How to Add a List to a Set in Python
- Add a List to a Set Using the Tuple in Python
-
Add a List to a Set Using the
set.update()
Method in Python
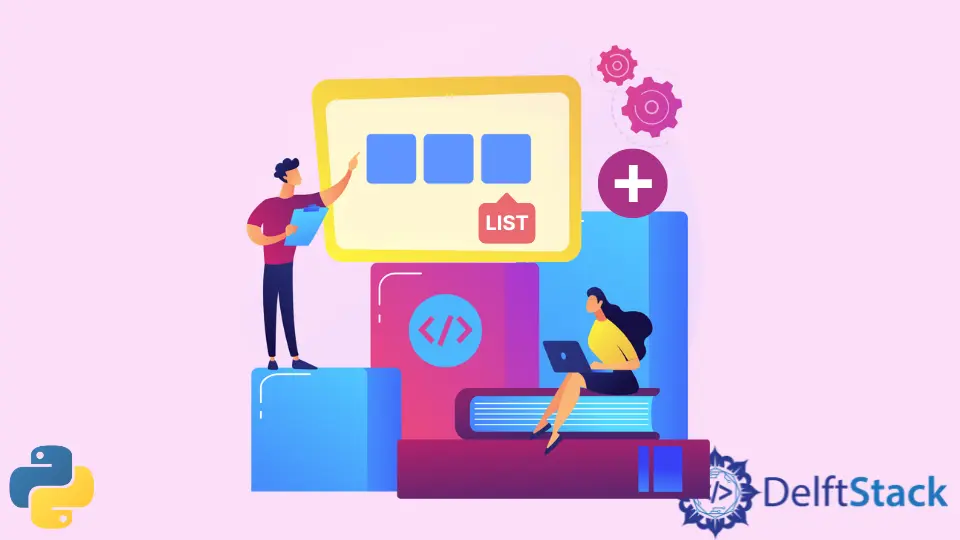
This tutorial will explain multiple methods to add the value of a list to a set in Python. In Python, a set is an unordered and immutable collection of values or objects, and a list is an ordered and mutable collection of objects.
As the list is a mutable data type, it can not be added to a set, as it is not an immutable or hashable object.
Add a List to a Set Using the Tuple in Python
A tuple is an ordered and unchangeable collection of data in Python. Therefore we can add a tuple to a set in Python. We can not add a set to another set because a set is not hashable, but we can add a frozenset
to a set, as it is a hashable data type.
We will first have to convert a list to a tuple and then add it to the set. The below example code demonstrates how to add a complete tuple to a set. We can use the set.add()
method to add an object to the set, the set.add()
method will add the complete tuple as it is to the set.
myset = set((1, 2, 3, 4))
mylist = list([1, 2, 3])
myset.add(tuple(mylist))
print(myset)
Output:
{1, 2, 3, 4, (1, 2, 3)}
Add a List to a Set Using the set.update()
Method in Python
In case we want to add the elements of a list to a set and not the whole list, we can do so using the set.update()
method.
The set.update()
takes an iterable object as input and adds all its elements to the set. If the provided argument is not iterable, the set.update()
method will return a TypeError
.
Since a list is also an iterable object, its elements can also be added to a set using the set.update()
method. The below example code demonstrates how to use the set.update()
method to add all the elements of a list to a set in Python.
myset = set((1, 2, 3, 4))
mylist = list([8, 9, 12])
myset.update(tuple(mylist))
print(myset)
Output:
{1, 2, 3, 4, 8, 9, 12}
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python