Copy-Item Excluding Folder in PowerShell
-
Excluding Folders in PowerShell Copy Operations Using the
Get-Item
Function and-Exclude
Parameter -
Excluding Folders in PowerShell Copy Operations Using
Where-Object
andPSIsContainer
- Conclusion
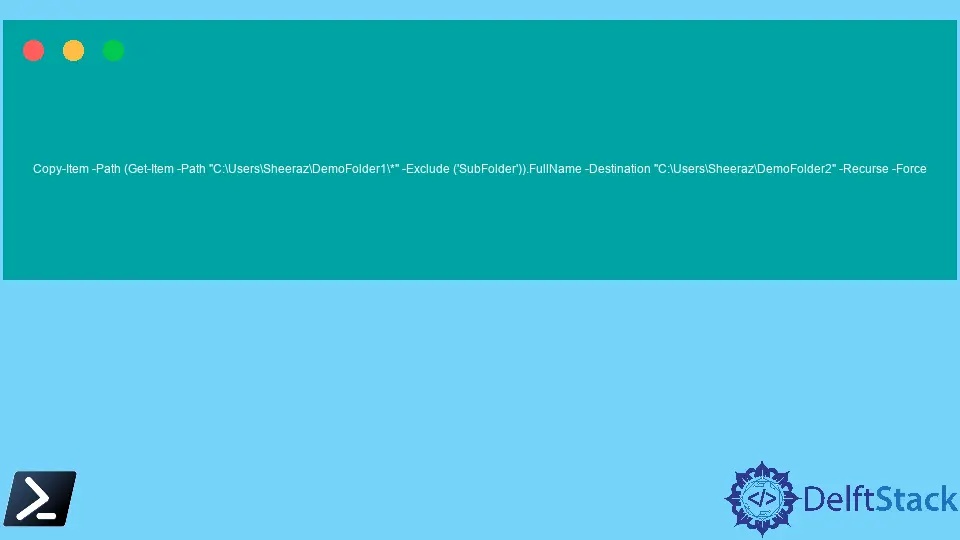
In the realm of file management and automation using PowerShell, the task of copying files while excluding specific folders is a common yet nuanced challenge.
This article delves into two effective methods to accomplish this task: using the Get-Item
function with the -Exclude
parameter and leveraging Where-Object
with the PSIsContainer
property.
Both methods, while serving the same purpose, approach the problem from different angles and offer unique advantages.
Our goal is to provide a comprehensive understanding of these methods, their applications, and the nuances of their usage. Through detailed explanations, syntax breakdowns, and practical examples, we aim to equip both new and experienced PowerShell users with the skills to efficiently manage file copying tasks, customizing their approaches to fit the specific requirements of their workflows.
Excluding Folders in PowerShell Copy Operations Using the Get-Item
Function and -Exclude
Parameter
We can use the Copy-Item
command to copy the files while excluding a folder in PowerShell. There are two methods to use Copy-Item
while excluding a folder, one is excluding a single folder, and the other is excluding multiple folders.
The syntax for this operation combines Copy-Item
with Get-Item -Exclude
:
Copy-Item -Path (Get-Item -Path "sourcePath\*" -Exclude ('folderToExclude')).FullName -Destination "destinationPath" -Recurse -Force
Parameters:
Copy-Item
: This cmdlet copies files and directories to a new location.-Path
: Specifies the path of the items to copy. In our case, this is provided byGet-Item
.Get-Item
: Retrieves the items from the specified location.-Exclude
: This parameter inGet-Item
filters out items, particularly the names of folders or files to exclude.('folderToExclude')
: The folder name you want to exclude..FullName
: Retrieves the full path of the items.-Destination
: The path to copy the items to.-Recurse
: Includes the contents of subdirectories.-Force
: Forces the command to run without asking for user confirmation.
Code Example:
Copy-Item -Path (Get-Item -Path "origin\*" -Exclude ('SubFolder')).FullName -Destination "destination\" -Recurse -Force
In our script, we begin by targeting the source directory (C:\origin\
) and employ Get-Item
to retrieve all the items it contains. The use of the asterisk (*
) symbol here acts as a wildcard, representing every item within the directory.
We then leverage the -Exclude
parameter to articulate our intention to exclude a specific subfolder, notably named SubFolder
. The employment of the .FullName
property is critical in this context as it fetches the complete path for each item we are dealing with.
After curating this list, which pointedly omits the designated folder, it is handed over to Copy-Item
. We proceed to define our target location as the destination directory (C:\destination
). To ensure a comprehensive copying process, encompassing all subdirectories bar the one we’ve opted to exclude, we incorporate the -Recurse
parameter.
To smoothen our process further and avert potential interruptions, the -Force
parameter comes into play. It effectively suppresses any system prompts that might otherwise pause the operation, particularly those associated with overwriting existing files in the destination directory.
origin
folder:
Output:
The above command will only exclude one given folder. We can also exclude multiple folders by just putting the multiple folder names in -Exclude()
.
See the command for excluding multiple folders:
Copy-Item -Path (Get-Item -Path "origin\*" -Exclude ('SubFolder', 'SubFolder2')).FullName -Destination "destination\" -Recurse -Force
See the output:
Excluding Folders in PowerShell Copy Operations Using Where-Object
and PSIsContainer
PowerShell provides an extensive set of cmdlets for file and folder manipulation, with Copy-Item
being a key player for copying operations. However, its native functionality doesn’t directly support the exclusion of folders.
To achieve this, we employ a combination of the Get-ChildItem
, Where-Object
, and the PSIsContainer
property. This method is particularly useful for copying operations where you need to exclude directories while preserving files.
The script’s structure is as follows:
Get-ChildItem -Path "sourcePath\" -Recurse | Where-Object { $_.PSIsContainer -notmatch 'True' } | Copy-Item -Destination "destinationPath" -Recurse -Container
Parameters:
Get-ChildItem -Path "sourcePath\" -Recurse
: Retrieves all items (files and folders) from the specified path, including subdirectories.Where-Object { $_.PSIsContainer -notmatch 'True' }
: Filters out all directories.PSIsContainer
is a boolean property that isTrue
for directories andFalse
for files.Copy-Item -Destination "destinationPath" -Recurse -Container
: Copies the remaining items (files) to the destination.-Recurse
is used to ensure subdirectory traversal, and-Container
preserves the directory structure.
Code Example:
Get-ChildItem -Path "origin\" -Recurse | Where-Object { $_.PSIsContainer -notmatch 'True' } | Copy-Item -Destination "destination\" -Recurse -Container
In our script, we begin by collecting all items from the source directory (origin\
). To do this, we employ the Get-ChildItem
cmdlet.
The real magic happens with the Where-Object
cmdlet, where we introduce a filter to specifically exclude directories. This exclusion is based on the $_ .PSIsContainer -notmatch 'True'
condition.
This particular piece of code is crucial as it effectively sifts through the gathered items, ensuring that only files (and not directories) are passed down the pipeline.
Next, these filtered files are handed over to the Copy-Item
cmdlet. Our command here is to copy these files to our specified destination (destination\
).
An important aspect of this operation is how it preserves the original directory structure in the destination despite excluding the folders themselves. This balance is achieved through the careful orchestration of the -Recurse
and -Container
parameters within Copy-Item
, ensuring that the integrity of the file structure is maintained, minus the actual folders.
Output:
Conclusion
In conclusion, the techniques presented in this article — using Get-Item
with -Exclude
and Where-Object
with PSIsContainer
— offer powerful and flexible ways to exclude folders during PowerShell copy operations. By understanding and applying these methods, users can significantly enhance their file management and automation capabilities in PowerShell.
The Get-Item
and -Exclude
approach is straightforward and highly effective for excluding specific folders, while the Where-Object
and PSIsContainer
methods provide a more nuanced control, especially useful in scenarios where the exclusion criteria are more complex or dynamic. Both methods demonstrate PowerShell’s versatility and power in handling files and directories, making it an invaluable tool for system administrators, developers, and IT professionals.
Armed with this knowledge, users can confidently tackle a wide array of file copying tasks, ensuring efficient and precise control over their file management operations in Windows environments.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook