How to Use the CmdletBinding Attribute in PowerShell
-
CmdletBinding
Attribute in PowerShell -
Use the
CmdletBinding
Attribute With theVerbose
Parameter -
Use the
CmdletBinding
Attribute With the$PSCmdlet
Object andSupportsShouldProcess
-
Use the
CmdletBinding
Attribute With theParameter
Attribute to Control Function Parameters
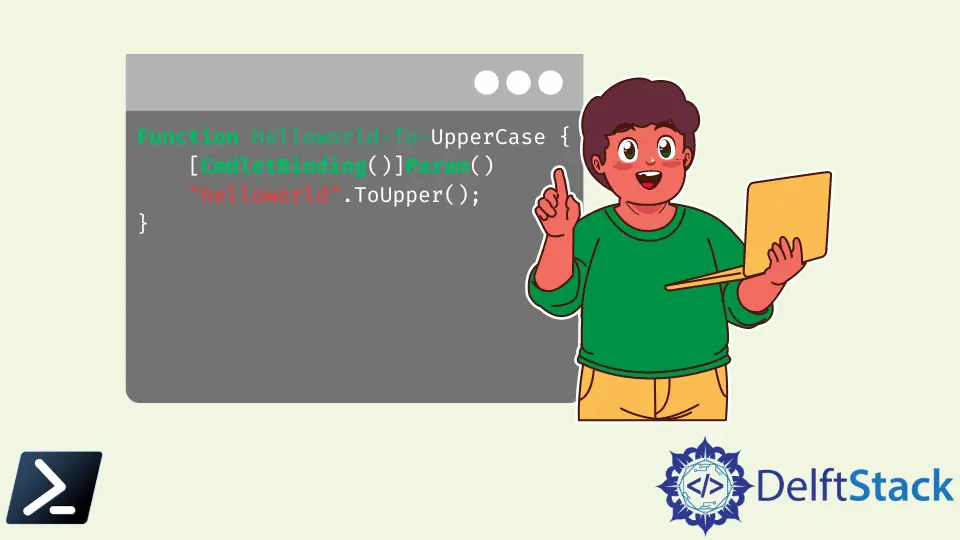
The cmdlet
is a lightweight script that performs a single function within the PowerShell environment. The cmdlets
can be written in any .Net
language.
Usually, a cmdlet
is expressed as a verb-noun pair to execute a command. The command is an order to the underlying operating system to perform a specific service by the end-user.
The PowerShell environment includes more than 200 basic cmdlets
such as New-Item
, Move-Item
, Set-Location
, and Get-Location
. The cmdlet
share a common set of features not available in simple PowerShell functions.
- Supports common parameters such as
-WhatIf
,ErrorAction
,Verbose
, etc. - Prompt for confirmations
- Mandatory parameter support
CmdletBinding
Attribute in PowerShell
The simple PowerShell function can be written as an advanced function by inheriting the basic cmdlet
features discussed above. The CmdletBinding
attribute enables you to access these basic cmdlet
features.
The following shows the CmdletBinding
attribute syntax with all the possible arguments.
{
[CmdletBinding(ConfirmImpact = <String>,
DefaultParameterSetName = <String>,
HelpURI = <URI>,
SupportsPaging = <Boolean>,
SupportsShouldProcess = <Boolean>,
PositionalBinding = <Boolean>)]
Param ($myparam)
Begin {}
Process {}
End {}
}
Let’s say we have a simple PowerShell function called Helloworld-To-UpperCase
.
Function Helloworld-To-UpperCase {
"helloworld".ToUpper();
}
There are no parameters attached to this function. So, this is called a simple PowerShell function.
But we can use the CmdletBinding
attribute to convert this function to an advanced one and access the basic cmdlet
features and parameters, as shown in the following.
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
"helloworld".ToUpper();
}
The Helloworld-To-UpperCase
function has been converted to an advanced function that inherits all the basic cmdlet
features. The basic cmdlet
parameters are available to this function.
If you call this function with -
in the PowerShell window, it should list all the common parameters coming from the cmdlet
.
helloworld-to-uppercase -
Output:
The common cmdlet
parameters and features can be used within our advanced functions to extend the functionality.
Use the CmdletBinding
Attribute With the Verbose
Parameter
The -verbose
is one of the valuable common parameters that can display a message when the advanced function is executed.
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
"helloworld".ToUpper();
}
When we call the above function with the -verbose
parameter, it will print all the Write-Verbose
strings to the PowerShell window.
HelloWorld-To-UpperCase -Verbose
Output:
Use the CmdletBinding
Attribute With the $PSCmdlet
Object and SupportsShouldProcess
Since we have used the CmdletBinding
attribute, our advanced function can access the $PSCmdlet
object without hassle. This object contains several methods such as ShouldContinue
, ShouldProcess
, ToString
, WriteDebug
, etc.
Use the CmdletBinding
Attribute With the ShouldContinue
Method
This method allows users to handle the confirmation requests. In the meantime, it is mandatory to set the SupportsShouldProcess
argument to $True
.
The ShouldContinue
method got several overloaded methods, and we will use the one with two parameters.
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
"helloworld".ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
We can call the function with the -Confirm
parameter, and it will display a confirmation box as shown in the following.
HelloWorld-To-UpperCase -Confirm
Output:
If the user clicks on Yes
, it should implement the method in the if
block and print the helloworld
string in uppercase letters.
If not, it should show the helloworld kept in lowercase
message.
Use the CmdletBinding
Attribute With the Parameter
Attribute to Control Function Parameters
Let’s make our advanced function take one parameter as a string.
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param([string]$word)
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
We have changed the Helloworld-To-UpperCase
function to take one string type parameter called $word
. When the function has called, we need to provide a string as an argument.
The provided text will convert to uppercase. If the user hasn’t provided any text argument, the function will give an empty output.
We can control this by making the $word
parameter mandatory and giving parameter position 0
.
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param(
[Parameter(
Mandatory = $True, Position = 0
) ]
[string]$word
)
#Verbose
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
#If/Else block for request processing
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
We have added some flags to control the behavior of the $word
parameter. Since it is mandatory, we need to provide a string value when the function executes.
HelloWorld-To-UpperCase -Confirm "stringtouppercase"
PowerShell keeps asking for that if we don’t provide the text argument.
You can use several flags to control the parameters in your functions, as shown in the following Parameter
attribute syntax.
Param
(
[Parameter(
Mandatory = <Boolean>,
Position = <Integer>,
ParameterSetName = <String>,
ValueFromPipeline = <Boolean>,
ValueFromPipelineByPropertyName = <Boolean>,
ValueFromRemainingArguments = <Boolean>,
HelpMessage = <String>,
)]
[string[]]
$Parameter1
)
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.