PHP ビデオ プレーヤー
Sheeraz Gul
2024年2月15日
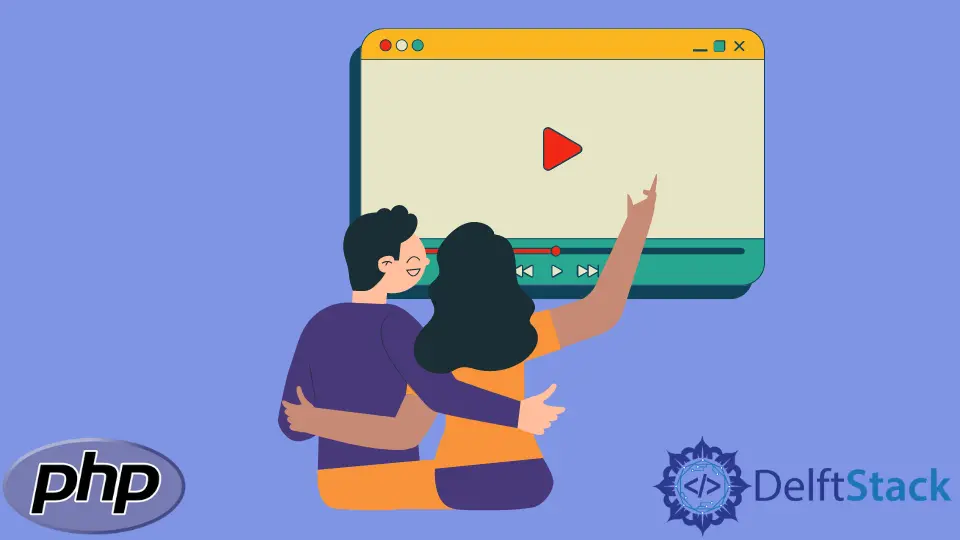
このチュートリアルでは、PHP でビデオ プレーヤーを作成する方法を示します。
PHP ビデオ プレーヤー
HTML ストリーミング ロジックを使用して、PHP でビデオ プレーヤーを作成できます。 PHP を使用して、ビデオ ファイルを開いて読み取り、ストリーミング用に送信できます。
例にジャンプして、それがどのように機能するかを説明しましょう。
<?php
$Video_File = "C:\Users\Sheeraz\OneDrive\Desktop\New folder\sample.ogv";
if(!file_exists($Video_File)) return;
$File_Open = @fopen($Video_File, 'rb');
$File_Size = filesize($Video_File); // size of the video file
$Video_Length = $File_Size; // length of the video
$Video_Start = 0; // The start byte
$Video_End = $File_Size - 1; // The end byte
header('Content-type: video/mp4');
header("Accept-Ranges: 0-$Video_Length");
header("Accept-Ranges: bytes");
if (isset($_SERVER['HTTP_RANGE'])) {
$V_Start = $Video_Start;
$V_End = $Video_End;
list(, $Video_Range) = explode('=', $_SERVER['HTTP_RANGE'], 2);
if (strpos($Video_Range, ',') !== false) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
if ($Video_Range == '-') {
$V_Start = $File_Size - substr($Video_Range, 1);
}else{
$Video_Range = explode('-', $Video_Range);
$V_Start = $Video_Range[0];
$V_End = (isset($Video_Range[1]) && is_numeric($Video_Range[1])) ? $Video_Range[1] : $File_Size;
}
$V_End = ($V_End > $Video_End) ? $Video_End : $V_End;
if ($V_Start > $V_End || $V_Start > $File_Size - 1 || $V_End >= $File_Size) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
$Video_Start = $V_Start;
$Video_End = $V_End;
$Video_Length = $Video_End - $Video_Start + 1;
fseek($File_Open, $Video_Start);
header('HTTP/1.1 206 Partial Video Content');
}
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
header("Content-Length: ".$Video_Length);
$buffer = 1024 * 8;
while(!feof($File_Open) && ($p = ftell($File_Open)) <= $Video_End) {
if ($p + $buffer > $Video_End) {
$buffer = $Video_End - $p + 1;
}
set_time_limit(0);
echo fread($File_Open, $buffer);
ob_flush();
}
fclose($File_Open);
exit();
?>
上記のコードは、HTML 5 ストリーミング機能のようなブラウザーでビデオを再生できるビデオ ストリーミング プレーヤーを実装します。 出力を参照してください。
このビデオ プレーヤーが PHP でどのように実装されているかを説明してみましょう。
-
まず、コードはビデオ ファイルを開こうとし、ビデオ サイズ、長さ、開始バイト、終了バイトなどのパラメータを設定します。
-
その後、コードはビデオの適切なヘッダーを設定しようとします。 ブラウザーを使用してブラウザーと通信する必要があるため、これは最も重要なステップです。
-
ヘッダーのステップでは、動画のデフォルト形式と、ブラウザーが受け入れる動画の範囲を決定します。
-
HTTP_RANGE
は、ブラウザーが範囲要求を行うときに使用されます。 範囲要求を検証し、それに応じてデータを送信します。 -
カスタマイズされたバッファは、サーバーのメモリ使用率を最適化するために使用されるため、ビデオのストリーミング中に大量のメモリが消費されることはありません。
-
最後に、ファイルを閉じて終了します。
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook