如何在 PowerShell 中使用 CmdletBinding 属性
-
PowerShell 中的
CmdletBinding
属性 -
使用
CmdletBinding
属性与Verbose
参数 -
使用
CmdletBinding
属性与$PSCmdlet
对象和SupportsShouldProcess
-
使用
CmdletBinding
属性与Parameter
属性控制函数参数
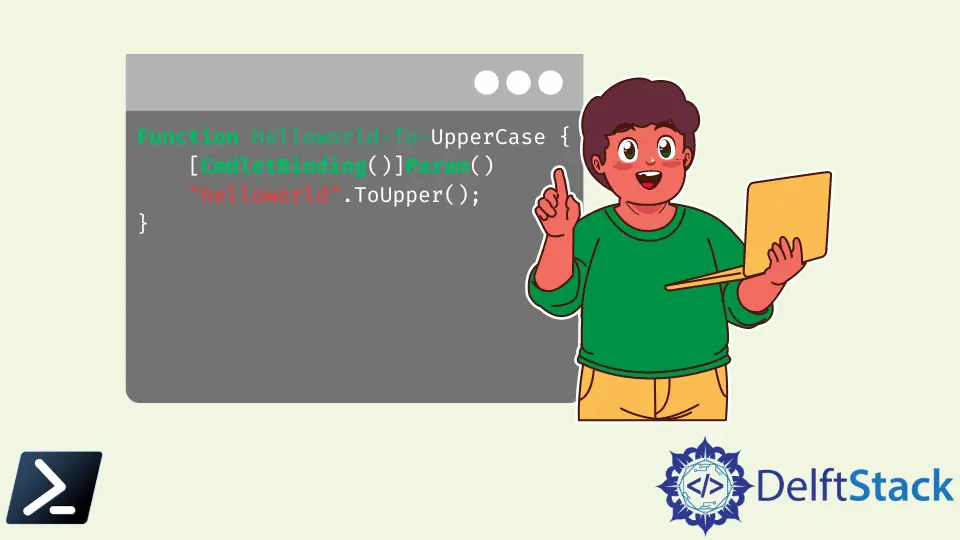
cmdlet
是一个轻量级脚本,在 PowerShell 环境中执行单个功能。cmdlets
可以用任何 .Net
语言编写。
通常,cmdlet
表达为动词-名词对以执行命令。该命令是向底层操作系统下达的指令,以由最终用户执行特定服务。
PowerShell 环境包括 200 多个基本 cmdlets
,例如 New-Item
、Move-Item
、Set-Location
和 Get-Location
。cmdlet
具有简单 PowerShell 函数中不具备的共同特性。
- 支持普通参数,如
-WhatIf
、ErrorAction
、Verbose
等。 - 提示确认
- 强制参数支持
PowerShell 中的 CmdletBinding
属性
简单 PowerShell 函数可以通过继承上述基本 cmdlet
特性,写成高级函数。CmdletBinding
属性使您可以访问这些基本 cmdlet
特性。
以下显示了 CmdletBinding
属性语法及所有可能的参数。
{
[CmdletBinding(ConfirmImpact = <String>,
DefaultParameterSetName = <String>,
HelpURI = <URI>,
SupportsPaging = <Boolean>,
SupportsShouldProcess = <Boolean>,
PositionalBinding = <Boolean>)]
Param ($myparam)
Begin {}
Process {}
End {}
}
假设我们有一个名为 Helloworld-To-UpperCase
的简单 PowerShell 函数。
Function Helloworld-To-UpperCase {
"helloworld".ToUpper();
}
此函数没有附加参数。因此,它被称为简单 PowerShell 函数。
但是,我们可以使用 CmdletBinding
属性将此函数转换为高级函数,并访问基本 cmdlet
特性和参数,如下所示。
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
"helloworld".ToUpper();
}
当我们使用 -verbose
参数调用上述函数时,它将把所有 Write-Verbose
字符串打印到 PowerShell 窗口。
HelloWorld-To-UpperCase -Verbose
输出:
使用 CmdletBinding
属性与 $PSCmdlet
对象和 SupportsShouldProcess
由于我们使用了 CmdletBinding
属性,我们的高级函数可以毫无麻烦地访问 $PSCmdlet
对象。该对象包含多个方法,如 ShouldContinue
、ShouldProcess
、ToString
、WriteDebug
等。
使用 CmdletBinding
属性与 ShouldContinue
方法
此方法允许用户处理确认请求。同时,必须将 SupportsShouldProcess
参数设置为 $True
。
ShouldContinue
方法有多种重载方法,我们将使用带有两个参数的那一个。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
"helloworld".ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
我们可以使用 -Confirm
参数调用该函数,它将显示一个确认框,如下所示。
HelloWorld-To-UpperCase -Confirm
输出:
如果用户点击 Yes
,它应该在 if
块中执行该方法,并按大写字母打印 helloworld
字符串。
如果没有,它应该显示 helloworld kept in lowercase
消息。
使用 CmdletBinding
属性与 Parameter
属性控制函数参数
让我们使我们的高级函数接受一个字符串参数。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param([string]$word)
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
我们已将 Helloworld-To-UpperCase
函数更改为接受一个名为 $word
的字符串类型参数。当函数被调用时,我们需要提供一个字符串作为参数。
提供的文本将转换为大写。如果用户没有提供任何文本参数,函数将给出空输出。
我们可以通过使 $word
参数强制并给出参数位置 0
来控制这一点。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param(
[Parameter(
Mandatory = $True, Position = 0
) ]
[string]$word
)
#Verbose
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
#If/Else block for request processing
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
我们添加了一些标志来控制 $word
参数的行为。既然它是强制性的,函数执行时我们需要提供字符串值。
HelloWorld-To-UpperCase -Confirm "stringtouppercase"
如果我们不提供文本参数,PowerShell 会不断询问。
您可以使用几个标志来控制函数中的参数,如下所示的 Parameter
属性语法。
Param
(
[Parameter(
Mandatory = <Boolean>,
Position = <Integer>,
ParameterSetName = <String>,
ValueFromPipeline = <Boolean>,
ValueFromPipelineByPropertyName = <Boolean>,
ValueFromRemainingArguments = <Boolean>,
HelpMessage = <String>,
)]
[string[]]
$Parameter1
)
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.