How to Find Index of Minimum Element in a List in Python
-
Python Find Index of Minimum in List Using the
min()
andindex()
Functions -
Use the
min()
Function andfor
Loop to Find the Index of Minimum in the List in Python -
Python Find Index of Minimum in List Using the
min()
andenumerate()
Functions -
Use
min()
andoperator.itemgetter()
to Find Index of the Minimum Element in a List in Python -
Use the
min()
and__getitem__()
Functions to Find the Index of the Minimum Element in a List in Python -
Use the
numpy.argmin()
Function to Find the Index of the Minimum Element in a List in Python - Conclusion
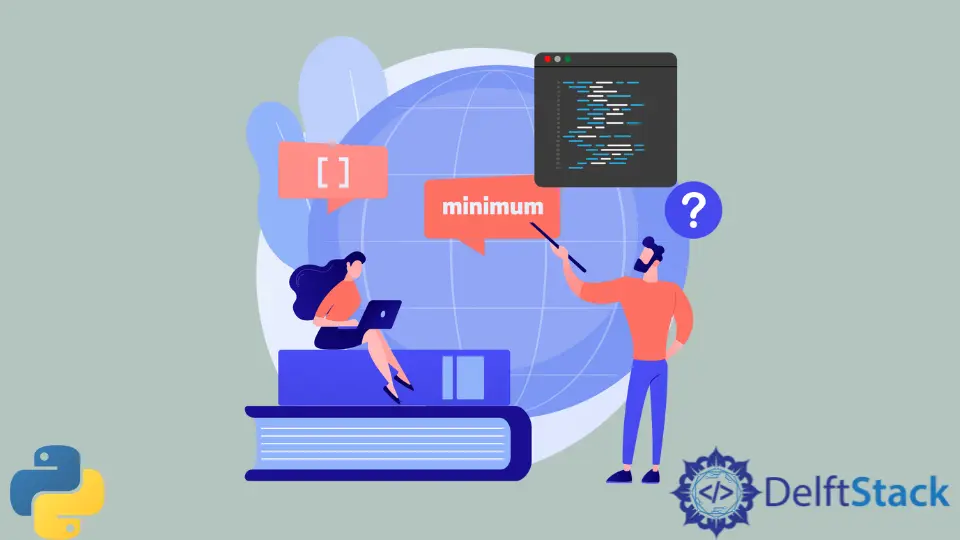
Finding the index of the minimum element in a list is a common task in programming, especially when dealing with data analysis or algorithmic problem-solving. In Python, there are several methods to achieve this goal, each with its advantages and use cases.
A list object in Python emulates an array and stores different elements under a common name. Elements are stored at a particular index, which we can use to access them.
We can perform different operations with a list. It is straightforward to use built-in list functions like max()
, min()
, len
, and more to return the maximum element, smallest element, and list length.
This article will discuss various methods to find the index of the minimum element in a list, providing detailed explanations and example codes for each approach.
Python Find Index of Minimum in List Using the min()
and index()
Functions
The min()
function in Python is a versatile built-in function that returns the smallest item in an iterable or the smallest of 2 or more arguments. Its usage extends beyond finding the minimum value; it can also be employed to find the minimum element in a list.
The index()
function is another valuable built-in function in Python, specifically designed for finding the index of the first occurrence of a specified value in a sequence. This function complements min()
perfectly when our goal is to locate the index of the minimum element.
ValueError
is raised if the given element is not in the list.Now, let’s explore how the combination of these two functions simplifies the process of finding the index of the minimum element in a list.
lst = [8, 6, 9, -1, 2, 0]
m = min(lst)
print(lst.index(m))
Output:
3
The min()
function identifies the minimum element in the list, and then the index()
function is applied to find its index. This concise approach eliminates the need for separate variables and streamlines the code.
Remember, the index of a list starts from 0. The above answer shows 3 since the smallest element is in the fourth position.
Use the min()
Function and for
Loop to Find the Index of Minimum in the List in Python
We can substitute the use of the index()
function in the previous method with a for
loop. It can iterate over the list and compare elements individually.
When there is a match, we return the value of that index and break out of the loop using the break
statement.
Example:
lst = [8, 6, 9, -1, 2, 0]
m = min(lst)
for i in range(len(lst)):
if lst[i] == m:
print(i)
break
Output:
3
The code initializes a list lst
and finds its minimum element using the min()
function.
It then iterates through the list, checking for the first occurrence of the minimum element. Once found, it prints the index of that occurrence and breaks out of the loop.
The code is designed to efficiently identify and print the index of the first instance of the minimum element in the given list, in this case, [8, 6, 9, -1, 2, 0]
, where the minimum element is -1
, and its index is 3
.
Python Find Index of Minimum in List Using the min()
and enumerate()
Functions
The enumerate()
function in Python is a valuable tool when iterating over elements of a list while simultaneously keeping track of their indices. This is particularly useful when the goal is to find the index of a specific element, such as the minimum element in a list.
The enumerate()
function accepts an iterable. It returns an object containing the elements of the iterable with a counter variable for the element index.
This object can be iterated using a for
loop. Then, we will iterate over this object using list comprehension, create a new list, and use the min()
function to locate the minimum element in a list.
We will get the element and its index in one line.
lst = [8, 6, 9, -1, 2, 0]
a, i = min((a, i) for (i, a) in enumerate(lst))
print(i)
Output:
3
We can also use these methods with a custom lambda function to find the index of the minimum element in a list in Python.
# Example 2: Using enumerate()
numbers = [4, 2, 7, 1, 9, 3]
min_index = min(enumerate(numbers), key=lambda x: x[1])[0]
print("Index of minimum element:", min_index)
Output:
Index of minimum element: 3
The enumerate(numbers)
returns pairs of index and value. The min()
function is then used with a custom lambda function to find the minimum element based on values, and [0]
extracts the index.
Use min()
and operator.itemgetter()
to Find Index of the Minimum Element in a List in Python
The operator
module in Python provides additional operators which we can use to simplify our code. The itemgetter()
function from this module returns a callable object and can retrieve some element from its operand.
The min()
function accepts a key
parameter to determine the criteria for the comparison. We can provide the itemgetter()
function as the value for this parameter to return the index of the minimum element.
Example:
from operator import itemgetter
lst = [8, 6, 9, -1, 2, 0]
i = min(enumerate(lst), key=itemgetter(1))[0]
print(i)
Output:
3
We first find the minimum element and its index in the previous methods. This method does both these steps in one line; therefore, it is considered a faster approach.
Use the min()
and __getitem__()
Functions to Find the Index of the Minimum Element in a List in Python
The operator.itemgetter()
function calls the magic function __getitem__()
internally. We can avoid the need for importing the operator
module by directly working with this function and improving the speed of the code.
It is similar to the previous method to return the minimum element index in a list in Python.
Example:
lst = [8, 6, 9, -1, 2, 0]
i = min(range(len(lst)), key=lst.__getitem__)
print(i)
Output:
3
This code efficiently finds the index of the minimum element in the given list lst
. It uses the min()
function with a custom key argument to determine the minimum based on the values in the list.
The range(len(lst))
creates an iterable of indices, and the key=lst.__getitem__
specifies that the comparison should be based on the values in the list. The result, assigned to the variable i
, is the index of the minimum element.
Finally, the code prints the value of i
. In the context of the provided list [8, 6, 9, -1, 2, 0]
, the minimum element is -1
, and the code will output the index 3
.
Use the numpy.argmin()
Function to Find the Index of the Minimum Element in a List in Python
The numpy.argmin()
function is used to find the position of the smallest element in Python. We can use this function with lists, and it will return an array with the indices of the minimum element of the list.
Example:
import numpy as np
lst = [8, 6, 9, -1, 2, 0]
i = np.argmin(lst)
print(i)
Output:
3
This Python code utilizes the numpy
library to find the index of the minimum element in a given list lst
. The code uses np.argmin(lst)
to find the index of the minimum element in the list using the NumPy function argmin()
. Finally, the obtained index is printed to the console.
This code essentially provides a concise way to find and display the index of the minimum element in the specified list using the capabilities of the NumPy library.
Conclusion
This article explores various methods for finding the index of the minimum element in a Python list. The central approach involves using the min()
function to identify the minimum element and the index()
function to obtain its index.
Alternative methods are also included, such as combining min()
with enumerate()
, leveraging the numpy
library, or using the operator.itemgetter()
with the__getitem__()
function.
Each method is detailed with example codes, providing developers with a diverse toolkit to choose from based on their specific requirements. The article emphasizes factors like simplicity, performance, and readability, ensuring that programmers can make informed decisions when tackling this common programming task.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python