使用 PowerShell 测试变量的数据类型
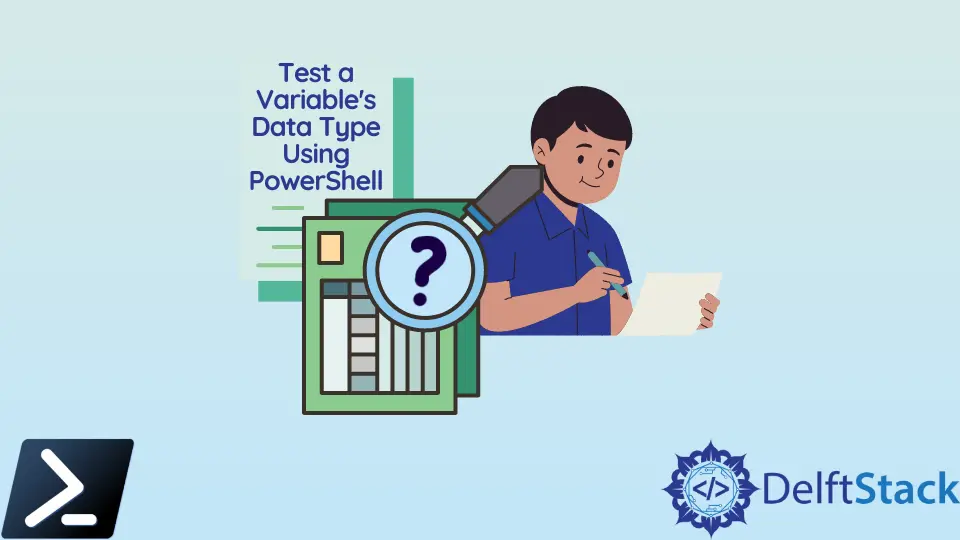
我们通常使用的 PowerShell 中的一种技术是获取用户输入并将其存储在变量中。但是,在某些情况下,从用户那里获取的用户输入与我们预期的数据类型不同。
一个例子是一个用户输入了他的名字,一个 String
数据类型,而不是他的年龄,它是一个 Int
或整数数据类型。在本文中,我们将讨论如何处理可变数据类型不匹配的几种方法,以及如何使用 PowerShell 充分纠正它们。
使用 PowerShell 测试变量
我们将向你展示处理可变数据类型不匹配的三个示例。让我们从使用参数开始。
使用参数测试变量
要求用户输入的一种方法是在脚本执行期间预先配置强制参数。在预先确定参数时,我们可以添加一个数据类型来支持该参数。
这样,脚本可以在脚本开始之前判断用户是否传递了有效变量。让我们看一下这个例子。
假设我们需要运行脚本的用户的年龄。我们可以运行下面的脚本来强制脚本只接受整数值。
示例代码(param.ps1):
param(
[Parameter(Mandatory)]
[Int]$age
)
Write-Output $age
假设用户已经使用 .\param.ps1 -age 2
执行了它,脚本应该将它与数字的输出一起返回。
输入:
Supply values for the following parameters:
age: 2
输出:
2
但是,如果用户尝试使用除整数之外的数据类型传递不同的值,例如 .\param.ps1 -age "hello"
,脚本将抛出错误。
输入:
Supply values for the following parameters:
age: hello
输出:
"System.Int32". Error: "Input string was not in a correct format."
也许我们正在脚本执行期间处理用户输入。以下方法将在脚本执行过程中检查变量的数据类型。
使用运算符测试变量
检查变量数据类型的另一种方法是使用条件运算符。条件运算符是将两个或多个值匹配在一起产生布尔变量的函数。
我们将在本文中使用条件运算符 -is
。在这种情况下,我们可以通过将变量直接与数据类型匹配来使用 -is
运算符。
示例代码:
$age -is [Int]
输出:
True
例如,使用下面的代码片段,如果变量具有整数值,脚本将输出 True
的值。否则,假
。由于这些输出导出一个布尔值,我们可以使用条件语句来执行特定的操作,例如,也许使用 -as
运算符转换变量的数据类型。
示例代码:
if($age -is [Int]){
Write-Output "Age $age is an integer"
}else{
Write-Output "Age is not an integer... Converting"
try{
$age = $age -as [Int]
Write-Output "Converted! Age $age is now an integer"
}catch{
Write-Output "Input is a string, could not be converted."
}
}
输出:
Age is not an integer... Converting
Converted! Age 2 is now an integer
用函数测试变量
我们的最后一个示例与上一个示例类似,因为我们将使用运算符来创建我们的自定义函数。我们可以使用这些运算符创建一个函数,该函数将检查变量的数据类型并返回一个布尔值。
下面这个函数的好处在于,与我们之前的例子相比,这个函数还会检查是否为负值,为我们提供更准确的结果。
示例代码:
function isNumeric($_){
return $_ -is [int] -or $_ -is [int16] -or $_ -is [int32] -or $_ -is [int64]
}
isNumeric(-1)
输出:
True
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn