How to Test a Variable's Data Type Using PowerShell
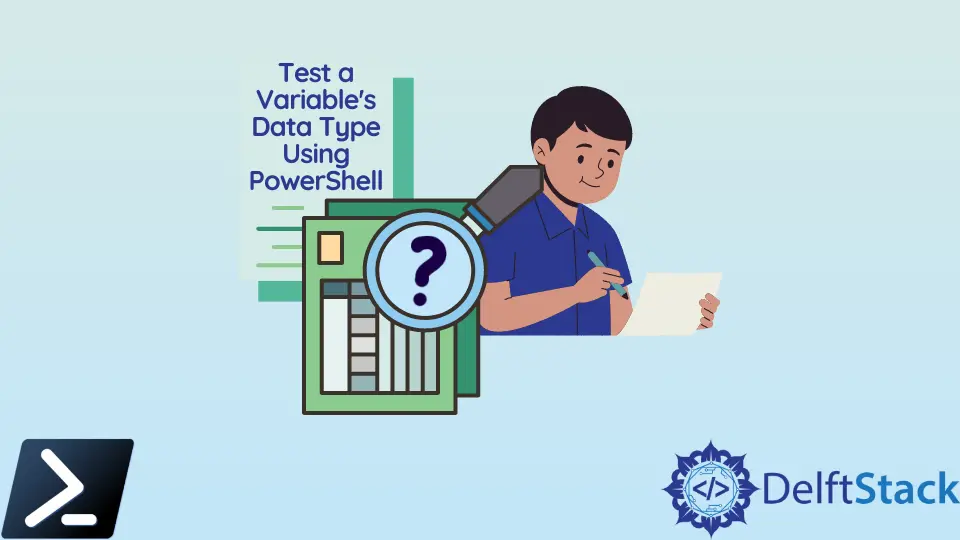
One technique in PowerShell we usually use is taking user input and storing it in a variable. However, there will be situations where a user input taken from the user is different from our expected data type.
One example would be a user who has entered his name, a String
data type, instead of his age which is an Int
or integer data type. In this article, we will discuss several ways how we can handle variable data type mismatches and how we can adequately correct them using PowerShell.
Test a Variable Using PowerShell
We will show you three examples of handling variable data type mismatches. Let’s start with using parameters.
Test a Variable Using Parameters
One way of asking for user input is by pre-configuring mandatory parameters during script execution. When pre-determining parameters, we can add a data type to support the parameter.
This way, the script can tell if the user is passing valid variables before the script begins. Let’s look at the example.
Let’s say we need the age of the user running the script. We can run the following script below to force the script only to accept integer values.
Example Code (param.ps1):
param(
[Parameter(Mandatory)]
[Int]$age
)
Write-Output $age
Suppose the user has executed it with .\param.ps1 -age 2
, the script should return it with the output of the number.
Input:
Supply values for the following parameters:
age: 2
Output:
2
However, if the user tries passing a different value with a data type aside from an integer, for example, .\param.ps1 -age "hello"
, the script will throw an error.
Input:
Supply values for the following parameters:
age: hello
Output:
"System.Int32". Error: "Input string was not in a correct format."
Perhaps we are processing a user input during the script execution. The following methods will check the variable’s data type while the script execution is in progress.
Test a Variable With Operators
Another method of checking a variable’s data type is the use of conditional operators. Conditional operators are functions that match two or more values together that produce a Boolean variable.
We will use the conditional operators -is
for this article. In this situation, we can use the -is
operator by matching our variable directly to the data type.
Example Code:
$age -is [Int]
Output:
True
For example, with our snippet of code below, the script will output the value of True
if the variable has an integer value. Otherwise, False
. Since these outputs export a Boolean value, we can use conditional statements to do specific actions, like, perhaps, converting a variable’s data type with the -as
operator.
Example Code:
if ($age -is [Int]) {
Write-Output "Age $age is an integer"
}
else {
Write-Output "Age is not an integer... Converting"
try {
$age = $age -as [Int]
Write-Output "Converted! Age $age is now an integer"
}
catch {
Write-Output "Input is a string, could not be converted."
}
}
Output:
Age is not an integer... Converting
Converted! Age 2 is now an integer
Test a Variable With Functions
Our last example is similar to our previous one because we will use operators to create our custom function. We can use these operators to create a function that will check for the variable’s data type and return a Boolean value.
What’s great about the function below is that compared to our previous example, this function will also check for values that are also negative, providing us with more accurate results.
Example Code:
function isNumeric($_) {
return $_ -is [int] -or $_ -is [int16] -or $_ -is [int32] -or $_ -is [int64]
}
isNumeric(-1)
Output:
True
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn