How to Move Column to Front in Pandas DataFrame
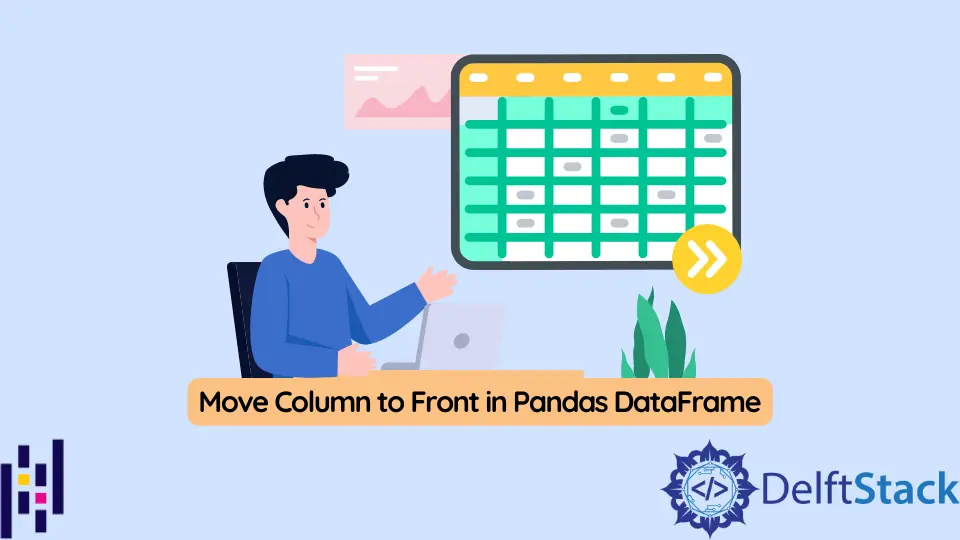
Python has a data analysis library called Pandas
. We can perform many different types of manipulation on a DataFrame using Pandas in Python.
This guide will explain the methods to reorder the columns in a pandas DataFrame such that it moves to the front.
Move a Column to the Front in Pandas DataFrame
Suppose we want to rearrange the columns’ positions in the DataFrame. Pandas provide the insert()
and reindex()
methods that move a single column to the front of the Pandas DataFrame.
Use the pop()
and the insert()
Methods
The idea is to remove the column we want to move to the front using the pop()
method. Then insert the column again into the DataFrame using the insert()
method by specifying the location to insert.
In the following code, we have a DataFrame consisting of student records. Our goal is to move the column Name
to the front.
First, we will remove it using the pop()
method, the pop()
method takes the column’s label that we want to delete as a parameter and returns it. We stored the returned column in the col
.
Through the insert()
method, we pushed the column back to the DataFrame by defining its location as loc=0
(first column position), the column name, and the actual column).
Example Code:
# Python 3.x
import pandas as pd
student = {
"Course": ["Java", "Python", "C++", "Dart"],
"Marks": [70, 80, 90, 60],
"Age": [19, 20, 21, 19],
"Name": ["Jhon", "Aliya", "Nate", "Amber"],
}
df = pd.DataFrame(student)
print(df)
col = df.pop("Name")
df.insert(loc=0, column="Name", value=col)
print(df)
Output:
$python3 Main.py
Course Marks Age Name
0 Java 70 19 Jhon
1 Python 80 20 Aliya
2 C++ 90 21 Nate
3 Dart 60 19 Amber
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
Use the reindex()
Method
We can rearrange the columns by re-indexing
them. The reindex()
method reorders the columns.
This method is available in the pandas.DataFrame
module. We’ll provide the list of column names in our desired order and pass the list
and the DataFrame
to the reindex()
method to return the updated DataFrame.
Example Code:
# Python 3.x
import pandas as pd
student = {
"Course": ["Java", "Python", "C++", "Dart"],
"Marks": [70, 80, 90, 60],
"Age": [19, 20, 21, 19],
"Name": ["Jhon", "Aliya", "Nate", "Amber"],
}
df = pd.DataFrame(student)
print(df)
df = pd.DataFrame.reindex(df, columns=["Name", "Course", "Marks", "Age"])
print(df)
Output:
$python3 Main.py
Course Marks Age Name
0 Java 70 19 Jhon
1 Python 80 20 Aliya
2 C++ 90 21 Nate
3 Dart 60 19 Amber
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn