How to Get Last Element of List in Python
-
Get the Last Element of a List With
pop()
Function in Python -
Get the Last Element of a List With
-1
Index in Python - Get the Last Element of a List using a Reversing List
- Get the Last Element of a List Using the Slicing Method in Python
-
Get the Last Element of a List Using the
itemgetter()
Method in Python -
Get the Last Element of a List Using the
len()
Function in Python
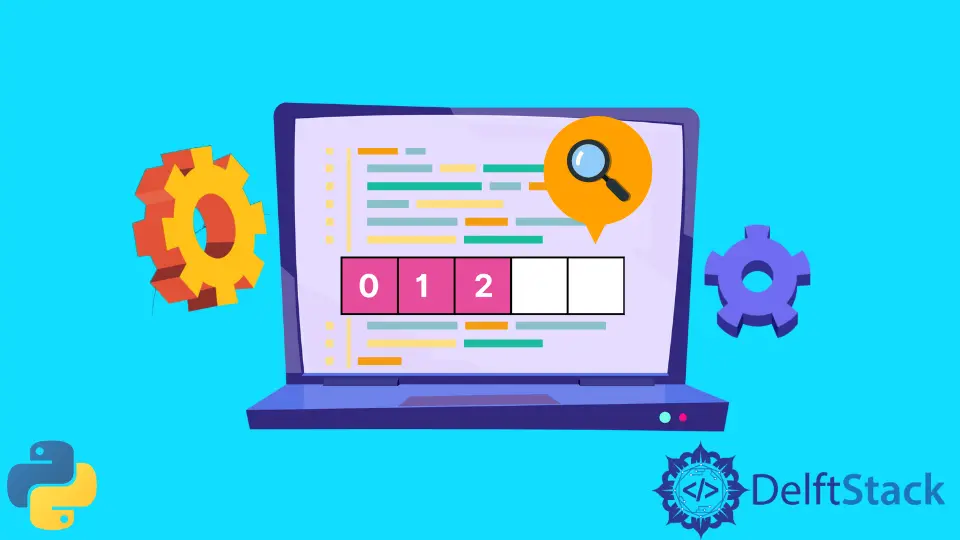
In this tutorial, we will discuss methods to get the last element of a list in Python.
Get the Last Element of a List With pop()
Function in Python
The pop()
function can be used to get the last element of a list. The pop()
function by default returns the last element of the list and removes that element from the list. The following code example shows us how we can get the last element of a list with the pop()
function in Python.
list1 = [0, 1, 2, 3, 4]
last = list1.pop()
print(last)
print(list1)
Output:
4
[0, 1, 2, 3]
In the above code, we first initialize a list list1
and then get the last element of the list1
with the pop()
function.
Get the Last Element of a List With -1
Index in Python
If we do not want to remove the last element from the list, we have to use -1
as the list index. In Python, the -1
index means the last index. Similarly, the -2
index indicates the second last index and so on. The following code example shows us how we can get the last element of a list with the -1
index in Python.
list1 = [0, 1, 2, 3, 4]
print(list1[-1])
print(list1)
Output:
4
[0, 1, 2, 3, 4]
In the above code, we first initialize a list list1
and then get the last element of the list1
with the -1
index.
Both methods are doing the same thing, and the only difference is that the pop()
function deletes the last element while the -1
index does not.
Get the Last Element of a List using a Reversing List
Reversing the list is also a method to find the last element of the list. First of all, the list is reversed using python’s built-in method reverse()
, and then the first index element of the reversed list is the last element of the original list, as shown below.
Code:
# python
lang_list = ["python", "CSS", "java", "C++", "Groovy"]
lang_list.reverse()
print(" last element is :", lang_list[0])
Output:
Using the reverse()
method, we can reverse any list, and with the help of which the last element will be now at the start, we can use the indexing to get the last element that will be now the first element of the list.
Get the Last Element of a List Using the Slicing Method in Python
Slicing is another method of finding the last element of any list. It can be used in both conditions when we know the length of the list or when we don’t know the length of the list.
# python
lang_list = ["python", "CSS", "java", "C++", "Groovy"]
print(lang_list[-1:][0])
Output:
We got the last element from the list using the slicing method.
Get the Last Element of a List Using the itemgetter()
Method in Python
Itemgetter
methods can get the last element from the list. Itemgetter
creates a callable that takes an iterable object (e.g., list, tuple, set) as input and returns the element you asked for.
Let’s have an example and use this method to get the last element from the list. First, we have to import the operator
module, and then we can use the itemgetter
function to get the list’s last element, as shown.
Code:
# python
import operator
lang_list = ["python", "CSS", "java", "C++", "Groovy"]
last_element = operator.itemgetter(-1)(lang_list)
print("last element is:", last_element)
Output:
After importing the operator
module, we can easily manipulate the list using the itemgetter
method and easily get the last element from the list.
Get the Last Element of a List Using the len()
Function in Python
len()
is a function used to find the length of the list, and by using this function, we can also find the last element of the list.
Code:
# python
lang_list = ["python", "CSS", "java", "C++", "Groovy"]
last_element = lang_list[len(lang_list) - 1]
print("last element is: ", last_element)
Output:
Many methods and built-in functions can be used to get the list’s last element. These functions can also be used in different conditions, and there can be many situations in which these functions and methods can be used to make your daily life easier.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python