How to Break Out of Multiple Loops in Python
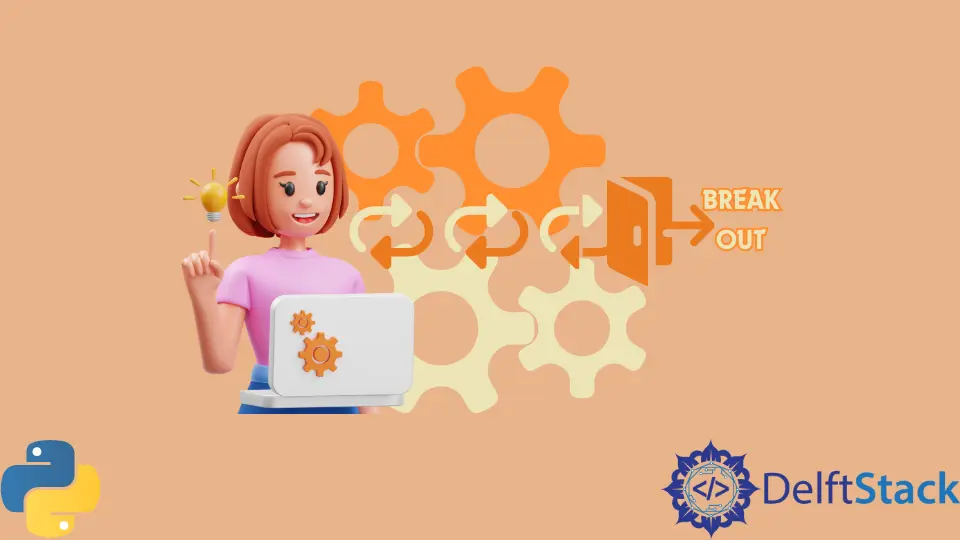
When working with nested loops in Python, you might find yourself in situations where you need to exit not just one loop, but multiple loops at once. This can be particularly challenging, especially if you’re dealing with complex logic or deeply nested structures. Fortunately, Python provides several methods to help you break out of these loops effectively.
In this article, we will explore two primary methods: using the return statement and the for/else loop. By the end of this guide, you will have a clear understanding of how to manage multiple loops in your Python code, making your programming experience smoother and more efficient.
Using the Return Statement
One of the most straightforward ways to break out of multiple loops in Python is by utilizing the return statement. This method is particularly useful when you’re working with functions. When a return statement is executed, the function exits immediately, and control returns to the calling function. This can effectively break out of all loops that are nested within that function.
Here’s a simple example to illustrate this:
def find_number(numbers, target):
for i in numbers:
for j in numbers:
if i * j == target:
return f"Found: {i} * {j} = {target}"
return "Not found"
result = find_number([1, 2, 3, 4, 5], 8)
print(result)
Output:
Found: 2 * 4 = 8
In this code, we define a function called find_number
that takes a list of numbers and a target value. The function contains two nested loops that iterate over the list. If the product of any two numbers equals the target, the function immediately returns a message indicating success. This effectively breaks out of both loops, as the return statement halts the function’s execution. If no such pair is found, the function returns “Not found.” This method is clean and efficient for managing multiple loops, especially when encapsulated within functions.
Using the For/Else Loop
Another effective way to exit multiple loops in Python is by using the for/else construct. This approach allows you to execute a block of code after a loop completes normally, which can be particularly handy when you want to perform an action if the loop didn’t encounter a break statement.
Here’s an example of how this can be implemented:
def search_pair(numbers, target):
for i in numbers:
for j in numbers:
if i + j == target:
print(f"Found: {i} + {j} = {target}")
break
else:
continue
break
else:
print("No pairs found")
search_pair([1, 2, 3, 4, 5], 7)
Output:
Found: 3 + 4 = 7
In this example, the search_pair
function checks for pairs of numbers that sum to a specified target. The outer loop iterates through each number, while the inner loop checks for a matching pair. If a pair is found, it prints the result and breaks out of the inner loop. The else
block associated with the inner loop only executes if the inner loop completes without hitting a break statement. If no pairs are found after both loops have been executed, the outer else
prints a message indicating that no pairs were found. This structure allows for clear control over loop execution and can be very useful for managing more complex logic.
Conclusion
Breaking out of multiple loops in Python can be easily accomplished using either the return statement or the for/else loop construct. Each method has its advantages and can be chosen based on the specific requirements of your code. The return statement is highly effective within functions, while the for/else loop provides a clear mechanism for handling scenarios where you want to perform actions based on loop completion. By mastering these techniques, you’ll enhance your coding skills and improve the efficiency of your Python programs.
FAQ
-
How does the return statement work in nested loops?
The return statement exits the current function immediately, effectively breaking out of all nested loops within that function. -
Can I use the for/else loop with
while
loops?
No, the for/else construct is specific to for loops.while
loops do not support this syntax. -
What happens if a break statement is used in the inner loop?
A break statement will exit only the innermost loop in which it is placed, allowing the outer loop to continue executing. -
Is there a way to break out of multiple loops without using return or for/else?
Yes, you can use flags or exceptions to control flow, but these methods are typically less clean than the return or for/else approaches. -
Are there performance differences between these methods?
Generally, there are no significant performance differences, but the choice of method can affect code readability and maintainability.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn