How to Pandas DataFrame Dimensions
-
Display
DataFrame
Size in Pandas Python Usingdataframe.size
Property -
Display
DataFrame
Shape in Pandas Python Using thedataframe.shape
Property -
Display
DataFrame
Dimension in Pandas Python Using thedataframe.ndim
Property - Conclusion
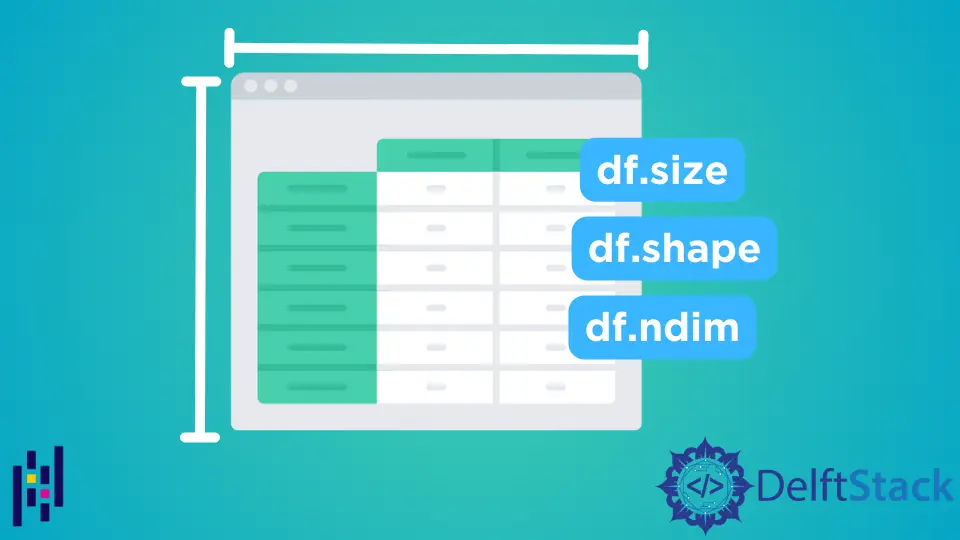
Python Pandas library comes with a bundle of properties that helps us to perform various tasks. While working with pandas DataFrame
, we may need to display the size, shape, and dimension of a DataFrame
, and this task we can easily do using some popular pandas properties such as df.size
, df.shape
, and df.ndim
.
This article will demonstrate how to return or calculate the size
, shape
, and dimensions
of a DataFrame
using python pandas properties such as dataframe.size
, dataframe.shape
, and dataframe.ndim
.
Display DataFrame
Size in Pandas Python Using dataframe.size
Property
In Python Pandas, the dataframe.size
property is used to display the size of Pandas DataFrame
. It returns the size of the DataFrame
or a series which is equivalent to the total number of elements. If you want to calculate the size of the series
, it will return the number of rows. In the case of a DataFrame
, it will return the rows multiplied by the columns.
In the following example, we imported or read a .csv
file using pd.csvread()
and created a DataFrame
. Using the Pandas property dataframe.size
, we displayed the size of the given DataFrame
.
Example Code:
import pandas as pd
# create a dataframe after reading .csv file
dataframe = pd.read_csv(r"C:\Users\DELL\OneDrive\Desktop\CSV_files\Samplefile1.csv")
# print dataframe
print(dataframe)
# displaying dataframe size
print("The size of the DataFrame is: ", dataframe.size)
Output:
Name Team Position Age
0 Adam Donachie "BAL" "Catcher" 22.99
1 Paul Bako "BAL" "Catcher" 34.69
2 Ramon Hernandez "BAL" "Catcher" 30.78
3 Kevin Millar "BAL" "First Baseman" 35.43
4 Chris Gomez "BAL" "First Baseman" 35.71
5 Brian Roberts "BAL" "Second Baseman" 29.39
6 Miguel Tejada "BAL" "Shortstop" 30.77
7 Melvin Mora "BAL" "Third Baseman" 35.07
8 Aubrey Huff "BAL" "Third Baseman" 30.19
9 Adam Stern "BAL" "Outfielder" 27.05
The size of the DataFrame is: 40
Display DataFrame
Shape in Pandas Python Using the dataframe.shape
Property
The dataframe.shape
property pandas python returns the tuples shape in the form of (rows, columns)
of a DataFrame
or series
.
In the code sample we provided below, we have created a DataFrame
after reading a .csv file. To return the shape of dataframe, we use the dataframe.shape
property in the following way:
Example Code:
import pandas as pd
# create a dataframe after reading .csv file
dataframe = pd.read_csv(r"C:\Users\DELL\OneDrive\Desktop\CSV_files\Samplefile1.csv")
# print dataframe
print(dataframe)
# displaying dataframe shape
print("The shape of the DataFrame is: ", dataframe.shape)
Output:
Name Team Position Age
0 Adam Donachie "BAL" "Catcher" 22.99
1 Paul Bako "BAL" "Catcher" 34.69
2 Ramon Hernandez "BAL" "Catcher" 30.78
3 Kevin Millar "BAL" "First Baseman" 35.43
4 Chris Gomez "BAL" "First Baseman" 35.71
5 Brian Roberts "BAL" "Second Baseman" 29.39
6 Miguel Tejada "BAL" "Shortstop" 30.77
7 Melvin Mora "BAL" "Third Baseman" 35.07
8 Aubrey Huff "BAL" "Third Baseman" 30.19
9 Adam Stern "BAL" "Outfielder" 27.05
The shape of the DataFrame is: (10, 4)
Display DataFrame
Dimension in Pandas Python Using the dataframe.ndim
Property
The Pandas dataframe.ndim
property returns the dimension of a series
or a DataFrame
. For all kinds of dataframes
and series
, it will return dimension 1
for series that only consists of rows and will return 2
in case of DataFrame
or two-dimensional data.
In the sample code below, we have created a DataFrame
by importing the .csv file. To return the dimension of the DataFrame
, we used dataframe.ndim
pandas property that is 2
in the case of pandas DataFrame
.
Example Code:
import pandas as pd
# create a dataframe after reading .csv file
dataframe = pd.read_csv(r"C:\Users\DELL\OneDrive\Desktop\CSV_files\Samplefile1.csv")
# print dataframe
print(dataframe)
# displaying dataframe dimension
print("The dimension of the DataFrame is: ", dataframe.ndim)
Output:
Name Team Position Age
0 Adam Donachie "BAL" "Catcher" 22.99
1 Paul Bako "BAL" "Catcher" 34.69
2 Ramon Hernandez "BAL" "Catcher" 30.78
3 Kevin Millar "BAL" "First Baseman" 35.43
4 Chris Gomez "BAL" "First Baseman" 35.71
5 Brian Roberts "BAL" "Second Baseman" 29.39
6 Miguel Tejada "BAL" "Shortstop" 30.77
7 Melvin Mora "BAL" "Third Baseman" 35.07
8 Aubrey Huff "BAL" "Third Baseman" 30.19
9 Adam Stern "BAL" "Outfielder" 27.05
The dimension of the DataFrame is: 2
Conclusion
We explored three different Pandas properties such as dataframe.size
, dataframe.shape
, and dataframe.ndim
through which we can easily return the size, shape, and dimension of the DataFrame
or series
. I hope all the above-explained demonstrations will help you to understand the basic use of pandas properties.