Float vs Int in Python
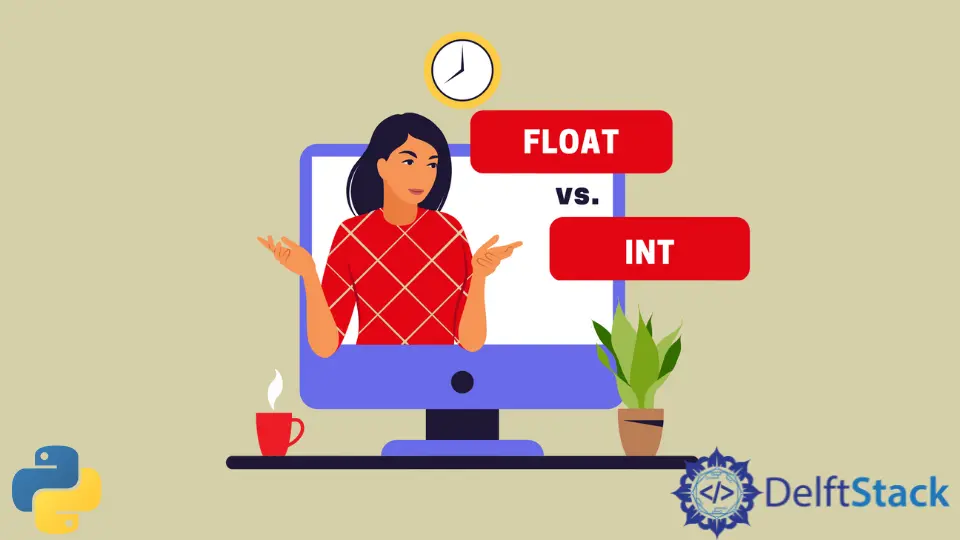
Data types represent the type of data that determines what kind of operations we can perform on the data. We have a lot of data types in Python Programming, such as int, float, List, Tuple, and many more.
However, this article will majorly cover the integer and float types.
Float vs Integer Data Type in Python
A variable holds data, and every data or value has a type associated with it. Since Python is a dynamically typed language, whenever it takes input from the user, it takes everything as a string data type: a sequence of characters.
But it is not always the case for taking a string as an input. We may require an integer, a floating-point number, also.
In those cases, we need to explicitly convert the input from the user’s end to an integer or float type, whichever we need. However, the compiler will only interpret it as a string unless we convert the input to other data types.
Numeric Data Types in Python
Numeric data types store only numbers. The integer, floating, and complex values come under the category of Numeric data types.
Python’s int, float, and complex classes define the data types. Both integer and floating numbers are immutable data types which means changing the value of these numeric data types results in creating a newly allocated object.
In Python Programming, we use the type()
function to determine the type of a particular variable.
Integer Data Type in Python
An integer is a number that does not have any fractional parts that can be positive, negative, or zero. We can say that integers are whole numbers that do not have a fractional part and have unlimited precision.
Integers can be represented in binary, octal, and hexadecimal format. All these integer variables are an object of the integer class.
Using the type()
function, we can check the type of any variable, like in the following example.
a = 10
type(a)
Output:
int
We must explicitly convert the type while taking input from the user, for which we utilize the int()
function to transform the input to an integer.
The code’s output below shows that the variable b
type is str
, a string, but the value kept in it is an integer.
print("Enter the value of b")
b = input()
print(b)
print(type(b))
Output:
-6
-6
<class 'str'>
In this next example, we have converted the input to integer type using the int()
function.
print("Enter the value of b")
b = int(input())
print(b)
print(type(b))
Output:
Enter the value of b
10
10
<class 'int'>
The need for converting these values to their actual data types comes in whenever we have to perform certain arithmetic operations on them.
print("Enter the value of a")
a = input()
print("Enter the value of b")
b = input()
print(a + b)
print(type(a + b))
Output:
Enter the value of a
2
Enter the value of b
3
23
<class 'str'>
In the above example, the two values that have been taken as input are not converted to an int
type. Therefore, the values 2
and 3
are concatenated instead of adding up since the string types use the +
operator to concatenate two strings.
print("Enter the value of a")
a = int(input())
print("Enter the value of b")
b = int(input())
print(a + b)
print(type(a + b))
Output:
Enter the value of a
2
Enter the value of b
3
5
<class 'int'>
In this last example, after taking a
and b
as input from the user and converting both of the values 2
and 3
to the int type, they are properly added and give proper desired results.
Float Data Type in Python
Float data types in Python represent real numbers with a decimal or a fractional part. The numbers with a decimal point are divided into an integer and a fractional part, making them a floating-point number when combined.
In python, floating-point values are represented using 64-bit double-precision values. We can also represent a floating-point number in a scientific notation using the letter e
or E
, and the number written after e
will represent the power of 10
.
We use the float()
function to convert a number to its float representation. The same problems that we discussed in the integer data type while taking input from the user go here as well.
print("Enter the value in decimal: ")
a = input()
print(a)
print(type(a))
Output:
Enter the value in decimal:
2.4
2.4
<class 'str'>
In the above example, when we are taking our input from the user and the user inputs a floating value, we can see that the type of this floating value is still a string.
This mismatch of data types causes irregularities in the arithmetic operations. For example, adding two input values that are not converted to float types will give the concatenation of the two values instead of the addition operation that we wanted.
Therefore, we convert the input to the float data type to avoid these irregularities. Let us see the example to convert the value to the float type using the float()
function.
print("Enter the value in decimal: ")
a = float(input())
print(a)
print(type(a))
Output:
Enter the value in decimal:
2.4
2.4
<class 'float'>
Now let us perform some arithmetic operations on the float data types. In this next example, we try to subtract the two numbers.
However, the user’s two input numbers are not explicitly converted to the float type. Therefore, operation a-b
gives an error stating that the -
operation can not be performed on string variables.
print("Enter the value of a")
a = input()
print("Enter the value of b")
b = input()
print(a - b)
print(type(a - b))
Output:
Enter the value of a
2
Enter the value of b
4
Traceback (most recent call last):
File "D:\ex1.py", line 5, in <module>
print(a-b)
TypeError: unsupported operand type(s) for -: 'str' and 'str'
The arithmetic operations on the floating values can also be performed easily and correctly after converting the input to float type.
print("Enter the value of a")
a = float(input())
print("Enter the value of b")
b = float(input())
print(a - b)
print(type(a - b))
Output:
Enter the value of a
4
Enter the value of b
6
-2.0
<class 'float'>
Many people have a problem using float and when to use it in a program. If we think that a variable will contain a decimal point value, we convert them to float. Otherwise, convert it into an integer.
principal = int(input())
rate = float(input())
time = int(input())
interest = principal * rate * time
print(interest)
Output:
1200
2.5
2
6000.0
In the above code, we calculate the simple interest, and as we know, principal amount and time are integers, whereas the interest rate can also be a decimal number. Therefore we convert it into float type.
However, the simple interest stored in the interest variable is float data type because basic arithmetic operations convert integer to float implicitly by default if one of its operands has a float type.
Float vs Int in Python
It would be best if you used int data type in Python, where we need to store the count of objects in the variables and use float data types in variables where we perform operations on decimal numbers. Similarly, it would help if you used variables with float data types in programs where you need to perform scientific calculations.
Conclusion
In this article, we studied data types numeric data types and had a detailed discussion about integer and float data types. We have also discussed many examples to show the working of integer and float data types in Python.