PHP Video Player
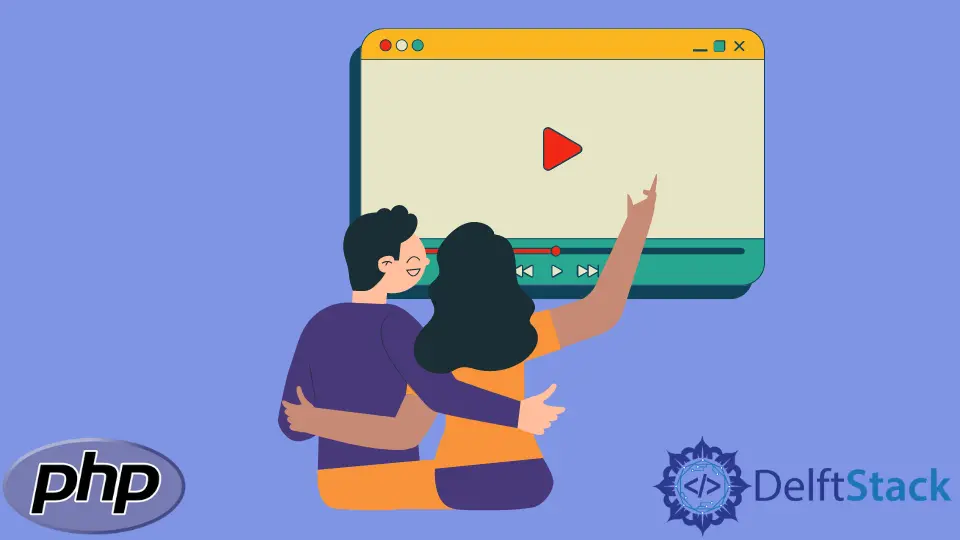
This tutorial demonstrates how to create a video player in PHP.
PHP Video Player
We can create a video player in PHP using the HTML streaming logic. We can use PHP to open and read the video file and send it for streaming.
Let’s jump to an example and then describe how it is working:
<?php
$Video_File = "C:\Users\Sheeraz\OneDrive\Desktop\New folder\sample.ogv";
if(!file_exists($Video_File)) return;
$File_Open = @fopen($Video_File, 'rb');
$File_Size = filesize($Video_File); // size of the video file
$Video_Length = $File_Size; // length of the video
$Video_Start = 0; // The start byte
$Video_End = $File_Size - 1; // The end byte
header('Content-type: video/mp4');
header("Accept-Ranges: 0-$Video_Length");
header("Accept-Ranges: bytes");
if (isset($_SERVER['HTTP_RANGE'])) {
$V_Start = $Video_Start;
$V_End = $Video_End;
list(, $Video_Range) = explode('=', $_SERVER['HTTP_RANGE'], 2);
if (strpos($Video_Range, ',') !== false) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
if ($Video_Range == '-') {
$V_Start = $File_Size - substr($Video_Range, 1);
}else{
$Video_Range = explode('-', $Video_Range);
$V_Start = $Video_Range[0];
$V_End = (isset($Video_Range[1]) && is_numeric($Video_Range[1])) ? $Video_Range[1] : $File_Size;
}
$V_End = ($V_End > $Video_End) ? $Video_End : $V_End;
if ($V_Start > $V_End || $V_Start > $File_Size - 1 || $V_End >= $File_Size) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
$Video_Start = $V_Start;
$Video_End = $V_End;
$Video_Length = $Video_End - $Video_Start + 1;
fseek($File_Open, $Video_Start);
header('HTTP/1.1 206 Partial Video Content');
}
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
header("Content-Length: ".$Video_Length);
$buffer = 1024 * 8;
while(!feof($File_Open) && ($p = ftell($File_Open)) <= $Video_End) {
if ($p + $buffer > $Video_End) {
$buffer = $Video_End - $p + 1;
}
set_time_limit(0);
echo fread($File_Open, $buffer);
ob_flush();
}
fclose($File_Open);
exit();
?>
The code above implements a video streaming player which can play the video on a browser like the HTML 5 streaming functionality. See the output:
Let’s try to describe how this video player is implemented in PHP:
-
First, the code tries to open the video file and then sets parameters for it, including video size, length, start byte, and end byte.
-
After that, the code tries to set the proper headers for the video. This is the most important step because we need to communicate with the browser using a browser.
-
In the headers step, we decide the default formats of the video and the range of videos that the browser will accept.
-
The
HTTP_RANGE
is used when the browser makes the range request. It will validate the range request and send data accordingly. -
The customized buffer is used to optimize the server memory utilization, so no huge memory is taken while streaming the video.
-
Finally, close the file and exit.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook