How to Add Key to a Dictionary in Python
- Add a New Key/Value Pair to the Dictionary in Python
-
Update an Existing Key/Value Pair With
update()
Function in Python
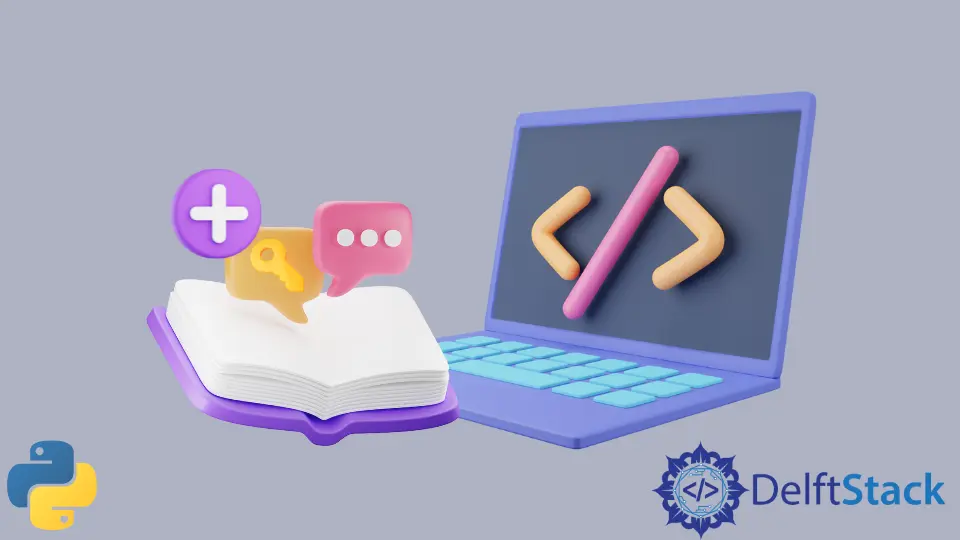
In this tutorial, we will discuss methods to add new keys to a dictionary in Python.
Add a New Key/Value Pair to the Dictionary in Python
The dictionary object holds data in the form of key-value
pairs. Adding a new key/value pair to a dictionary is straightforward in Python. The following code example shows us how to add a new key/value pair to a Python dictionary.
dictionary = {"key1": "value1", "key2": "value2", "key3": "value3"}
print(dictionary)
dictionary["key4"] = "value4"
print(dictionary)
dictionary["key2"] = "value4"
print(dictionary)
Output:
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3', 'key4': 'value4'}
{'key1': 'value1', 'key2': 'value4', 'key3': 'value3', 'key4': 'value4'}
In the above code, we first initialize a dictionary and then add a new key-value
pair to the dictionary using dictionary[key]
. If the key
does not exist, then this new key-value
pair is added to the dictionary. If the key
already exists, then the value of the existing key
is updated to the new value
.
Update an Existing Key/Value Pair With update()
Function in Python
In the previous section, we discussed a method that updates an existing key-value
pair and adds a new key-value
pair to the dictionary if the key is not found. But, it works with only one key/value at a time. If we need to update multiple key-value
pairs in the dictionary, we have to use the update()
function. The update()
function can also add multiple dictionaries into one single dictionary. The following code example shows how we can update multiple key-value
pairs in a dictionary with the update()
function.
dictionary = {"key1": "value1", "key2": "value2", "key3": "value3"}
print(dictionary)
dictionary.update({"key4": "value4", "key2": "value4"})
print(dictionary)
Output:
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
{'key1': 'value1', 'key2': 'value4', 'key3': 'value3', 'key4': 'value4'}
In the above code, we first initialize a dictionary and then update multiple key/value pairs using the update()
function. If the key
does not exist, then a new key-value
pair is added to the dictionary. If the key
already exists, then the existing key
has the new value
.
As it is clear from the above example, the update()
function reduces the code if you want to update multiple key-value
pairs simultaneously.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn