How to Get Hour and Minutes From Datetime in Python
-
Get Hour and Minute From
datetime
in Python Using thedatetime.hour
anddatetime.minute
Attributes -
Get Hour and Minute From Current Time in Python Using
datetime.now()
andstrftime()
-
Get Hour and Minute From Current Time in Python Using
datetime.now()
andtime()
- Conclusion
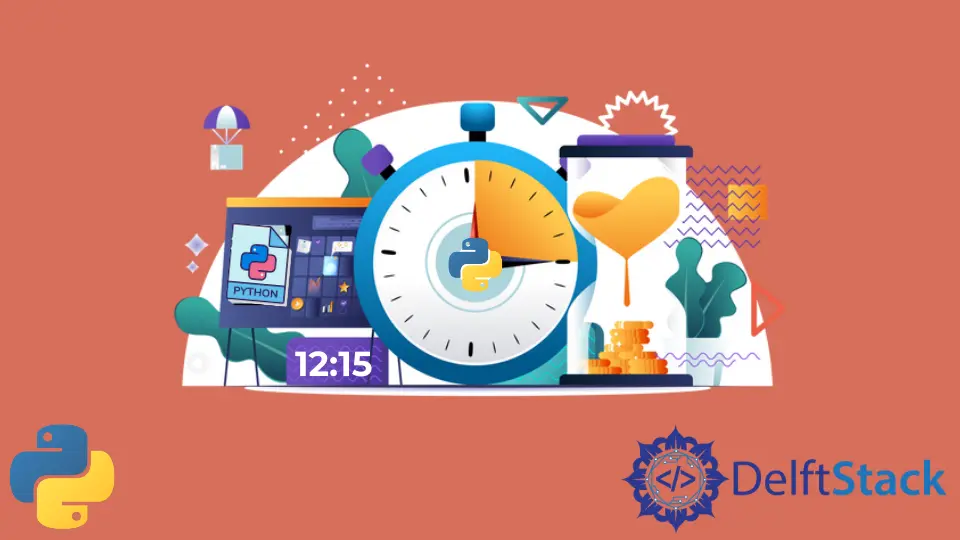
Understanding how to extract specific components, such as hour and minute, from date and time information is crucial in various Python applications.
The datetime
module provides a comprehensive set of tools, and in this article, we’ll delve into two methods: using datetime.hour
and datetime.minute
attributes to extract from a specific time and employing datetime.now()
with strftime()
and time()
to obtain hour and minute from the current time.
Let’s explore these techniques for effective time manipulation in Python.
Get Hour and Minute From datetime
in Python Using the datetime.hour
and datetime.minute
Attributes
The datetime
module in Python provides the datetime
class, which is instrumental for working with dates and times. This class includes various attributes and methods that allow you to manipulate and access different components of a date-time object.
To get started, import the datetime
class:
from datetime import datetime
Suppose you have a specific time in mind, such as 03/02/21 16:30
, and you want to extract the hour and minute from it. In this case, you can use the datetime.strptime()
method to create a datetime
object from a string, specifying the format of the input string.
from datetime import datetime
specific_time = datetime.strptime("03/02/21 16:30", "%d/%m/%y %H:%M")
print("Specific Time is", specific_time)
The output will be:
Specific Time is 2021-02-03 16:30:00
This specific_time
object now represents the date and time 03/02/21 16:30
.
Once you have the datetime
object, extracting the hour and minute is straightforward. Use the .hour
and .minute
attributes, respectively:
from datetime import datetime
specific_time = datetime.strptime("03/02/21 16:30", "%d/%m/%y %H:%M")
specific_hour = specific_time.hour
specific_minute = specific_time.minute
print("Specific Time is", f"{specific_hour:02d}:{specific_minute:02d}")
In this example, the f"{specific_hour:02d}"
and f"{specific_minute:02d}"
ensure that the hour and minute are formatted with leading zeros if needed.
The output will be:
Specific Time is 16:30
By running this script, you’ll obtain the hour and minute from the specified time in the HH:MM
format.
Get Hour and Minute From Current Time in Python Using datetime.now()
and strftime()
The datetime.now()
method is part of the datetime
module and allows us to obtain the current date and time. It returns a datetime
object representing the current date and time based on the system’s clock.
Here’s a basic example of using datetime.now()
:
from datetime import datetime
current_time = datetime.now()
print("Current Time is", current_time)
This will output something like:
Current Time is 2023-11-10 15:30:00.123456
While the default output of datetime.now()
is informative, it often contains more information than we need. To extract only the hour and minute, we can use the strftime()
method.
The strftime()
method allows us to format a datetime
object as a string based on a format string. In this case, we’re interested in formatting the time, so we use format codes such as %H
for the hour (00
-23
) and %M
for the minute (00
-59
).
Let’s see how we can use strftime()
to get the current time as hour and minute:
from datetime import datetime
current_time = datetime.now()
formatted_time = current_time.strftime("%H:%M")
print("Current Time is", formatted_time)
When you run this script, you’ll see output similar to:
Current Time is 15:30
In the example above, %H
represents the hour in 24-hour format, and %M
represents the minute. The resulting formatted_time
variable will contain a string like 15:30
representing the current hour and minute.
Feel free to customize the format string passed to strftime()
according to your specific needs. For example, you can include additional information such as seconds or am/pm indicators.
Get Hour and Minute From Current Time in Python Using datetime.now()
and time()
The datetime
module also provides a time()
method that allows us to create a time
object representing the current time. By calling this method, you get a time
object that contains attributes such as hour
, minute
, second
, and microsecond
.
Here’s an example of how to use the time()
method to obtain the current time:
from datetime import datetime
current_time = datetime.now().time()
print("Current Time is", current_time)
The output will be something like:
Current Time is 15:30:00.123456
Now that we have the current time as a time
object, extracting the hour and minute is straightforward.
from datetime import datetime
current_time = datetime.now().time()
current_hour = current_time.hour
current_minute = current_time.minute
print("Current Time is", f"{current_hour:02d}:{current_minute:02d}")
In this example, datetime.now()
retrieves the current date and time as a datetime
object. We then use .time()
to extract the time component, and finally, we access the hour
and minute
attributes to obtain the desired information.
Feel free to customize the format of the output based on your requirements. The f"{current_hour:02d}"
and f"{current_minute:02d}"
ensure that the hour and minute are formatted with leading zeros if needed.
By running this script, you’ll obtain the current hour and minute in the HH:MM
format.
Current Time is 15:30
Conclusion
In conclusion, the Python datetime
module offers an array of methods and attributes that simplify the extraction of hour and minute components from time data. Whether parsing a specific time using datetime.strptime()
or working with the current time using datetime.now()
and time()
, these techniques allow us to manipulate time information efficiently.
By leveraging the versatility of the datetime
module, we can streamline tasks related to time management and enhance the user experience in applications.