How to Check if a Word Exists in a String in Python
-
Use the
in
Operator to Check if a Word Exists in a String in Python -
Use the
String.find()
Method to Check if a Word Exists in a String in Python -
Use the
String.index()
Method to Check if a Word Exists in a String in Python -
Use the
search()
Method to Check if a Word Exists in a String in Python
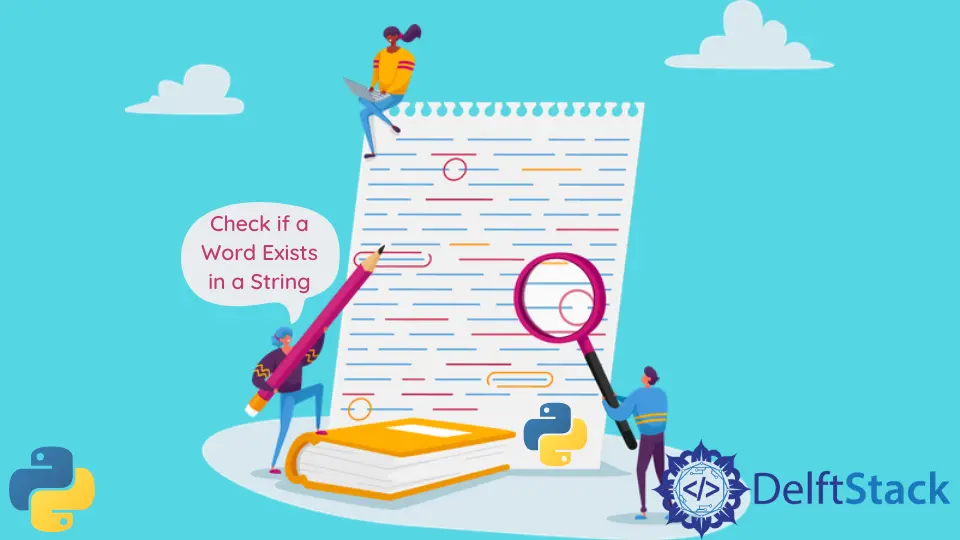
Suppose there exist a string "The weather is so pleasant today"
. If we want to check if the word "weather"
is present in the string or not, we have multiple ways to find out.
In this guide, we will look at the in
operator, string.find()
method, string.index()
method, and regular expression(RegEx)
.
Use the in
Operator to Check if a Word Exists in a String in Python
One of the easiest ways of searching a word in a string or sequences like list, tuple, or arrays is through the in
operator. It returns a boolean value when used in a condition.
It can be either true
or false
. If the specified word exists, the statement evaluates to true
; if the word does not exist, it evaluates to false
.
This operator is case-sensitive
. If we try to locate the word Fun
in the following code, we will obtain the message Fun not found
in the output.
Example code:
# Python 3.x
sentence = "Learning Python is fun"
word = "fun"
if word in sentence:
print(word, "found!")
else:
print(word, "not found!")
Output:
#Python 3.x
fun found!
If we want to check for a word within a string without worrying about the case, we must convert the main string and the word to search in the lowercase. In the following code, we will check the word Fun
.
Example Code:
# Python 3.x
sentence = "Learning Python is fun"
word = "Fun"
if word.lower() in sentence.lower():
print(word, "found!")
else:
print(word, "not found!")
Output
#Python 3.x
Fun found!
Use the String.find()
Method to Check if a Word Exists in a String in Python
We can use the find()
method with a string to check for a specific word. If the specified word exists, it will return the word’s left-most
or starting index
in the main string.
Else, it will simply return the index –1
. The find()
method also counts the index of spaces
. In the following code, we get the output 9
because 9
is the starting index of Python, the index of character P
.
This method is also case-sensitive by default. If we check for the word python
, it will return -1
.
Example Code:
# Python 3.x
string = "Learning Python is fun"
index = string.find("Python")
print(index)
Output:
#Python 3.x
9
Use the String.index()
Method to Check if a Word Exists in a String in Python
index()
is the same as the find()
method. This method also returns the lowest index of the substring in the main string.
The only difference is that when the specified word or substring does not exist, the find()
method returns the index –1, while the index()
method raises an exception (value error exception)
.
Example Code:
# Python 3.x
mystring = "Learning Python is fun"
print(mystring.index("Python"))
Output:
#Python 3.x
9
Now we try to find a word that doesn’t exist in the sentence.
# Python 3.x
mystring = "Learning Python is fun"
print(mystring.index("Java"))
Output:
#Python 3.x
ValueError Traceback (most recent call last)
<ipython-input-12-544a99b6650a> in <module>()
1 mystring = "Learning Python is fun"
----> 2 print(mystring.index("Java"))
ValueError: substring not found
Use the search()
Method to Check if a Word Exists in a String in Python
We can check for a specific word through pattern matching of strings through the search()
method. This method is available in the re
module.
The re
here stands for Regular Expression
. The search method accepts two arguments.
The first argument is the word to find, and the second one is the entire string. But this method works slower than the other ones.
Example Code:
# Python 3.x
from re import search
sentence = "Learning Python is fun"
word = "Python"
if search(word, sentence):
print(word, "found!")
else:
print(word, "not found!")
Output:
#Python 3.x
Python found!
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn