How to Copy Files and Folders in PowerShell
-
Introduction to
Copy-Item
Command in PowerShell -
Use
Copy-Item
Command to Copy Specific Files in PowerShell -
Use
Copy-Item
Command to Merge Multiple Folders in PowerShell -
Use
Copy-Item
Command to Copy Files Recursively in PowerShell -
Use
Copy-Item
Command to Copy Files Using PowerShell Remoting
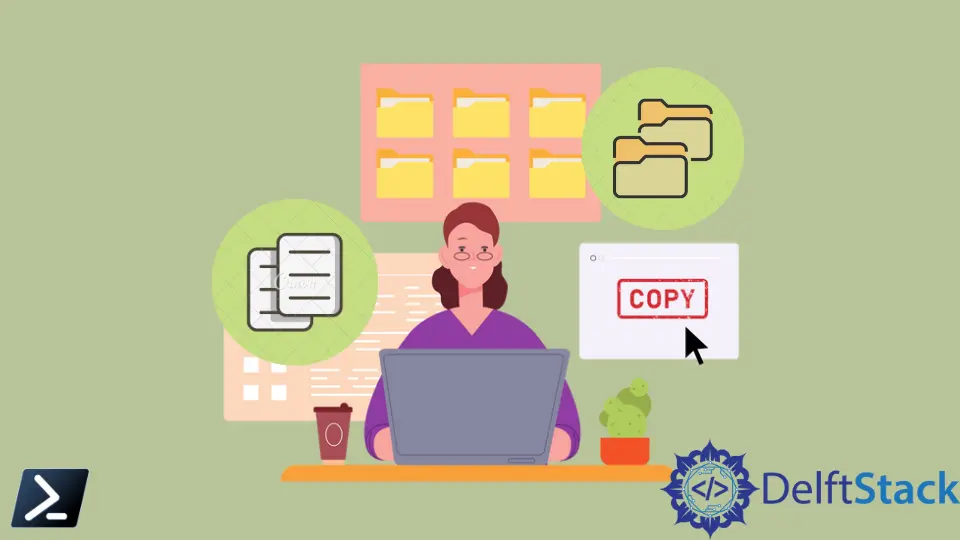
Several commands for copying files have been around in all programming languages. However, in PowerShell, the most popular way to get a copy of a file or folder in your PowerShell script from location A to location B is by using the PowerShell Copy-Item
cmdlet.
This article will discuss how to copy a folder and file while giving us the ability to recurse files in a folder, using wildcards to select the files we need to copy and Windows PowerShell Remoting for a file copy.
Introduction to Copy-Item
Command in PowerShell
The Copy-Item
command is a part of the Windows PowerShell provider cmdlets. It’s a generic cmdlet recognized by its Item
noun.
Using the Copy-Item
cmdlet, PowerShell allows a developer to copy folders and files in many different ways.
The Copy-Item
command copies a single file from one location to another using the -Path
parameter as the source file path and the -Destination
parameter as the destination folder path.
Copy-Item -Path C:\Temp\File1.txt -Destination C:\PS\
This cmdlet can also copy folders.
Copy-Item -Path C:\Temp\Scripts -Destination C:\PS\
For example, there is a read-only file in the folder. By default, Copy-Item
will not overwrite it.
Add the -Force
parameter in your code snippet to force the override.
Copy-Item -Path C:\Temp\Scripts -Destination C:\PS\ -Force
Use Copy-Item
Command to Copy Specific Files in PowerShell
In addition to copying a single folder or file, we can also copy the entire contents. The -Path
parameter of the Copy-Item
command accepts wildcard characters like the asterisk (*
) to match zero to multiple characters or the question mark (?
) to only match a single character.
Copy-Item -Path C:\Temp\*.ps1 -Destination C:\PS\
Copy-Item -Path 'C:\Temp\File?.txt' -Destination C:\PS\
Use Copy-Item
Command to Merge Multiple Folders in PowerShell
Another feature of Copy-Item
is copying multiple folders together simultaneously. In addition, we can pass various paths to the -Path
parameter.
The Copy-Item
will look at each one, copy either the folder or file(s) depending on the path, and merge them all into a single destination.
Copy-Item -Path C:\Temp\*, C:\Scripts\*, C:\Docs\* -Destination C:\PS
Use Copy-Item
Command to Copy Files Recursively in PowerShell
We usually run into situations where we have many subfolders in the parent folder, which files we’d like to copy over. Using the -Recurse
parameter on Copy-Item
will look in each subfolder and copy all files and folders in each recursively.
Copy-Item -Path C:\Temp\ -Destination C:\PS -Recurse
Use Copy-Item
Command to Copy Files Using PowerShell Remoting
One feature of PowerShell version 5 is this command’s ability to use WinRM and a PowerShell remote session. So, for example, Copy-Item
uses an existing PowerShell session and transfers the -Session
parameter files.
This is a great method to get around firewalls and an extra layer of security when the session communication is encrypted.
$session = New-PSSession -ComputerName WIN-FS01
Copy-Item -Path C:\Temp\File1.txt -ToSession $session -Destination 'C:\Temp'
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn