How to Add Empty Column to Pandas DataFrame
-
Add Empty Columns in Pandas
DataFrame
Using theassignment operator
-
Add an Empty Column in Pandas
DataFrame
Using theDataFrame.assign()
Method -
Add an Empty Column in Pandas
DataFrame
Using theDataFrame.reindex()
Method -
Add an Empty Column in Pandas
DataFrame
Using theDataFrame.insert()
Method -
Add an Empty Column in Pandas
DataFrame
Using theDataFrame.apply()
Method - Conclusion
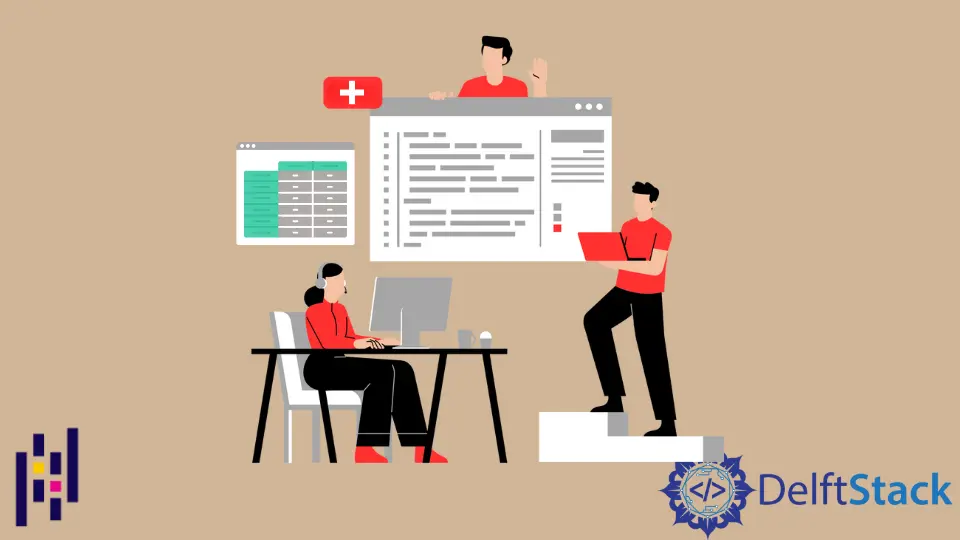
Pandas also offer a feature to add one or multiple empty columns to the DataFrame
(table). There are different ways available through which we can easily add empty columns in Pandas DataFrame
.
We will show in this tutorial how to add one or more empty columns in Pandas DataFrame
using various approaches such as using the assignment operator
and by using the assign()
, insert()
, reindex()
, and apply()
methods. We will also show the implementation of each method to explain the working of each approach briefly.
Add Empty Columns in Pandas DataFrame
Using the assignment operator
Using the assignment operator or empty string, we can add empty columns in Pandas DataFrame
. And using this approach, the null or NaN
values are assigned to any column in the DataFrame
.
In the following example, we have created a DataFrame
, and then using the assignment operator
, we assigned empty string and NaN
values to two newly added columns as in the Pandas DataFrame
. These columns are Address
and Designation
. Using the NumPy
library, we will import the NaN
values into the DataFrame
column.
Let’s see how to add the empty columns to the DataFrame
in Pandas using the assignment operator
or empty string
.
Example Code:
import pandas as pd
import numpy as np
company_data = {
"Employee Name": ["Samreena", "Mirha", "Asif", "Raees"],
"Employee ID": [101, 102, 103, 104],
}
dataframe = pd.DataFrame(company_data)
print("------------ Original DataFrame --------------\n", dataframe)
# Add empty column using Assignment operator
dataframe["Blank_Column"] = " "
dataframe["Address"] = np.nan
dataframe["Designation"] = None
print("------------ After Adding Empty Columns ---------------\n", dataframe)
Output:
------------ Original DataFrame --------------
Employee Name Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
------------ After Adding Empty Columns ---------------
Employee Name Employee ID Designation Address
0 Samreena 101 NaN
1 Mirha 102 NaN
2 Asif 103 NaN
3 Raees 104 NaN
Add an Empty Column in Pandas DataFrame
Using the DataFrame.assign()
Method
The DataFrame.assign()
method is used to add one or multiple columns to the DataFrame
. Applying the assign()
method on a DataFrame
returns a new DataFrame
after adding the new empty columns in the existing Pandas DataFrame
.
Example Code:
import pandas as pd
import numpy as np
company_data = {
"Employee Name": ["Samreena", "Mirha", "Asif", "Raees"],
"Employee ID": [101, 102, 103, 104],
}
dataframe1 = pd.DataFrame(company_data)
print("------------ Original DataFrame --------------\n", dataframe1)
# Add empty column into the DataFrame using assign() method
dataframe2 = dataframe1.assign(Designation=" ", Empty_column=np.nan, Address=None)
print("------------ After Adding Empty Columns ---------------\n", dataframe2)
Output:
------------ Original DataFrame --------------
Employee Name Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
------------ After Adding Empty Columns ---------------
Employee Name Employee ID Designation Empty_column Address
0 Samreena 101 NaN None
1 Mirha 102 NaN None
2 Asif 103 NaN None
3 Raees 104 NaN None
Add an Empty Column in Pandas DataFrame
Using the DataFrame.reindex()
Method
The DataFrame.reindex()
method assigned NaN
values to empty columns in the Pandas DataFrame
. This reindex()
method takes the list of the existing and newly added columns. Using this method, we can add empty columns at any index location into the DataFrame
.
In the following example, we have created a new DataFrame
with two-columns names as Employee Name
and Employee ID
. Later, we used dataframe.reindex()
method to add two more new columns, Address
and Designation
, to the columns list with assigned NaN
values.
Example Code:
import pandas as pd
import numpy as np
company_data = {
"Employee Name": ["Samreena", "Mirha", "Asif", "Raees"],
"Employee ID": [101, 102, 103, 104],
}
dataframe1 = pd.DataFrame(company_data)
print("------------ Original DataFrame --------------\n", dataframe1)
# Pandas Add empty columns to the DataFrame using reindex() method
dataframe2 = dataframe1.reindex(
columns=dataframe1.columns.tolist() + ["Designation", "Address"]
)
print("------------ After Adding Empty Columns ---------------\n", dataframe2)
Output:
------------ Original DataFrame --------------
Employee Name Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
------------ After Adding Empty Columns ---------------
Employee Name Employee ID Designation Address
0 Samreena 101 NaN NaN
1 Mirha 102 NaN NaN
2 Asif 103 NaN NaN
3 Raees 104 NaN NaN
Add an Empty Column in Pandas DataFrame
Using the DataFrame.insert()
Method
The DataFrame.insert()
method inserts an empty column at any index position (beginning, middle, end, or specified location) in the Pandas DataFrame
.
Example Code:
import pandas as pd
import numpy as np
company_data = {
"Employee Name": ["Samreena", "Mirha", "Asif", "Raees"],
"Employee ID": [101, 102, 103, 104],
}
dataframe = pd.DataFrame(company_data)
print("------------ Original DataFrame --------------\n", dataframe)
# Pandas Add empty columns to the DataFrame using insert() method
dataframe.insert(1, "Designation", "")
print("------------ After Adding Empty Columns ---------------\n", dataframe)
Output:
------------ Original DataFrame --------------
Employee Name Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
------------ After Adding Empty Columns ---------------
Employee Name Designation Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
Add an Empty Column in Pandas DataFrame
Using the DataFrame.apply()
Method
Using the DataFrame.apply()
method and Lambda
function, we can also add empty columns to the Pandas DataFrame
. See the following example to add empty columns to the DataFrame
in Pandas using the DataFrame.apply()
method.
Example Code:
import pandas as pd
import numpy as np
company_data = {
"Employee Name": ["Samreena", "Mirha", "Asif", "Raees"],
"Employee ID": [101, 102, 103, 104],
}
dataframe = pd.DataFrame(company_data)
print("------------ Original DataFrame --------------\n", dataframe)
# Pandas Add empty columns to the DataFrame using apply() method
dataframe["Empty_column"] = dataframe.apply(lambda _: " ", axis=1)
print("------------ After Adding Empty Columns ---------------\n", dataframe)
Output:
------------ Original DataFrame --------------
Employee Name Employee ID
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
------------ After Adding Empty Columns ---------------
Employee Name Employee ID Empty_column
0 Samreena 101
1 Mirha 102
2 Asif 103
3 Raees 104
Conclusion
In this tutorial, we introduced different methods such as assign()
, insert()
, apply()
, and reindex()
to add one or multiple empty columns in the Pandas DataFrame
. We have also shown how we can add the empty columns to the DataFrame
using the assignment operator
.