Class Methods in Python
-
Use the
classmethod()
Function to Create a Class Method in Python -
Use the
@classmethod
Decorator to Create a Class Method in Python
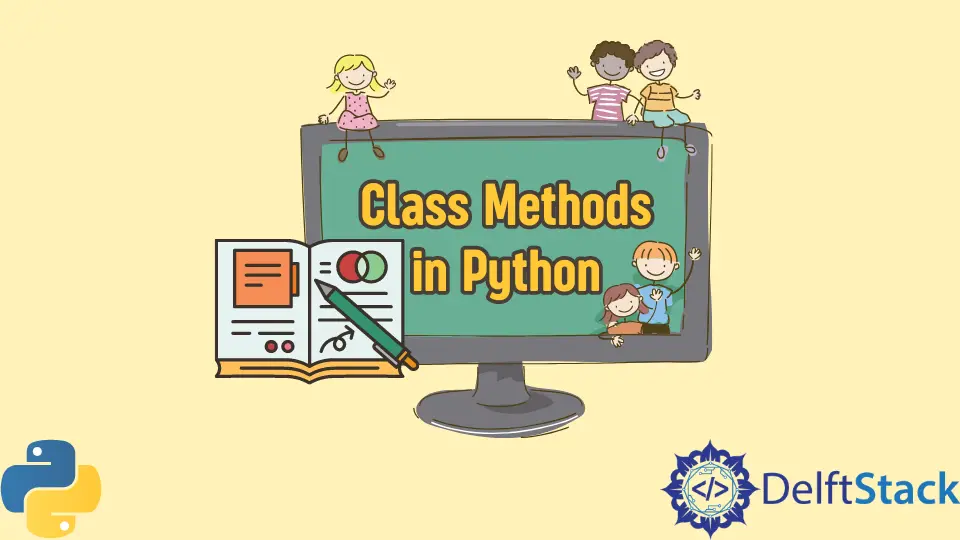
A class acts as a pre-defined structure or blueprint for creating objects.
We can provide a class with different attributes and methods in Python. Python enables us to define various methods for a class.
First, we have the instance methods that can access all the class attributes and be accessed via the class instances. We also have class methods and static methods for a class.
This tutorial will demonstrate the class methods in Python.
Class methods can be called without any instance of the class. These methods have access to the states of the class.
They can access the fields and attributes of the class and take an argument of the class itself, not an instance.
This argument holds the class object and not an instance of the given class. We can either use the inbuilt classmethod()
function or the @classmethod
decorator to create class methods in Python.
Use the classmethod()
Function to Create a Class Method in Python
The classmethod()
function accepts a function that must be converted to a class method. We can define a function in the class and then convert it to a class method.
For example:
class Emp:
dept = "python"
def dept_name(obj):
print("Department: ", obj.dept)
Emp.dept_name = classmethod(Emp.dept_name)
Emp.dept_name()
Output:
Department: python
In the above example, we converted the dept_name()
function of the class Emp
to a class method using the classmethod()
function. We access this function using the class name.
However, this method is considered outdated and un-Pythonic. The @classmethod
decorator is preferred in the recent versions of Python.
We discuss this method below.
Use the @classmethod
Decorator to Create a Class Method in Python
The @classmethod
decorator can be placed before a function to return a class method in Python. This decorator is evaluated after the function definition.
Using this method, we need to provide the cls
argument to the function as a compulsion. It shows that the class method refers to the class itself and not any instance.
We will use this method with the function in the previous example.
class Emp:
dept = "python"
@classmethod
def dept_name(obj):
print("Department: ", obj.dept)
Emp.dept_name()
Output:
Department: python
One should remember that a class method is applied to the attributes of all class instances. These methods will get inherited by a child class.
We can use class methods for implementing overloading functionalities in Python.
See the following example.
class Emp(object):
def __init__(self, name="Na", age=10):
self.name = name
self.age = age
@classmethod
def from_str(cls, sample):
name, age = sample.split(",")
e1 = cls(name, age)
return e1
e1 = Emp.from_str("Matt,15")
print(e1.name, e1.age)
Output:
Matt 15
The class method returns an Emp
class object using the cls
object in the above example. We created an object of this class by splitting a string.
A static method is similar to a class method. We can access static methods like a class method without creating class instances.
However, a static function has no access to the class state and cannot modify it. No class attribute can access this method and cannot be called by any class method.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn