension in Python
On some occasions, list comprehension is a better alternative to for loop while working with lists in Python. We can also concatenate two or more lists using list comprehension. The syntax for the same is as follows.
newList= [element for temp_list in [list1, list2] for element in temp_list]
Here,
list1
andlist2
are the lists that we want to concatenate.newList
is the list created by concatenatinglist1
andlist2
.
Here, we choose each list that we have to concatenate in the temp_list
one by one. After that, we put all the elements of temp_list
in newList
.The temp_list
chooses each list that we need to concatenate one by one, and their elements are added to newList
, creating the output list with all the elements from the input lists.
We can use list comprehension to concatenate two lists in Python as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
newList = [element for temp_list in [list1, list2] for element in temp_list]
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
If you are not able to understand the syntax of the list comprehension above, the for loop in the following program is equivalent to the list comprehension syntax.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
print("First list is:", list1)
print("Second list is:", list2)
newList = list()
lists = [list1, list2]
for temp_list in lists:
for element in temp_list:
newList.append(element)
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
We can also concatenate more than two lists using list comprehension. We will just have to insert the input lists in the list of lists as follows.
list1 = [1, 2, 3, 4, 5, 6, 7]
list2 = [8, 9, 10]
list3 = [11, 12]
list4 = [13, 14, 15]
print("First list is:", list1)
print("Second list is:", list2)
print("Third list is:", list3)
print("Fourth list is:", list4)
newList = [
element for temp_list in [list1, list2, list3, list4] for element in temp_list
]
print("The concatenated list is:", newList)
Output:
First list is: [1, 2, 3, 4, 5, 6, 7]
Second list is: [8, 9, 10]
Third list is: [11, 12]
Fourth list is: [13, 14, 15]
The concatenated list is: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
What Method Should You Use to Concatenate Lists in Python?
In this article, we have discussed seven ways to concatenate lists in Python.
- If you need to concatenate two lists, you can use the
append()
method, the extend() method, or the+= operator
. On the other hand, if you want to concatenate more than two lists, you can use the + operator, theitertools.chain()
function, list comprehension, or the packing and unpacking approach. - If you want to keep the original lists unchanged while creating a new list containing elements from all the original lists, you should use only the + operator approach, the
itertools.chain()
function, the list comprehension approach, and the packing and unpacking approach. In all other approaches, we have modified one of the original lists. - If you also focus on the time complexity and space complexity of the programs, you can use the + operator, += operator, the
extend()
method, or the packing and unpacking approach for writing efficient programs. All other approaches are costly either in terms of time or memory compared to these approaches. The list comprehension approach to concatenate two lists is costliest in terms of both memory and time. So, you should try to avoid this approach.
Conclusion
In this article, we have discussed seven approaches to concatenate two or more lists in Python. We have also discussed what approach you can use based on your requirements to write an efficient program.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHubRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python