How to Covert Text File to CSV in Python
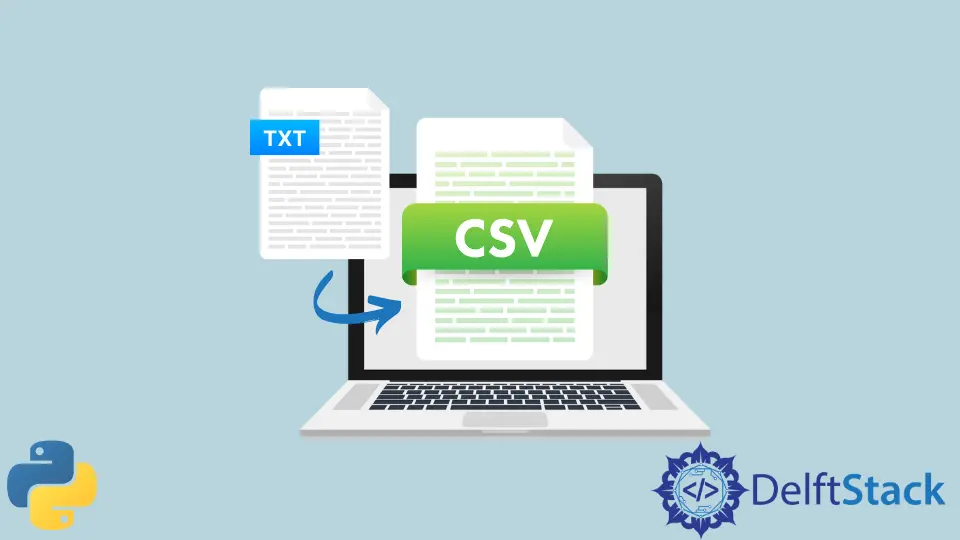
Pandas offers a range of functions, among which is the ability to convert a text file to a CSV file.
To get started, we first need to install the pandas using Anaconda. Alternatively, pandas can also be installed using the pip package manager on Windows, as shown below.
pip install pandas
Output:
Defaulting to user installation because normal site-packages is not writeable
Collecting pandas
Downloading pandas-1.3.4-cp310-cp310-win_amd64.whl (10.2 MB)
|████████████████████████████████| 10.2 MB 1.3 MB/s
Collecting numpy>=1.21.0
Downloading numpy-1.21.4-cp310-cp310-win_amd64.whl (14.0 MB)
|████████████████████████████████| 14.0 MB 90 kB/s
Collecting python-dateutil>=2.7.3
Downloading python_dateutil-2.8.2-py2.py3-none-any.whl (247 kB)
|████████████████████████████████| 247 kB 1.3 MB/s
Collecting pytz>=2017.3
Downloading pytz-2021.3-py2.py3-none-any.whl (503 kB)
|████████████████████████████████| 503 kB 1.3 MB/s
Collecting six>=1.5
Downloading six-1.16.0-py2.py3-none-any.whl (11 kB)
Installing collected packages: six, pytz, python-dateutil, numpy, pandas
WARNING: The script f2py.exe is installed in 'C:\Users\tonyloi\AppData\Roaming\Python\Python310\Scripts' which is not on PATH.
Consider adding this directory to PATH or, if you prefer to suppress this warning, use --no-warn-script-location.
Successfully installed numpy-1.21.4 pandas-1.3.4 python-dateutil-2.8.2 pytz-2021.3 six-1.16.0
Once the installation is done, we need to import the pandas package into our code using the import statement.
This then gives us access to the Pandas I/O API, which has both reader and writer functions.
Before implementing the reader function, we need to take note of the path to the file location. The path is often similar to the one below with variations depending on where the file is located in your computer.
# python 3
C:\Users\tonyloi\Desktop\sample.txt
On the other hand, we also need to determine the location that the new CSV file that will be generated will be stored, as this is one common argument passed to the writer function.
In addition, the name of the CSV file also needs to be determined beforehand. This location may be similar to the one below depending on where you want the new CSV file to be stored.
C:\Users\tonyloi\Desktop\sample.csv
Once we have all this in place, it is now the right time to write the two functions: a reader function to read the text file into our current workspace and a writer function to convert the text to CSV format.
Example Codes:
# python 3.x
import pandas as pd
file = pd.read_csv(r"C:\Users\tonyloi\Desktop\sample.txt")
new_csv_file = file.to_csv(r"C:\Users\tonyloi\Desktop\sample_csv_file.csv")
Executing the code above creates a new CSV file named sample_csv_file.csv
at the specified location.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedInRelated Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python