How to Create Pandas Dataframe From a List
- Method 1: Using a Simple List
- Method 2: Using a List of Lists
- Method 3: Using a List of Dictionaries
- Method 4: Using NumPy Arrays
- Conclusion
- FAQ
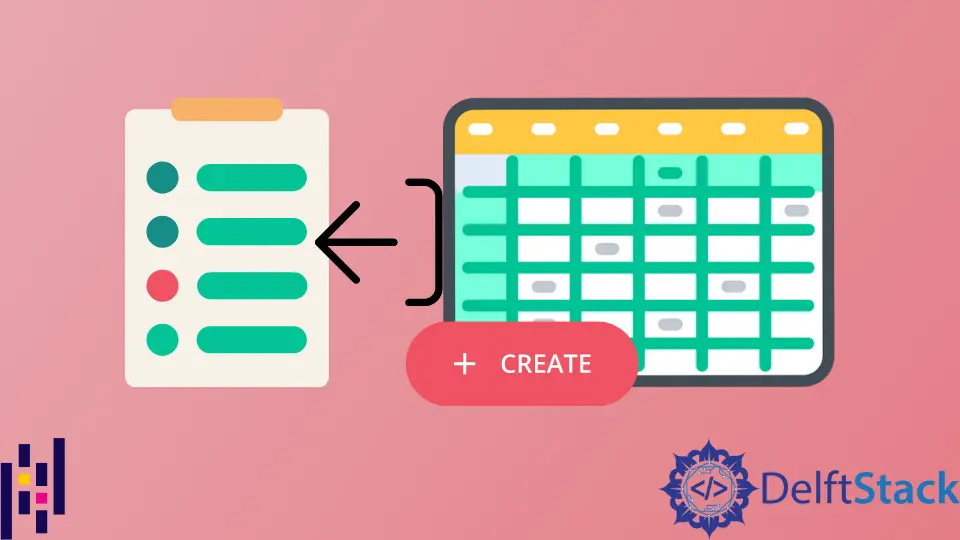
Creating a Pandas DataFrame from a list can seem daunting at first, especially if you’re new to Python or data manipulation. However, once you understand the fundamentals, it becomes an effortless task.
In this tutorial, we will walk you through the process step-by-step, ensuring that you grasp the concepts clearly. Whether you’re compiling data for analysis, visualizations, or machine learning, knowing how to create a DataFrame from a list is a valuable skill. We will explore various methods to achieve this, complete with code examples and explanations. Let’s dive in and unlock the potential of Pandas!
Method 1: Using a Simple List
One of the most straightforward ways to create a DataFrame in Pandas is by using a simple list. This method is particularly handy when you have a one-dimensional list of data that you want to convert into a DataFrame.
import pandas as pd
data = [10, 20, 30, 40, 50]
df = pd.DataFrame(data, columns=['Numbers'])
print(df)
Output:
Numbers
0 10
1 20
2 30
3 40
4 50
In this example, we first import the Pandas library. We then create a list called data
containing five integers. To convert this list into a DataFrame, we use the pd.DataFrame()
function and pass our list as the first argument. We also specify the column name as ‘Numbers’ using the columns
parameter. Finally, we print the DataFrame to see the result. This method is simple and effective for creating a DataFrame when you have a single list of values.
Method 2: Using a List of Lists
If your data is more complex, such as a list of lists where each sub-list represents a row of data, you can easily create a DataFrame using this structure.
import pandas as pd
data = [[1, 'Alice', 25], [2, 'Bob', 30], [3, 'Charlie', 35]]
df = pd.DataFrame(data, columns=['ID', 'Name', 'Age'])
print(df)
Output:
ID Name Age
0 1 Alice 25
1 2 Bob 30
2 3 Charlie 35
In this code snippet, we define a list of lists called data
. Each sub-list contains an ID, a name, and an age. We then create a DataFrame using the same pd.DataFrame()
function, passing the list of lists as the first argument and specifying the column names. The resulting DataFrame displays each person’s details in a structured format. This method is useful for organizing multi-dimensional data and makes it easy to manipulate and analyze.
Method 3: Using a List of Dictionaries
Another powerful way to create a DataFrame is by using a list of dictionaries. This approach is particularly useful when your data has different attributes, as each dictionary can represent a row with named columns.
import pandas as pd
data = [
{'ID': 1, 'Name': 'Alice', 'Age': 25},
{'ID': 2, 'Name': 'Bob', 'Age': 30},
{'ID': 3, 'Name': 'Charlie', 'Age': 35}
]
df = pd.DataFrame(data)
print(df)
Output:
ID Name Age
0 1 Alice 25
1 2 Bob 30
2 3 Charlie 35
In this example, we create a list called data
, where each element is a dictionary representing a person with unique attributes. When we pass this list to the pd.DataFrame()
function, Pandas automatically infers the column names from the dictionary keys. The resulting DataFrame is well-structured and easy to read. This method is particularly beneficial when dealing with JSON-like data or when your data has varying fields, allowing for greater flexibility.
Method 4: Using NumPy Arrays
If you’re working with numerical data, you might find it convenient to create a DataFrame from a NumPy array. This method is efficient and can handle large datasets with ease.
import pandas as pd
import numpy as np
data = np.array([[1, 'Alice', 25], [2, 'Bob', 30], [3, 'Charlie', 35]])
df = pd.DataFrame(data, columns=['ID', 'Name', 'Age'])
print(df)
Output:
ID Name Age
0 1 Alice 25
1 2 Bob 30
2 3 Charlie 35
Here, we first import the NumPy library alongside Pandas. We create a NumPy array called data
, which contains the same information as before. When creating the DataFrame, we specify the column names just like in previous examples. One thing to note is that when using NumPy arrays with mixed data types, all values will be converted to strings. This method is efficient for numerical computations and can be particularly useful when working with large datasets.
Conclusion
Creating a Pandas DataFrame from a list is a fundamental skill in data manipulation and analysis. Whether you’re using a simple list, a list of lists, dictionaries, or NumPy arrays, Pandas provides flexible methods to transform your data into a structured format. This tutorial has covered various techniques, each suited for different types of data. With these tools at your disposal, you can efficiently organize and analyze your data, making your work easier and more productive. Keep practicing, and soon you’ll be a Pandas pro!
FAQ
-
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). -
Can I create a DataFrame from a CSV file?
Yes, you can create a DataFrame from a CSV file using thepd.read_csv()
function. -
What are the advantages of using a DataFrame?
DataFrames provide powerful data manipulation capabilities, including filtering, grouping, and merging datasets, making data analysis much easier. -
How do I convert a DataFrame back to a list?
You can convert a DataFrame back to a list using thedf.values.tolist()
method.
- Is it possible to create a DataFrame with mixed data types?
Yes, a DataFrame can contain mixed data types in different columns, allowing for flexibility in data representation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn