How to Exit From Foreach Object in PowerShell
-
Use the
break
Condition to Exit FromForEach-Object
in PowerShell -
Use the
if
andbreak
to Exit FromForEach-Object
in PowerShell -
Use the
if
andreturn
to Exit FromForEach-Object
in PowerShell -
Use the
if
andcontinue
to Exit FromForEach-Object
in PowerShell - Conclusion
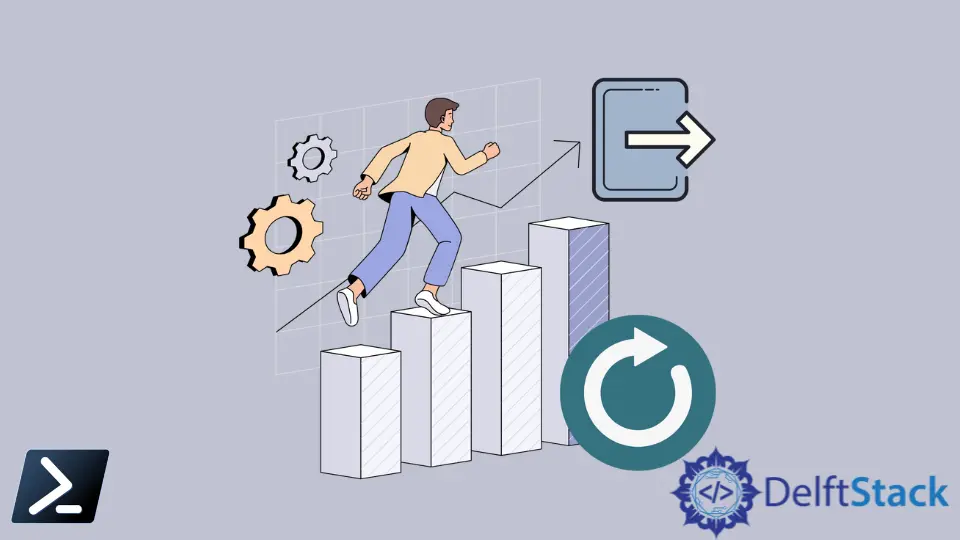
The ForEach-Object
cmdlet allows the user to iterate through collections and operate against each item in a collection of input objects. In ForEach-Object
, the input objects are piped to the cmdlet or specified using the -InputObject
parameter.
There are two different ways of constructing a ForEach-Object
command in PowerShell: Script block
and Operation statement
. The ForEach-Object
cmdlet runs each input object’s script block or operation statement.
Use a script block
to specify the operation. The $_
variable is used within the script block to represent the current input object.
The script block
can contain any PowerShell script. For example, the following command gets the value of each cmdlet’s Name
property, function, and aliases
installed on the computer.
Get-Command | ForEach-Object { $_.Name }
Another way to construct the ForEach-Object
command is to use the operation
statement. You can specify a property value or call a method with the operation statement.
Get-Command | ForEach-Object Name
Sometimes, there are situations when you might want to exit from the ForEach-Object
, but it works differently than the ForEach
statement. In ForEach-Object
, the statement is executed as soon as each object is produced.
In the ForEach
statement, all the objects are collected before the loop executes. The ForEach-Object
is a cmdlet, not an actual loop.
When you use break
or continue
to exit the loop, the whole script is terminated instead of skipping the statement after it. However, it is possible to exit from the ForEach-Object
object using some conditions in PowerShell.
Use the break
Condition to Exit From ForEach-Object
in PowerShell
In PowerShell, the foreach-object
loop is commonly used to iterate over each item in a collection or pipeline. Occasionally, there arises a need to exit this loop prematurely based on certain conditions.
The break
statement provides a straightforward way to achieve this by immediately terminating the loop when a specific condition is met.
$numbers = "one", "two", "three", "four", "five"
$Break = $False;
$numbers | Where-Object { $Break -eq $False } | ForEach-Object {
$Break = $_ -eq "three";
Write-Host "The number is $_.";
}
In this code snippet, we initialize an array $numbers
containing strings representing numbers. We also set $Break
to $False
initially.
Within the foreach-object
loop, we use Where-Object
to filter items based on the $Break
variable. If $Break
is $False
, the loop continues; otherwise, it stops.
Inside the loop, we check if the current item is equal to three
. If it is, we set $Break
to $True
, causing the loop to terminate.
Output:
Use the if
and break
to Exit From ForEach-Object
in PowerShell
At times, there’s a need to exit this loop prematurely based on specific conditions. The if
method offers a straightforward approach to achieving this task by evaluating a condition within the loop and triggering an exit when the condition is met.
In this method, you need to use an empty
value in a collection of objects to exit from ForEach-Object
in PowerShell. For example, you can use the if
condition to exit from ForEach-Object
.
$numbers = "one", "two", "three", "", "four"
$numbers | ForEach-Object {
if ($_ -eq "") {
break;
}
Write-Host "The number is $_."
}
In the provided code snippet, we initialize an array $numbers
containing strings representing numbers. Within the foreach-object
loop, we iterate over each item in the array.
Using the if
statement, we check if the current item $_
is an empty string. If the condition evaluates to true, we use break
to exit from the loop immediately.
Otherwise, the loop continues, and we output the current number using Write-Host
.
Output:
Use the if
and return
to Exit From ForEach-Object
in PowerShell
Exiting from a foreach-object
loop in PowerShell using the combination of the if
and return
methods allows for the premature termination of the loop based on specific conditions. This method is particularly useful when you need to halt the loop execution and return control back to the caller when a certain condition is met.
Within the loop, an if
statement is employed to evaluate the condition for exiting the loop. If the condition evaluates to true, the return
statement is executed, immediately ending the loop execution and returning control to the caller.
$numbers = "one", "two", "three", "four", "five"
$numbers | ForEach-Object {
if ($_ -eq "three") {
return
}
Write-Host "The number is $_."
}
In the provided code snippet, we initialize an array $numbers
containing strings representing numbers. Within the foreach-object
loop, we iterate over each item in the array.
Using the if
statement, we check if the current item $_
is equal to three
. If the condition evaluates to true, we use return
to exit from the loop immediately.
Otherwise, the loop continues, and we output the current number using Write-Host
.
Output:
Use the if
and continue
to Exit From ForEach-Object
in PowerShell
In PowerShell, the continue
statement is used to skip the rest of the current iteration of a loop and proceed to the next iteration. While primarily employed in loops to skip specific iterations, continue
can also be utilized to implement a form of loop exit within a foreach-object
loop.
By strategically placing the continue
statement within the loop and incorporating conditional logic, you can achieve early termination of the loop based on specific conditions.
$numbers = 1..10
$numbers | ForEach-Object {
if ($_ -eq 5) {
continue
}
Write-Host "Number: $_"
}
In the provided code example, we initialize an array $numbers
containing numbers from 1 to 10. Within the foreach-object
loop, we iterate over each number in the array.
Using the if
statement, we check if the current number is equal to 5. If the condition evaluates to true, the continue
statement is executed, causing the loop to skip the current iteration and proceed to the next one.
This effectively achieves the early termination of the loop when the specified condition is met.
Output:
Conclusion
To summarize, the ForEach-Object
cmdlet in PowerShell facilitates iteration through collections, allowing operations on each item individually. We explored various methods to exit prematurely from a ForEach-Object
loop, including using break
, return
, and continue
.
While break
and continue
provide direct control flow within the loop, return
offers an alternative approach to exit the loop and return to the caller. Each method has its own use cases and benefits, providing flexibility in script execution.
Moving forward, users can deepen their understanding by experimenting with these methods in different scenarios, exploring their nuances, and mastering their application in PowerShell scripting.