How to Append List to DataFrame Pandas
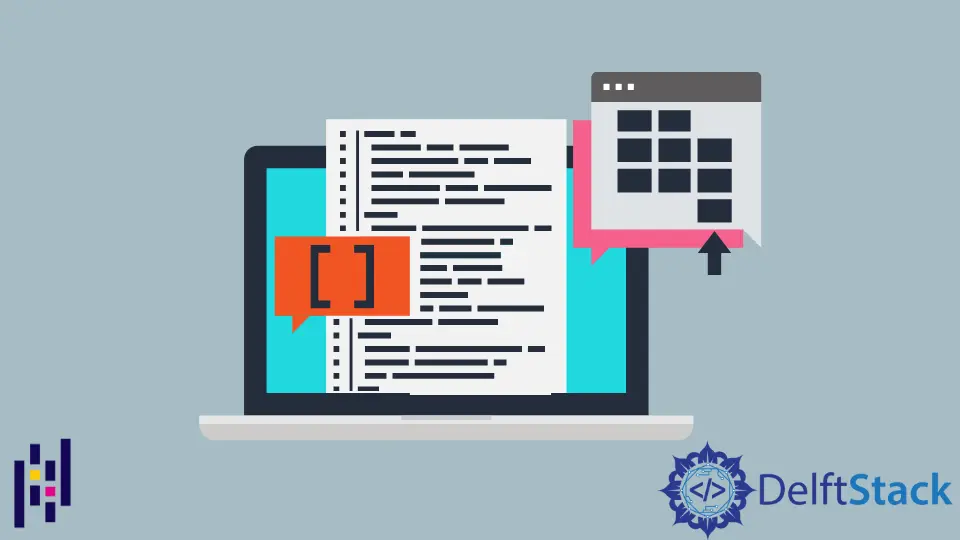
This guide will show how to append a list to a pandas DataFrame as a row. Append means to insert the list as a row at the bottom of the pandas DataFrame.
Append a List to a Pandas DataFrame
Here, we will look at the two methods to insert a list into a pandas DataFrame. One is the dataframe.append()
method, and the other is the dataframe.loc[]
method.
Use the dataframe.append()
Method
We have created a Pandas DataFrame consisting of students’ records in the following code. Then we made a list containing a single student record.
We append it to the pandas DataFrame using the append()
method. We have passed the list
as the new record to insert and the column names
to the append()
method.
This method inserts the list as the last record into the DataFrame and returns the new DataFrame.
Example Code:
# Python 3.x
import pandas as pd
student = {
"Name": ["Jhon", "Aliya", "Nate", "Amber"],
"Course": ["Java", "Python", "C++", "Dart"],
"Marks": [70, 80, 90, 60],
"Age": [19, 20, 21, 19],
}
df = pd.DataFrame(student)
print(df)
list = ["Ben", "JavaScript", 85, 21]
df = df.append(
pd.DataFrame([list], columns=["Name", "Course", "Marks", "Age"]), ignore_index=True
)
print(df)
Output:
$python3 Main.py
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
4 Ben JavaScript 85 21
Use the dataframe.loc[]
Method
The loc[]
property of a DataFrame selects records at a specified index. We have specified len(df)
as the location to insert the record.
It returns the length of the DataFrame. The length is equal to the last index+1
.
We will access this location and assign the list as a record to that location using loc[len(df)]
.
Example Code:
# Python 3.x
import pandas as pd
student = {
"Name": ["Jhon", "Aliya", "Nate", "Amber"],
"Course": ["Java", "Python", "C++", "Dart"],
"Marks": [70, 80, 90, 60],
"Age": [19, 20, 21, 19],
}
df = pd.DataFrame(student)
display(df)
list = ["Ben", "JavaScript", 85, 21]
df.loc[len(df)] = list
display(df)
Output:
$python3 Main.py
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
Name Course Marks Age
0 Jhon Java 70 19
1 Aliya Python 80 20
2 Nate C++ 90 21
3 Amber Dart 60 19
4 Ben JavaScript 85 21
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn