How to Decrement a Loop in Python
- Understanding the Basics of Loops
- Using a Decrementing For Loop
-
Implementing a Decrementing
while
Loop - Decrementing a Loop with Custom Steps
- Combining Decrementing Loops with Conditions
- Conclusion
- FAQ
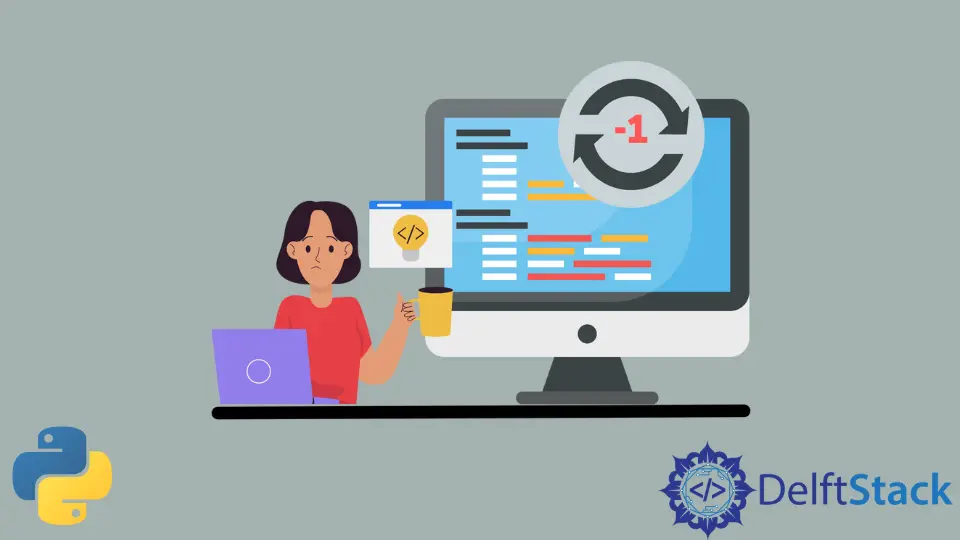
In the world of programming, loops are a fundamental concept that allows us to execute a block of code multiple times. While most tutorials focus on incrementing loops, decrementing a loop can be just as crucial, especially when you need to count down or reverse through a sequence.
In this tutorial, we’ll explore how to effectively decrement a loop in Python, providing you with clear examples and explanations. Whether you’re a beginner looking to grasp the basics or an experienced coder wanting to refine your skills, this guide will help you understand the nuances of decrementing loops in Python. Let’s dive in!
Understanding the Basics of Loops
Before we delve into decrementing loops, it’s essential to understand what loops are and how they function in Python. A loop allows you to execute a block of code repeatedly until a specified condition is met. In Python, the most common types of loops are for
loops and while
loops.
A decrementing loop is one that counts down from a specified number to a lower limit. This is particularly useful in scenarios where you need to reverse through a list or perform actions in a countdown format. Let’s look at how to implement this with both for
and while
loops.
Using a Decrementing For Loop
The for
loop in Python is a versatile tool that can also be used for decrementing. We can utilize the built-in range()
function to create a countdown. Here’s how it works:
for i in range(10, 0, -1):
print(i)
Output:
10
9
8
7
6
5
4
3
2
1
In this example, the range(10, 0, -1)
function generates a sequence starting from 10 down to 1, decrementing by 1 each time. The first argument is the starting point, the second argument is the stopping point (not inclusive), and the third argument is the step, which is negative in this case. The loop then prints each number in the countdown, making it a straightforward and efficient way to create a decrementing loop.
Implementing a Decrementing while
Loop
Another method to decrement a loop is by using a while
loop. This approach gives you more control over the loop’s exit condition, allowing for more complex scenarios. Here’s an example:
count = 10
while count > 0:
print(count)
count -= 1
Output:
10
9
8
7
6
5
4
3
2
1
In this snippet, we start with a variable count
initialized to 10. The while
loop continues to execute as long as count
is greater than 0. Inside the loop, we print the current value of count
and then decrement it by 1 using count -= 1
. This method is particularly useful when the decrementing condition is more complex or when you need to determine the loop’s exit dynamically.
Decrementing a Loop with Custom Steps
Sometimes, you may want to decrement by a value other than 1. This can be done easily with both for
and while
loops. Here’s how to do it using a for
loop:
for i in range(20, 0, -2):
print(i)
Output:
20
18
16
14
12
10
8
6
4
2
In this example, range(20, 0, -2)
creates a sequence that starts at 20 and decrements by 2 until it reaches 0. This allows for more flexibility in your decrementing loops, enabling you to customize the step size according to your needs.
Combining Decrementing Loops with Conditions
You can also integrate conditions within your decrementing loops to add more functionality. For instance, you might want to skip certain numbers or perform different actions based on the current value of the loop variable. Here’s an example using a while
loop:
count = 10
while count > 0:
if count % 2 == 0:
print(f"{count} is even")
else:
print(f"{count} is odd")
count -= 1
Output:
10 is even
9 is odd
8 is even
7 is odd
6 is even
5 is odd
4 is even
3 is odd
2 is even
1 is odd
In this code, we check if the current count
is even or odd. Depending on the condition, we print a corresponding message. This showcases how decrementing loops can be combined with conditional statements to perform more complex operations.
Conclusion
Decrementing loops in Python are a powerful tool for controlling the flow of your programs. Whether you use a for
loop or a while
loop, understanding how to decrement allows you to implement countdowns, reverse iterations, and more complex logic. With the examples provided, you should now feel confident in your ability to create decrementing loops tailored to your specific needs. Keep practicing, and soon you’ll be able to leverage this technique in various programming scenarios.
FAQ
-
What is the difference between a for loop and a
while
loop in Python?
A for loop iterates over a sequence or range, while awhile
loop continues until a specified condition is no longer true. -
Can I decrement by numbers other than 1?
Yes, you can specify any step size in the range function for a for loop or adjust the decrement value in awhile
loop. -
How do I stop a loop prematurely?
You can use thebreak
statement to exit a loop before it has completed all iterations. -
What happens if I set the starting point lower than the stopping point in a decrementing loop?
The loop will not execute, as the starting point must be greater than the stopping point for a decrementing loop to function correctly. -
Are decrementing loops only used for counting down?
No, decrementing loops can be used for various purposes, including iterating through lists in reverse order or executing code until certain conditions are met.