Pipeline Variable in PowerShell
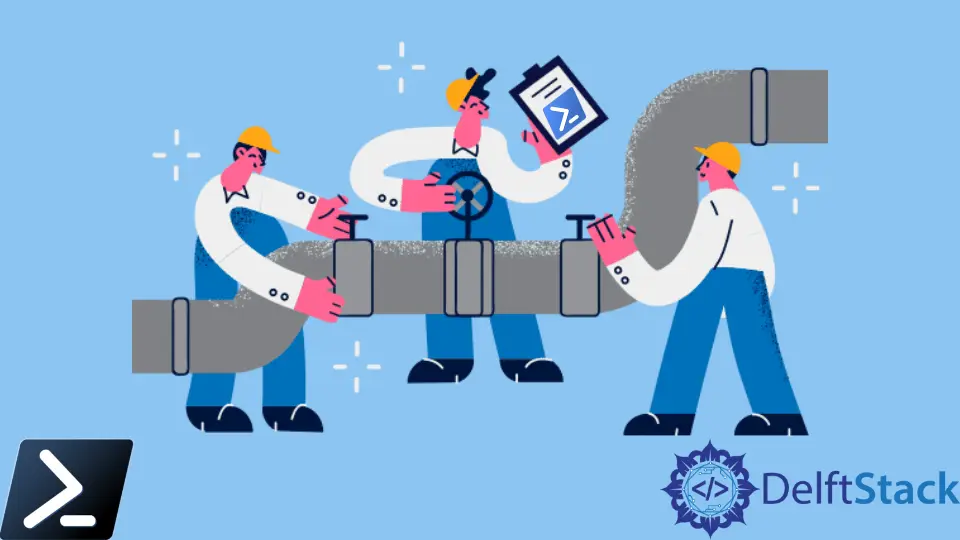
We usually use the this
variable or keyword to signify the last object presented in an expression in various programming languages. This common method of writing scripts is handy when we want to write scripts seamlessly.
This article will show us how to use the same concepts and techniques in Windows PowerShell by using PowerShell’s pipeline
variable.
Understanding the Pipeline Variable $_
or $PSItem
in PowerShell
Windows PowerShell has a unique pipeline variable $_
or $PSItem
. Windows PowerShell’s ability to pipe entire objects from one command to another is needed to represent that object traversing the pipeline.
When one Windows PowerShell cmdlet pipes something to another command, that cmdlet can send an object.
An object can be of any type. That object has a set of methods and properties attached to it.
We can reference those techniques and properties on a standalone object by creating the object itself, assigning it to a newly declared variable, and then referencing its properties and methods that way.
For example, when querying a directory, the Get-Item
cmdlet returns a System.IO.DirectoryInfo
object.
Get-Item -Path 'C:\Windows' | Get-Member
This object then has lots of different techniques and properties we can reference. So, for example, we could call methods on this object or reference its properties.
$windowsDir.GetFiles()
But, what if we are using a cmdlet like Get-ChildItem
, which enumerates lots of directories at once? It would be challenging to assign variables like $dir1
, dir2
, and $dir3
.
We need a more dynamic way to set them in a single variable representing each directory object upon processing it. So, we will need to use the pipeline
variable in this use case.
Using the Get-ChildItem
example, let us say we want to find all folders and subfolders in the C:\Windows
directory using Get-ChildItem -Path C:\Windows\ -Directory
.
This cmdlet returns a few dozen directories. If we assigned this output to a variable, it would be an array of objects, not just a single object.
So instead, we can pipe all of those objects, one at a time, from Get-ChildItem
cmdlet to ForEach-Object
cmdlet and call the pipeline variable.
The pipeline variable $_
or $PSItem
references each object upon processing it.
For example, below, we can see that we can reference the Name
property for every directory processed using the $_.Name
variable.
Get-ChildItem -Path C:\Windows\ -Directory | ForEach-Object { $_.Name }
Output:
addins
ADFS
appcompat
AppPatch
AppReadiness
assembly
With the pipeline variable, we can represent any method and property. The variable will always be the same type as the object from the previous command.
For example, we can see below that if you process the pipeline variable itself to Get-Member
, it returns the same object type as Get-Item
.
Get-ChildItem -Path C:\Windows\ -Directory | ForEach-Object { $_ | Get-Member } | Select-Object -First 1
Output:
TypeName: System.IO.DirectoryInfo
Name MemberType Definition
---- ---------- ----------
LinkType CodeProperty System.String LinkType{get=GetLinkType;}
We will see the pipeline variable most often used in commands that reference or perform specific actions on each object processed. Another example is using the Select-Object
command’s calculated properties.
This method allows the scripter to manipulate the output returned by representing objects from the pipeline.
For example, instead of using the ForEach-Object
command to return only the directory names, we could use a calculated property to create a DirectoryName
property on the fly and assign the directory names to that.
Get-ChildItem -Path C:\Windows\ -Directory | Select-Object -Property @{ Name = 'DirectoryName'; Expression = { $_.Name } }
Output:
DirectoryName
-------------
addins
ADFS
appcompat
AppPatch
AppReadiness
assembly
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn