How to Add Properties to Objects in PowerShell
- Use Hashtable to Create Custom Objects in PowerShell
-
Use
Add-Member
to Add Properties to Objects in PowerShell -
Use
Properties.Add()
to Add Properties to Objects in PowerShell -
Use
Select-Object
to Add Properties to Objects in PowerShell - Conclusion
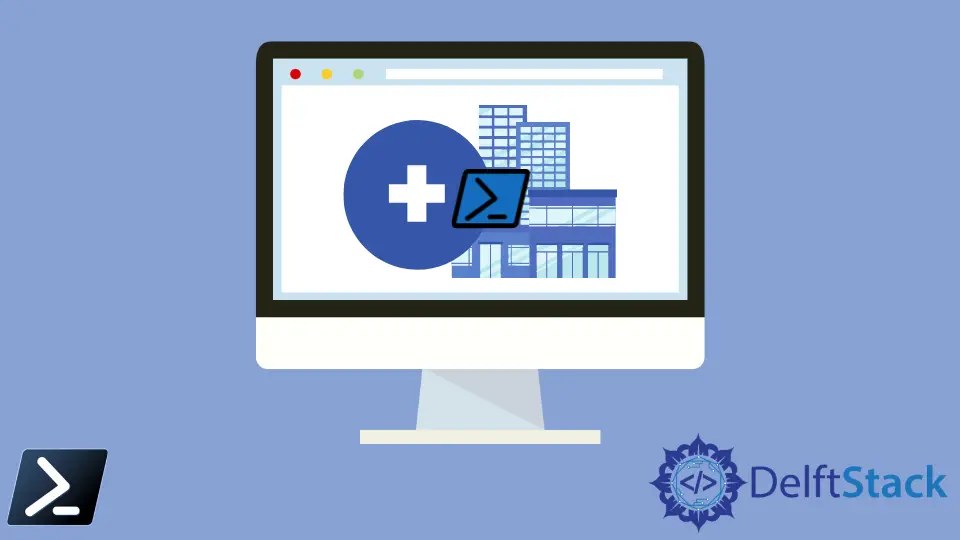
This article delves into various methods for adding properties to objects in PowerShell, an essential technique for PowerShell users ranging from beginners to experts. We begin by exploring the use of hashtables for creating custom objects, a straightforward yet powerful approach.
Following this, we introduce the Add-Member
cmdlet, a versatile tool for dynamically appending properties to existing objects. As we delve deeper, we uncover the intricacies of the Properties.Add()
method, offering a more nuanced and controlled way of enhancing objects.
Finally, we discuss using Select-Object
in combination with a hashtable, a method perfect for temporary modifications and preserving the original object’s state.
This comprehensive guide aims to provide practical insights and examples to empower PowerShell users to tailor objects to their specific needs.
Use Hashtable to Create Custom Objects in PowerShell
Before adding properties to an object, we must create one first. One way to do this is through hashtables.
To briefly define, hashtables are sets of keys or value pairs that we need to create and assign an object’s properties in PowerShell. In the example, we are creating a hashtable that represents a single object and its properties.
Once the hashtable, $sampleHashtable
, has been defined, we can then use the PSCustomObject
type accelerator to create an instance of the PSCustomObject
class.
After running the code snippet, we will obtain an object $sampleHashtable
of type PSCustomObject
with properties defined in the program.
Example 1:
$sampleObject = [PSCustomObject]@{
ID = 1
Shape = "Square"
Color = "Blue"
}
As we can see, after creating $sampleHashtable
, we will be able to reference each property as if it came from a built-in PowerShell cmdlet such as Get-Service
.
Use Add-Member
to Add Properties to Objects in PowerShell
PowerShell’s versatility as a scripting language is partly due to its ability to dynamically manipulate objects. A prime example of this capability is the Add-Member
cmdlet, which allows users to add properties and methods to objects at runtime.
The Add-Member
cmdlet is an essential tool in PowerShell for dynamically adding members, such as properties, methods, or events, to an instance of an object. This feature is especially useful for customizing objects to fit specific needs or for augmenting data structures with additional information.
Example:
$sampleObject | Add-Member -MemberType NoteProperty -Name 'Size' -Value 'Large'
Write-Output $sampleObject
In this example, we began by creating $sampleObject
, a custom PowerShell object with predefined properties. Our goal was to enhance this object by adding a new property.
Using the Add-Member
cmdlet, we appended a NoteProperty
named Size
with the value Large
to $sampleObject
. This process demonstrates the power of PowerShell in dynamically extending objects.
NoteProperty
was chosen because it’s suitable for static values, which was our requirement.
Lastly, we used Write-Host
to print the updated object, effectively demonstrating the addition of the new property.
Output:
Use Properties.Add()
to Add Properties to Objects in PowerShell
One lesser-known yet powerful method for enhancing objects is using the Properties.Add()
method. This approach is particularly useful when working with custom objects or when you need to modify objects in a more controlled manner.
The Properties.Add()
method is a way to add properties to an existing PowerShell object. This method is part of the underlying .NET
framework and provides a lower-level approach compared to the more commonly used Add-Member
cmdlet.
Example:
$sampleObject.psobject.Properties.Add([PSNoteProperty]::new('Size', 'Large'))
Write-Host ($sampleObject | Out-String)
In this example, we created $sampleObject
, an object with predefined properties.
Our goal was to enhance it by adding a new property named Size
. For this purpose, we utilized the .psobject.Properties.Add([PSNoteProperty]::new())
method.
This method is a bit more nuanced than Add-Member
. It involves creating a new PSNoteProperty
object directly and then adding it to the object’s property collection.
Finally, using Write-Host
, we output the updated object to confirm the addition of the new property.
Output:
Use Select-Object
to Add Properties to Objects in PowerShell
Another technique involves using Select-Object
combined with a hashtable (@{}
) to add properties to objects. This method is particularly useful for creating custom views or extending objects without modifying the original object.
The Select-Object *, @{}
method is used to create a new object by extending an existing one with additional properties. This approach is commonly used for temporary modifications of objects, such as for display purposes or when preparing data for export.
It’s non-destructive, meaning the original object remains unchanged.
Example:
$extendedObject = $sampleObject | Select-Object *, @{
Name = 'Size'
Expression = { 'Large' }
}
Write-Host ($extendedObject | Out-String)
In this example, we began with our $sampleObject
and set a goal to augment it with a new property named Size
. Our approach did not involve altering the original object, maintaining its integrity.
We achieved this by piping $sampleObject
into Select-Object
, effectively instructing PowerShell to carry over all existing properties (*
) and to introduce a new property defined by a hashtable.
In our hashtable, we clearly specified the new property’s name, Size
, and assigned its value, Large
, within an expression block. This approach is particularly elegant, as it constructs a new object, $extendedObject
, enriched with the additional property.
Output:
Conclusion
Throughout this article, we have journeyed through various methodologies for adding properties to objects in PowerShell, each with its unique advantages and applications. Starting with the creation of custom objects using hashtables, we laid the foundation for understanding object properties in PowerShell.
We then explored the Add-Member
cmdlet, a staple in PowerShell scripting for dynamically enhancing objects. The exploration of the Properties.Add()
method opened a window into a more in-depth approach, allowing for a controlled manipulation of object properties.
Lastly, the use of Select-Object
with a hashtable demonstrated a non-destructive and elegant way to extend objects. Each method, from the straightforward to the more complex, serves a unique purpose, offering PowerShell users a rich toolkit for customizing and managing objects in their scripts.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn