How to Check if Variable Is String in Python
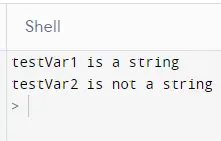
We will introduce two different methods to check whether a variable is a string or not in Python with Examples.
Check if Variable Is String in Python
In Python, every variable has a data type. Data type represents what kind of data a variable is storing inside.
Data types are the most important feature of programming languages to distinguish between different types of data we can store, such as string, int, and float.
While working on many programming problems, there may be some situations in which we may come across a problem in which we need to find the data type of a certain variable to perform some tasks on it.
Python provides us with two functions, isinstance()
and type()
, used to get the data type of any variables. If we want to ensure that a variable stores a particular data type, we can use the isinstance()
function.
Let’s go through an example in which we will create two variables, one with the data type string and another with the data type of int. We will test both variables and check whether the isinstance()
function can detect the data types or not.
Code Example:
# python
testVar1 = "This is a string"
testVar2 = 13
if isinstance(testVar1, str):
print("testVar1 is a string")
else:
print("testVar1 is not a string")
if isinstance(testVar2, str):
print("testVar2 is a string")
else:
print("testVar2 is not a string")
Output:
As you see from the output, the function can accurately detect any variable’s data type.
Try the same scenario with the second function, type()
.
Code Example:
# python
testVar1 = "This is a string"
testVar2 = 13
if type(testVar1) == str:
print("testVar1 is a string")
else:
print("testVar1 is not a string")
if type(testVar2) == str:
print("testVar2 is a string")
else:
print("testVar2 is not a string")
Output:
We can use the type()
to detect the data type of any variable and execute functions accordingly.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn