How to Apply a Function to a List in Python
-
Use the
for
Loop to Apply a Function to a List in Python -
Use the
map()
Function to Apply a Function to a List in Python - Use the List Comprehension Method to Apply a Function to a List in Python
- Use the Generator Expression Method to Apply a Function to a List in Python
- Conclusion
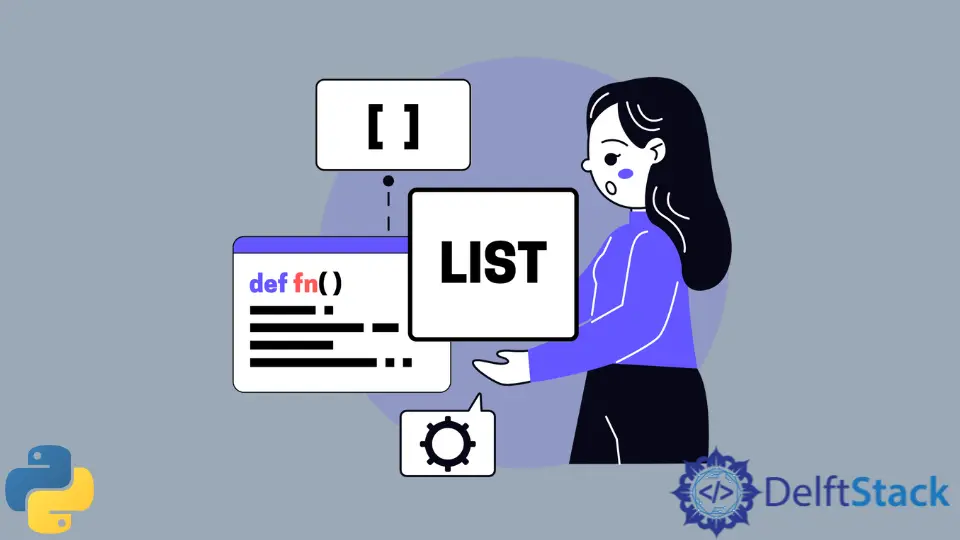
Applying a function to a list in Python involves executing a specific operation on each list element, producing a transformed or modified version of the original data. This technique is particularly useful when dealing with large datasets, allowing for concise and scalable data manipulation.
The importance of applying functions to lists cannot be overstated. It enables programmers to automate repetitive tasks, perform complex calculations, and transform data structures effortlessly.
Encapsulating operations within functions makes code modular, reusable, and easier to maintain. Furthermore, this approach promotes the concept of “Don’t Repeat Yourself” (DRY), a fundamental principle of software development.
Imagine we have a list of temperatures in Celsius and need to convert them to Fahrenheit. Instead of manually iterating through the list, applying a conversion function to each element streamlines the process.
Python’s ability to apply a function to a list allows us to accomplish this task efficiently. We obtain the desired Fahrenheit temperatures in a single line of code by writing the conversion logic within a function and applying it to the entire list.
In this tutorial, we will discuss applying a function to a list in Python.
Use the for
Loop to Apply a Function to a List in Python
In Python, the for
loop is a fundamental construct that allows us to iterate over elements in a list and perform operations on them. By leveraging the for
loop, we can seamlessly apply a function to each list element, transforming the data according to our needs.
This is the most straightforward method. We iterate through the list using the for
loop and apply the required function to each element individually.
We store the result in a separate variable and then append this variable to a new list.
In the following code, we apply a user-defined function to a list using Python’s for
loop.
def fn(a):
return 10 * a
lst = [1, 2, 3, 4]
ans = []
for i in lst:
x = fn(i)
ans.append(x)
print(ans)
Output:
The above code defines a function fn(a)
that multiplies a number by 10. It creates a list lst
with values [1, 2, 3, 4]
and an empty list ans
.
The code applies the fn()
function to each element of lst
using a for
loop, storing the results in the ans
list. Finally, it prints the contents of ans
, which will be [10, 20, 30, 40]
.
This code showcases the application of a function to each element of a list, producing a new list with the transformed values.
Use the map()
Function to Apply a Function to a List in Python
Python provides the map()
function as a powerful tool for applying a function to each element of a list, resulting in a new list with the transformed values. By utilizing map()
, we can achieve concise and efficient data manipulation.
Syntax:
map(func, iterable)
Here, func
refers to the function to be applied to each element of the iterable
(such as a list), and map()
returns an iterator that yields the results of applying the function to each element sequentially.
We can specify the function to apply and the list to operate on with a single line of code. The map()
function then iterates through the list, applying the specified function to each element, and returns an iterator object that can be converted into a list if desired.
This approach simplifies code, enhances readability, and promotes code reusability, making it an essential technique for effectively processing data in Python. In the following code, we apply a user-defined function to a list using Python’s map
function.
def fn(a):
return 10 * a
lst = [1, 2, 3, 4]
ans = list(map(fn, lst))
print(ans)
Output:
The above code applies the fn()
function to each element of lst
using the map()
function, generating an iterator of transformed values. The iterator is then converted into a list using the list()
function and stored in the variable ans
.
Finally, it prints the contents of ans
, which will be [10, 20, 30, 40]
. This code uses the map()
function to efficiently apply a function to each list element and obtain a new list with the transformed values.
Use the List Comprehension Method to Apply a Function to a List in Python
List comprehension is a powerful technique that allows us to apply a function to each element of a list and create a new list concisely and expressively. With list comprehension, we can specify the function to apply, the element transformation, and the iteration, all in a single line of code.
By combining the power of functions and iteration, list comprehension eliminates the need for explicit loops and reduces code verbosity. This method streamlines data transformation and enhances code readability, making it an essential tool for efficiently applying functions to lists in Python.
In the following code, we apply a user-defined function to a list using list comprehension in Python.
def fn(a):
return 10 * a
lst = [1, 2, 3, 4]
ans = [fn(i) for i in lst]
print(ans)
Output:
The above code applies the fn()
function to each element of lst
using list comprehension and generates a new list ans
containing the transformed values. Finally, it prints the contents of ans
, which will be [10, 20, 30, 40]
.
This code showcases the use of list comprehension to apply a function to each list element and obtain a new list with the transformed values, thereby simplifying data manipulation in Python.
Use the Generator Expression Method to Apply a Function to a List in Python
In Python, a generator expression is a compact and memory-efficient way to create an iterator. It has a similar syntax to a list comprehension but produces a generator object rather than a list.
Generator expressions are enclosed in parentheses and follow a list comprehension syntax with an optional condition. They allow for the dynamic and lazy evaluation of elements, generating values on-the-fly as they are requested rather than computing and storing all values in memory upfront.
Unlike lists, which store all elements simultaneously, generators produce one value at a time, which is useful when dealing with large or infinite sequences. They offer improved performance and reduced memory consumption compared to lists in scenarios where only a subset of elements is required.
Generator expressions are commonly used when memory efficiency is a concern or when working with large datasets. They provide a concise and efficient way to generate values iteratively, making them a valuable tool in Python for handling data streams and generating sequences on demand.
In the following code, we apply a user-defined function to a list using the generator expression in Python.
def fn(a):
return 10 * a
lst = [1, 2, 3, 4]
ans = (fn(i) for i in lst)
print(list(ans))
Output:
The above code applies the fn()
function to each element of lst
using a generator expression and creates a generator object ans
. The generator yields the transformed values on-demand, and by converting it into a list using list()
, we obtain [10, 20, 30, 40]
.
Finally, the code prints the contents of the list. This code demonstrates the use of a generator expression to efficiently apply a function to each list element and dynamically obtain the transformed values.
We can also use all the methods mentioned in this tutorial with built-in functions.
Conclusion
We have explored 4 techniques to apply a function to a list in Python: the for
loop, map()
function, list comprehension, and generator expression.
The for
loop offers simplicity and flexibility but can be more verbose and is the slowest of all the methods. The map()
function provides a concise approach but requires an explicit conversion to a list.
List comprehension combines readability and efficiency, generating a list directly. However, it may consume more memory for larger datasets.
On the other hand, generator expressions are memory-efficient and suitable for large or infinite sequences, but they are non-reusable.
To choose the appropriate method, consider the trade-offs: use the for
loop for basic operations, map()
for a compact approach, list comprehension for generating lists, and generator expressions for memory efficiency with large datasets or infinite sequences. Each method has its strengths and should be applied based on the specific requirements of the task at hand.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python