How to Check List Is Empty in Python
-
Use the
if not
Statement to Check if a List Is Empty or Not -
Use the
len()
Function to Check if a List Is Empty or Not -
Use an Empty List
[]
to Check if a List Is Empty or Not in Python
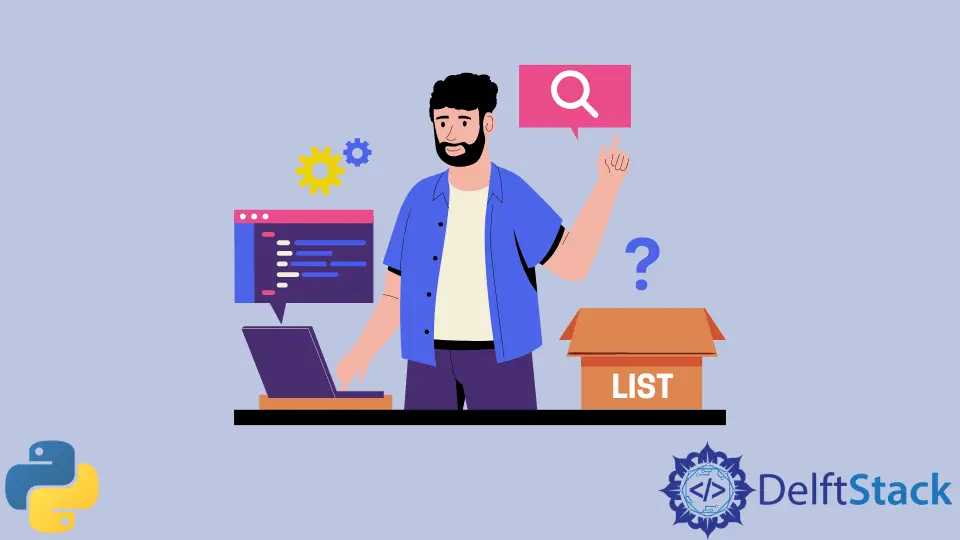
This tutorial will introduce how to check if a list is empty in Python.
Use the if not
Statement to Check if a List Is Empty or Not
In Python, if a list or some other data type is empty or NULL
then it is considered False
. The if not
statement is used to execute a block of code if a condition is False
; thus, we can use it to check if a list is empty or not. The following code will explain this.
lst = []
if not lst:
print("Empty")
else:
print("Not Empty")
Output:
Empty
Use the len()
Function to Check if a List Is Empty or Not
The len()
function in Python returns the total number of elements in a list. So if the len()
function returns 0 then the list is empty. We will implement this in the code below.
lst = []
if len(lst) == 0:
print("Empty")
else:
print("Not Empty")
Output:
Empty
Note that this method is considered a little slow but also works with a numpy array, whereas the if not
method fails with numpy arrays.
Use an Empty List []
to Check if a List Is Empty or Not in Python
This is an unconventional method and is not used very frequently, but still, it is worth knowing and provides the same result. In this method, we directly compare our list to an empty list, and if the comparison returns True
, then the list is empty. For example,
lst = []
if lst == []:
print("Empty")
else:
print("Not Empty")
Output:
Empty
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python