Difference Between Pandas apply, map and applymap
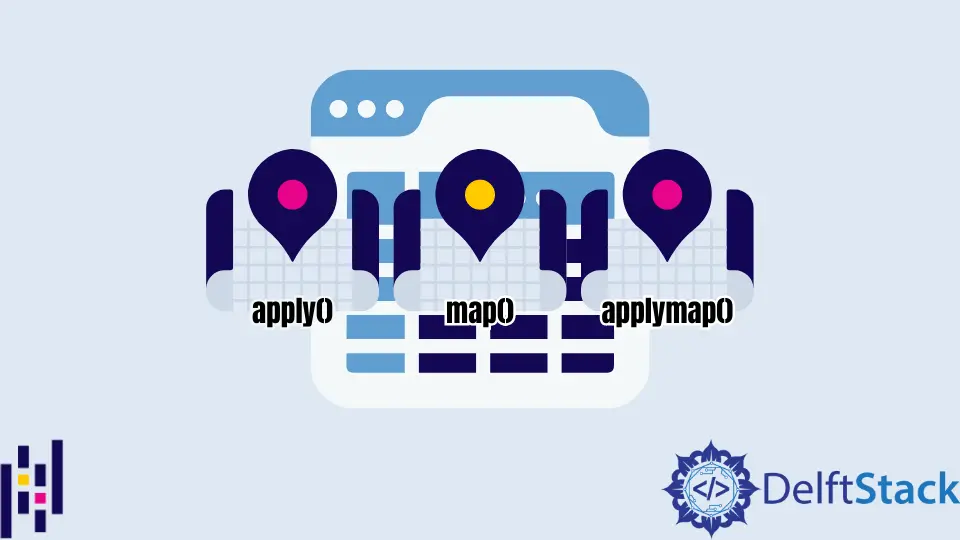
This tutorial explains the difference between apply()
, map()
and applymap()
methods in Pandas.
The function associated with applymap()
is applied to all the elements of the given DataFrame, and hence applymap()
method is defined for DataFrames only. Similarly, the function associated with the apply()
method can be applied to all the elements of DataFrame or Series
, and hence apply()
method is defined for both Series and DataFrame objects. The map()
method can only be defined for Series
objects in Pandas.
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print(df, "\n")
Output:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
We will use the DataFrame df
displayed in the above example to explain the difference between apply()
, map()
, and applymap()
methods in Pandas.
pandas.DataFrame.applymap()
Syntax
DataFrame.applymap(func, na_action=None)
It applies the function func
to every element of the DataFrame
.
Example: Use applymap()
Method to Alter Items of a DataFrame
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print("Initial DataFrame:")
print(df, "\n")
scaled_df = df.applymap(lambda a: a * 10)
print("Scaled DataFrame:")
print(scaled_df, "\n")
Output:
Initial DataFrame:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
Scaled DataFrame:
Col 1 Col 2 Col 3
A 300 230 850
B 400 350 870
C 500 650 900
D 600 450 890
It multiplies every element of df
DataFrame and stores the result in scaled_df
DataFrame. We pass a lambda
function as an argument to the applymap()
function, which returns a value by multiplying the input with 10
. So every element of the DataFrame df
is scaled by 10.
We can also use the for
loop to iterate over each element in the df
DataFrame, but it makes our code less readable, messy, and less efficient. applymap()
is an alternative approach that makes the code more readable and efficient.
Besides mathematical operations, we can also perform other operations to the elements of the DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print("Initial DataFrame:")
print(df, "\n")
altered_df = df.applymap(lambda a: str(a) + ".00")
print("Altered DataFrame:")
print(altered_df, "\n")
Output:
Initial DataFrame:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
Altered DataFrame:
Col 1 Col 2 Col 3
A 30.00 23.00 85.00
B 40.00 35.00 87.00
C 50.00 65.00 90.00
D 60.00 45.00 89.00
It appends .00
at the end of each element in the DataFrame df
.
map()
Method in Pandas
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print("Initial DataFrame:")
print(df, "\n")
df["Col 1"] = df["Col 1"].map(lambda x: x / 100)
print("DataFrame after altering Col 1:")
print(df)
Output:
Initial DataFrame:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
DataFrame after altering Col 1:
Col 1 Col 2 Col 3
A 0.3 23 85
B 0.4 35 87
C 0.5 65 90
D 0.6 45 89
We can only use the map()
method with the particular column of a DataFrame.
apply()
Method in Pandas
apply()
Method to Alter Entire DataFrame in Pandas
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print("Initial DataFrame:")
print(df, "\n")
altered_df = df.apply(lambda x: x / 100)
print("Altered DataFrame:")
print(altered_df, "\n")
Output:
Initial DataFrame:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
Altered DataFrame:
Col 1 Col 2 Col 3
A 0.3 0.23 0.85
B 0.4 0.35 0.87
C 0.5 0.65 0.90
D 0.6 0.45 0.89
apply()
Method to Alter Particular Column Only in Pandas
import pandas as pd
df = pd.DataFrame(
{
"Col 1": [30, 40, 50, 60],
"Col 2": [23, 35, 65, 45],
"Col 3": [85, 87, 90, 89],
},
index=["A", "B", "C", "D"],
)
print("Initial DataFrame:")
print(df, "\n")
df["Col 1"] = df["Col 1"].apply(lambda x: x / 100)
print("DataFrame after altering Col 1:")
print(df)
Output:
Initial DataFrame:
Col 1 Col 2 Col 3
A 30 23 85
B 40 35 87
C 50 65 90
D 60 45 89
DataFrame after altering Col 1:
Col 1 Col 2 Col 3
A 0.3 23 85
B 0.4 35 87
C 0.5 65 90
D 0.6 45 89
Hence, from the above examples, we can see that apply()
method can be used to apply a particular function to either all elements of the entire DataFrame or to all the elements of a particular column.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn