Reproductor de vídeo PHP
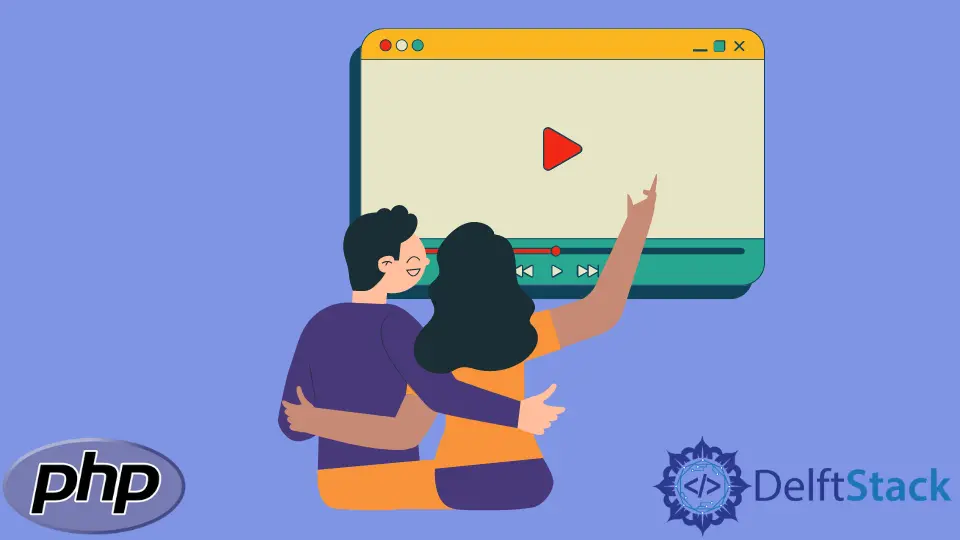
Este tutorial demuestra cómo crear un reproductor de video en PHP.
Reproductor de vídeo PHP
Podemos crear un reproductor de video en PHP utilizando la lógica de transmisión HTML. Podemos usar PHP para abrir y leer el archivo de video y enviarlo para su transmisión.
Pasemos a un ejemplo y luego describamos cómo funciona:
<?php
$Video_File = "C:\Users\Sheeraz\OneDrive\Desktop\New folder\sample.ogv";
if(!file_exists($Video_File)) return;
$File_Open = @fopen($Video_File, 'rb');
$File_Size = filesize($Video_File); // size of the video file
$Video_Length = $File_Size; // length of the video
$Video_Start = 0; // The start byte
$Video_End = $File_Size - 1; // The end byte
header('Content-type: video/mp4');
header("Accept-Ranges: 0-$Video_Length");
header("Accept-Ranges: bytes");
if (isset($_SERVER['HTTP_RANGE'])) {
$V_Start = $Video_Start;
$V_End = $Video_End;
list(, $Video_Range) = explode('=', $_SERVER['HTTP_RANGE'], 2);
if (strpos($Video_Range, ',') !== false) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
if ($Video_Range == '-') {
$V_Start = $File_Size - substr($Video_Range, 1);
}else{
$Video_Range = explode('-', $Video_Range);
$V_Start = $Video_Range[0];
$V_End = (isset($Video_Range[1]) && is_numeric($Video_Range[1])) ? $Video_Range[1] : $File_Size;
}
$V_End = ($V_End > $Video_End) ? $Video_End : $V_End;
if ($V_Start > $V_End || $V_Start > $File_Size - 1 || $V_End >= $File_Size) {
header('HTTP/1.1 416 Requested Video Range Not Satisfiable');
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
exit;
}
$Video_Start = $V_Start;
$Video_End = $V_End;
$Video_Length = $Video_End - $Video_Start + 1;
fseek($File_Open, $Video_Start);
header('HTTP/1.1 206 Partial Video Content');
}
header("Content-Range: bytes $Video_Start-$Video_End/$File_Size");
header("Content-Length: ".$Video_Length);
$buffer = 1024 * 8;
while(!feof($File_Open) && ($p = ftell($File_Open)) <= $Video_End) {
if ($p + $buffer > $Video_End) {
$buffer = $Video_End - $p + 1;
}
set_time_limit(0);
echo fread($File_Open, $buffer);
ob_flush();
}
fclose($File_Open);
exit();
?>
El código anterior implementa un reproductor de transmisión de video que puede reproducir el video en un navegador como la funcionalidad de transmisión de HTML 5. Ver la salida:
Intentemos describir cómo se implementa este reproductor de video en PHP:
-
Primero, el código intenta abrir el archivo de video y luego establece los parámetros para él, incluido el tamaño del video, la duración, el byte de inicio y el byte de finalización.
-
Después de eso, el código intenta establecer los encabezados adecuados para el video. Este es el paso más importante porque necesitamos comunicarnos con el navegador usando un navegador.
-
En el paso de encabezados, decidimos los formatos predeterminados del video y el rango de videos que aceptará el navegador.
-
El
HTTP_RANGE
se utiliza cuando el navegador realiza la solicitud de rango. Validará la solicitud de rango y enviará los datos en consecuencia. -
El búfer personalizado se utiliza para optimizar la utilización de la memoria del servidor, por lo que no se utiliza una gran cantidad de memoria mientras se transmite el video.
-
Finalmente, cierre el archivo y salga.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook