How to Get a Value From a Cell of a Pandas DataFrame
-
iloc
to Get Value From a Cell of a PandasDataFrame
-
iat
andat
to Get Value From a Cell of a PandasDataFrame
-
df['col_name'].values[]
to Get Value From a Cell of a Pandas Dataframe
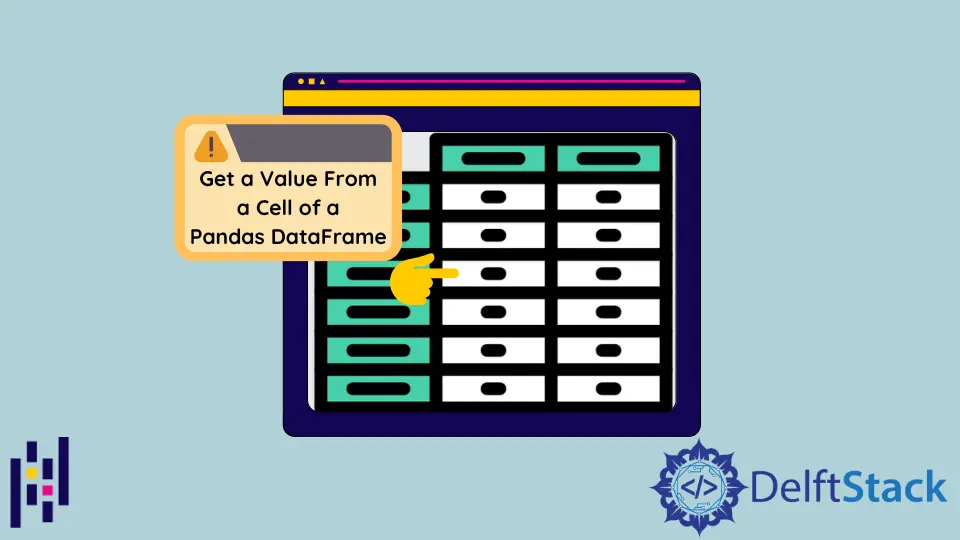
We will introduce methods to get the value of a cell in Pandas DataFrame
. They include iloc
and iat
. ['col_name'].values[]
is also a solution especially if we don’t want to get the return type as pandas.Series
.
iloc
to Get Value From a Cell of a Pandas DataFrame
iloc
is the most efficient way to get a value from the cell of a Pandas DataFrame
. Suppose we have a DataFrame
with the columns’ names as price
and stock
, and we want to get a value from the 3rd row to check the price and stock availability.
First, we need to access rows and then the value using the column name.
Example Codes:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"name": ["orange", "banana", "lemon", "mango", "apple"],
"price": [2, 3, 7, 21, 11],
"stock": ["Yes", "No", "Yes", "No", "Yes"],
}
)
print(df.iloc[2]["price"])
print(df.iloc[2]["stock"])
Output:
7
Yes
iloc
gets rows (or columns) at particular positions in the index. That’s why it only takes an integer as the argument. And loc
gets rows (or columns) with the given labels from the index.
iat
and at
to Get Value From a Cell of a Pandas DataFrame
iat
and at
are fast accesses for scalars to get the value from a cell of a Pandas DataFrame
.
Example Codes:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"name": ["orange", "banana", "lemon", "mango", "apple"],
"price": [2, 3, 7, 21, 11],
"stock": ["Yes", "No", "Yes", "No", "Yes"],
}
)
print(df.iat[0, 0])
print(df.at[1, "stock"])
Output:
orange
No
To get the last row entry, we will use at[df.index[-1],'stock']
.
Example Codes:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"name": ["orange", "banana", "lemon", "mango", "apple"],
"price": [2, 3, 7, 21, 11],
"stock": ["Yes", "No", "Yes", "No", "Yes"],
}
)
print(df.at[df.index[-1], "stock"])
Output:
Yes
df['col_name'].values[]
to Get Value From a Cell of a Pandas Dataframe
df['col_name'].values[]
will first convert datafarme
column into 1-D array then access the value at index of that array:
Example Codes:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"name": ["orange", "banana", "lemon", "mango", "apple"],
"price": [2, 3, 7, 21, 11],
"stock": ["Yes", "No", "Yes", "No", "Yes"],
}
)
print(df["stock"].values[0])
Output:
Yes
It does not return a pandas.Series
, and it’s the simplest to use.