PHP Variable in String
- Use Template String to Interpolate Variables Directly Into the String in PHP
- Use String Template to Concatenate a PHP Variable With a String Literal by Assigning the Value of the String to a Variable
-
Use the Dot (
.
) Operator to Concatenate a PHP Variable With a String
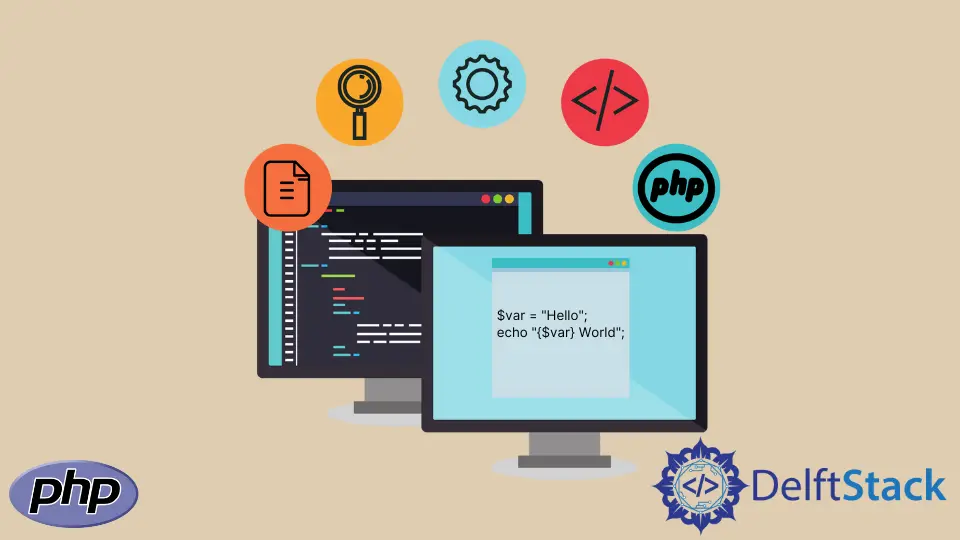
We will introduce a way to concatenate a PHP variable with a string with template strings.
We will introduce a method to interpolate a PHP variable with string literal by assigning the string literal to a variable. We use the template strings in this method too. In this method, we interpolate a prefix and suffix to form a word.
We will demonstrate a simple and most common way to concatenate a PHP variable with a string using the .
operator.
Use Template String to Interpolate Variables Directly Into the String in PHP
We can use a template string in PHP to concatenate a variable and a string. We use curly braces for the interpolation. The curly braces contain the variable, and we write the string that needs to be interpolated right after the curly braces. The variable inside the curly braces is the placeholder for the value. In this method, we interpolate a prefix and a suffix where prefix is a variable, and the suffix is a string literal. Please have a look at the Complex (curly) syntax in the PHP manual to learn about the string template.
For example, declare a variable $txt
and assign it with "salt"
. Use template string to interpolate the string y
to the string salt
. For this, write the variable $txt
inside the curly braces and write the string y
right after the curly braces without giving whitespace. Use double quotes for this template string and print it using the echo
command. Note that the use of single quotes in the template string will not print the interpolated string. Instead, it will print the text inside the single quotes. Always use double quotes while using template string.
Example Code:
# php 7.*
<?php
$txt = "salt";
echo "{$txt}y";
?>
Output:
salty
Use String Template to Concatenate a PHP Variable With a String Literal by Assigning the Value of the String to a Variable
We can use a string template not only to concatenate a PHP variable and a string but we can also use it to concatenate two PHP variables. We interpolate a suffix and a prefix in this method, where suffix and prefix both are variables. A string literal that is needed to be concatenated is stored in a variable. We make the use of curly braces to enclose the variable similar to the first method. For example, we declare two variables, $prefix
and $suffix
, and assign them with the values Comfort
and able
, respectively. We write each variable inside the curly braces individually without leaving whitespace between the two curly braces. Then we print it. The PHP compiler reads the value of the variables inside of the curly braces while compiling.
In the example below, the script concatenates the prefix Comfort
and the suffix able
and prints out as Comfortable
. Thus, we can use the complex/curly syntax to interpolate PHP variables with string literals and PHP variables.
Code Example:
#php 7.x
<?php
$prefix = "Comfort";
$suffix = "able";
echo "{$prefix}{$suffix}";
?>
Output:
Comfortable
Use the Dot (.
) Operator to Concatenate a PHP Variable With a String
We can use a simple method to concatenate a PHP variable with a string literal using the dot operator. We write a string literal and a .
after it. After the dot, we write the variable to be concatenated. In this method, we interpolate a prefix and a suffix where prefix is a string literal, and the suffix is a variable.
For example, declare a variable $taste
and assign it with the string value sweet
. Use the .
operator to interpolate the string ie
to the string sweet
. For this, write the variable $taste
, a dot operator after it, and the string ie
right after the dot operator. We display the message using the echo
command. We do not use any types of quotes in this method.
Example Code:
#php 7.x
<?php
$taste = "ie";
echo sweet.$taste;
?>
Output:
sweetie
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn