How to Write a Pandas DataFrame to CSV
-
Syntax of
pandas.DataFrame.to_csv()
Function -
Write a DataFrame Into CSV File Using the
pandas.DataFrame.to_csv()
Function -
Write a DataFrame Into CSV File Using the
pandas.DataFrame.to_csv()
Function Ignoring the Indices -
Specify a Seperator in the
pandas.DataFrame.to_csv()
Function
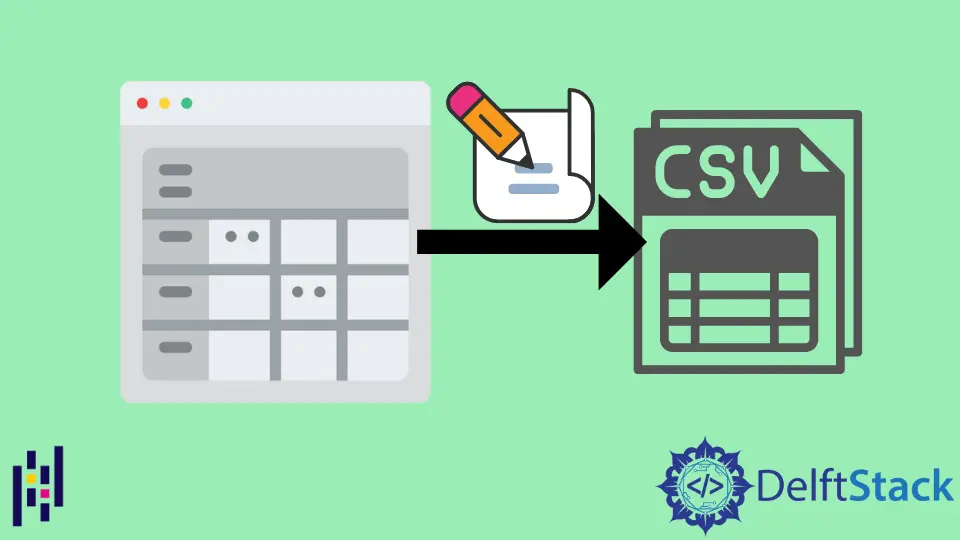
This tutorial explains how we can write a DataFrame into a CSV file using the pandas.DataFrame.to_csv()
function. The pandas.DataFrame.to_csv()
function writes the entries of DataFrame into a CSV file.
Syntax of pandas.DataFrame.to_csv()
Function
pandas.DataFrame.to_csv(
path_or_buf=None,
sep=",",
na_rep="",
float_format=None,
columns=None,
header=True,
index=True,
index_label=None,
mode="w",
encoding=None,
compression="infer",
quoting=None,
quotechar='""',
line_terminator=None,
chunksize=None,
date_format=None,
doublequote=True,
escapechar=None,
decimal=".",
)
Write a DataFrame Into CSV File Using the pandas.DataFrame.to_csv()
Function
import pandas as pd
mid_term_marks = {
"Student": ["Kamal", "Arun", "David", "Thomas", "Steven"],
"Economics": [10, 8, 6, 5, 8],
"Fine Arts": [7, 8, 5, 9, 6],
"Mathematics": [7, 3, 5, 8, 5],
}
mid_term_marks_df = pd.DataFrame(mid_term_marks)
print(mid_term_marks_df)
Output:
Student Economics Fine Arts Mathematics
0 Kamal 10 7 7
1 Arun 8 8 3
2 David 6 5 5
3 Thomas 5 9 8
4 Steven 8 6 5
Then we will write the DataFrame mid_term_marks_df
into a CSV file.
import pandas as pd
mid_term_marks = {
"Student": ["Kamal", "Arun", "David", "Thomas", "Steven"],
"Economics": [10, 8, 6, 5, 8],
"Fine Arts": [7, 8, 5, 9, 6],
"Mathematics": [7, 3, 5, 8, 5],
}
mid_term_marks_df = pd.DataFrame(mid_term_marks)
mid_term_marks_df.to_csv("midterm.csv")
It will create a file named midterm.csv
and write the DataFrame values into the file where the adjacent values in a row are separated by a comma ,
.
The content of the midterm.csv
file will be :
,Student,Economics,Fine Arts,Mathematics
0,Kamal,10,7,7
1,Arun,8,8,3
2,David,6,5,5
3,Thomas,5,9,8
4,Steven,8,6,5
By default, the pandas.DataFrame.to_csv()
function also writes the indices of DataFrame into the CSV, but the index might not always be useful in all cases.
Write a DataFrame Into CSV File Using the pandas.DataFrame.to_csv()
Function Ignoring the Indices
To ignore the indices, we can set index=False
in the pandas.DataFrame.to_csv()
function.
import pandas as pd
mid_term_marks = {
"Student": ["Kamal", "Arun", "David", "Thomas", "Steven"],
"Economics": [10, 8, 6, 5, 8],
"Fine Arts": [7, 8, 5, 9, 6],
"Mathematics": [7, 3, 5, 8, 5],
}
mid_term_marks_df = pd.DataFrame(mid_term_marks)
mid_term_marks_df.to_csv("midterm.csv", index=False)
In this case, the content of the midterm.csv
file will be:
Student,Economics,Fine Arts,Mathematics
Kamal,10,7,7
Arun,8,8,3
David,6,5,5
Thomas,5,9,8
Steven,8,6,5
Sometimes we may get UnicodeEncodeError
while writing the contents of a DataFrame into a CSV file. In such cases, we can set encoding='utf-8'
which enables the utf-8
encoding format.
Specify a Seperator in the pandas.DataFrame.to_csv()
Function
By default, while writing the DataFrame into a CSV file, the values are separated by a comma. If we want to use any other symbols as a separator, we can specify it using the sep
parameter.
import pandas as pd
mid_term_marks = {
"Student": ["Kamal", "Arun", "David", "Thomas", "Steven"],
"Economics": [10, 8, 6, 5, 8],
"Fine Arts": [7, 8, 5, 9, 6],
"Mathematics": [7, 3, 5, 8, 5],
}
mid_term_marks_df = pd.DataFrame(mid_term_marks)
mid_term_marks_df.to_csv("midterm.csv", index=False, sep="\t")
In this case, the content of the midterm.csv
file will be:
Student Economics Fine Arts Mathematics
Kamal 10 7 7
Arun 8 8 3
David 6 5 5
Thomas 5 9 8
Steven 8 6 5
Here the values are separated by a tab.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn